Question
c++ #ifndef LINKEDLIST_H #define LINKEDLIST_H #include using namespace std; template class LinkedList { private: // Declare a structure for the list struct ListNode { T
c++
#ifndef LINKEDLIST_H #define LINKEDLIST_H #include
template
ListNode *head; // List head pointer
public: LinkedList() // Constructor { head = nullptr; } ~LinkedList(); // Destructor void appendNode(T); void insertNode(T); void deleteNode(T); void displayList(); int search(T); // search function T getTotal(); int numNodes(); T getAverage(); T getLargest(); int getLargestPosition(); T getSmallest(); int getSmallestPosition(); };
//************************************************** // appendNode appends a node containing the value * // pased into newValue, to the end of the list. * //**************************************************
template
// Allocate a new node & store newValue newNode = new ListNode; newNode->value = newValue; newNode->next = nullptr;
// If there are no nodes in the list // make newNode the first node if (!head) head = newNode; else // Otherwise, insert newNode at end { // Initialize nodePtr to head of list nodePtr = head;
// Find the last node in the list while (nodePtr->next) nodePtr = nodePtr->next;
// Insert newNode as the last node nodePtr->next = newNode; } }
//************************************************** // displayList shows the value * // stored in each node of the linked list * // pointed to by head. * //**************************************************
template
nodePtr = head; while (nodePtr) { cout value next; } }
//************************************************** // The insertNode function inserts a node with * // newValue copied to its value member. * //**************************************************
template
// Allocate a new node & store newValue newNode = new ListNode; newNode->value = newValue; // If there are no nodes in the list // make newNode the first node if (!head) { head = newNode; newNode->next = nullptr; } else // Otherwise, insert newNode { // Initialize nodePtr to head of list and // previousNode to a null pointer. nodePtr = head; previousNode = nullptr;
// Skip all nodes whose value member is less // than newValue. while (nodePtr != nullptr && nodePtr->value next; }
// If the new node is to be the 1st in the list, // insert it before all other nodes. if (previousNode == nullptr) { head = newNode; newNode->next = nodePtr; } else // Otherwise, insert it after the prev. node. { previousNode->next = newNode; newNode->next = nodePtr; } } }
//***************************************************** // The deleteNode function searches for a node * // with searchValue as its value. The node, if found, * // is deleted from the list and from memory. * //*****************************************************
template
// If the list is empty, do nothing. if (!head) return; // Determine if the first node is the one. if (head->value == searchValue) { nodePtr = head->next; delete head; head = nodePtr; } else { // Initialize nodePtr to head of list nodePtr = head;
// Skip all nodes whose value member is // not equal to searchValue. while (nodePtr != nullptr && nodePtr->value != searchValue) { previousNode = nodePtr; nodePtr = nodePtr->next; }
// If nodePtr is not at the end of the list, // link the previous node to the node after // nodePtr, then delete nodePtr. if (nodePtr) { previousNode->next = nodePtr->next; delete nodePtr; } } }
//************************************************** // Destructor * // This function deletes every node in the list. * //**************************************************
template
nodePtr = head; while (nodePtr != nullptr) { nextNode = nodePtr->next; delete nodePtr; nodePtr = nextNode; } }
//***************************************************** // search member function * //This function performs a linear search of the list. * //*****************************************************
template
nodePtr = head; while (nodePtr) { if( nodePtr->value == val) { return count; } else { nodePtr = nodePtr->next; count++; } } return 0; }
//************************************************* // The getTotal function returns the total of * // all the nodes in the list. * //************************************************* template
//************************************************ // The numNodes function returns the number of * // nodes in the list. * //************************************************ template
//************************************************* // The getLargest function returns the largest * // value in the list. * //************************************************* template
//************************************************* // The getLargestPosition function returns the * // position of the largest value in the list. * //************************************************* template
return position; }
//************************************************* // The getSmallest function returns the smallest * // value in the list. * //************************************************* template
//************************************************* // The getSmallestPosition function returns the * // position of the smallest value in the list. * //************************************************* template
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
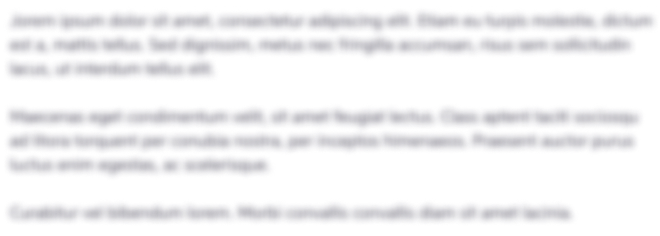
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started