Question
C++ - Implement functions in Roster.cpp: #include Roster.h #include #include #include Roster::Roster() { // initialize to empty array this->numStudents = 0; for (int i=0; i
C++ - Implement functions in Roster.cpp:
#include "Roster.h"
#include
#include
#include
Roster::Roster() {
// initialize to empty array
this->numStudents = 0;
for (int i=0; i
this->students[i] = NULL;
}
}
void Roster::resetRoster() {
// To avoid memory leaks:
// Recycle memory for all allocated students on roster
while (this->numStudents > 0) {
delete this->students[this->numStudents - 1];
this->numStudents --;
}
}
void Roster::addStudentsFromFile(std::string filename) {
std::ifstream ifs; // the stream we will use for the input file
ifs.open(filename);
if (ifs.fail()) {
std::cerr << "Could not open input file: "
<< filename << std::endl;
exit(2);
}
// ifs is an instance of ifstream
// ifstream inherits from istream,
// i.e. ifs is-a istream
this->addStudentsFromStream(ifs);
}
void Roster::addStudentsFromStream(std::istream &is) {
this->resetRoster();
std::string thisLine;
// Try to read past the header line.
getline(is,thisLine);
if ( is.eof() || is.fail() ) {
std::cerr << "Unable to read first line of input stream" << std::endl;
exit(3);
}
getline(is,thisLine);
while ( !is.eof() && !is.fail() ) {
// If we get here, it means the most recent attempt to read succeeded!
// So do something with thisLine
Student *sPtr = new Student(thisLine);
this->students[this->numStudents] = sPtr;
this->numStudents++;
// try to read another line
getline(is,thisLine);
} // end while
}
int Roster::getNumStudents() const {
return -999; // stub
}
Student Roster::getStudentAt(int index) const {
return Student(-999,"Stubbi","Stubsdottir");
}
std::string Roster::toString() const {
std::string result = "{ ";
result += "STUB!!!!"; // @@@ RESTORE THIS
result += "} ";
return result;
}
void Roster::sortByPerm() {
// SELECTION SORT
// stub does nothing
}
int Roster::indexOfMaxPermAmongFirstKStudents(int k) const {
return 0; // STUB
}
void Roster::sortByPermHelper(int k) {
// swaps max perm from [0..k-1] with elem [k-1]
int im = indexOfMaxPermAmongFirstKStudents(k);
// now swap the pointers between index im and index k-1
// THIS IS STILL A STUB !!!
}
----------------------------------------------------------------
Roster.h:
#ifndef ROSTER_H
#define ROSTER_H
#include
#include
#include "Student.h"
class Roster {
public:
Roster();
static const int ROSTER_MAX = 1024;
void addStudentsFromStream(std::istream &is);
void addStudentsFromFile(std::string filename);
int getNumStudents() const;
Student getStudentAt(int index) const;
std::string toString() const;
// Format of Roster::toString()
// opening "{ ",
// one Student toString() per line, each followed by ", "
// closing "} "
// No comma on last line before closing }
// Example:
// {
// [1234567,Smith,Mary Kay],
// [7654321,Perez,Carlos]
// }
void sortByPerm(); // use Selection Sort
void resetRoster(); // delete all students from roster
// By rights, these next two helper functions
// should probably be private. We expose them as
// public so they are easily unit testable.
void sortByPermHelper(int k); // swaps max perm from [0..k-1] with elem [k-1]
int indexOfMaxPermAmongFirstKStudents(int k) const;
private:
// pointers to Students on heap!
Student *students[ROSTER_MAX];
int numStudents;
};
#endif
------------------------------------------------
Student.h:
#ifndef STUDENT_H
#define STUDENT_H
#include
class Student {
public:
Student(int perm,
std::string lastName,
std::string firstAndMiddleNames);
//initialize one student from a comma separated string,
// e.g. 1234567,Smith,Mary Kay
Student(std::string csvString);
int getPerm() const;
std::string getLastName() const;
std::string getFirstAndMiddleNames() const;
std::string getFullName() const;
std::string toString() const; // e.g. [12345,Smith,Malory Logan]
private:
int perm;
std::string lastName;
std::string firstAndMiddleNames;
};
#endif
--------------------------------------
Student.cpp:
#include "Student.h"
#include
#include
Student::Student(int perm,
std::string lastName,
std::string firstAndMiddleNames) {
this->perm = perm;
this->lastName = lastName;
this->firstAndMiddleNames = firstAndMiddleNames;
}
// construct a Student object from a single line of
// comma separated text, e.g. "1234567,Smith,Mary Kay"
Student::Student (std::string csvString) {
std::istringstream iss(csvString);
// This version of getline takes an input string (istream), a string,
// and a delimiter character (in this case, comma).
// We read from the stream until the delimiter is encountered
std::string permAsString;
getline(iss, permAsString, ',');
this->perm = std::stoi(permAsString); // stoi converts std::string to int
getline(iss, this->lastName, ',');
getline(iss, this->firstAndMiddleNames, ' ');
}
int Student::getPerm() const {
return perm;
}
std::string Student::getLastName() const {
return lastName;
}
std::string Student::getFirstAndMiddleNames() const {
return firstAndMiddleNames;
}
std::string Student::getFullName() const {
return firstAndMiddleNames + " " + lastName;
}
std::string Student::toString() const {
// e.g. [12345,Smith,Malory Logan]
std::ostringstream oss;
oss << "["
<< this->getPerm() << ","
<< this->getLastName() << ","
<< this->getFirstAndMiddleNames() << "]";
return oss.str();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
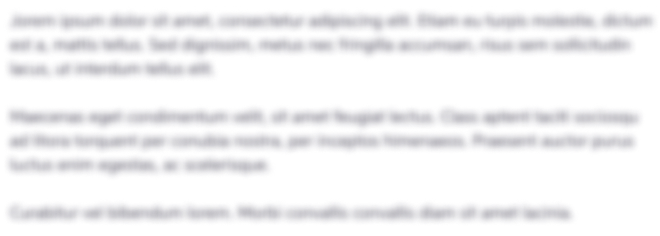
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started