Answered step by step
Verified Expert Solution
Question
1 Approved Answer
c++ In Part A, you will expand your search module to sort an array of strings and search the sorted array more efficiently. By the
c++
In Part A, you will expand your search module to sort an array of strings and search the sorted array more efficiently. By the end of Part A, your search module will have functions with the following prototype: Pertorm the following steps: 1. Add the new function prototypes into the header file. - Note: The functions with bold prototypes are new or changed in this part. The non-bold ones are unchanged. 2. Write an implementation for the mysort function. It should sort the elements of dat a from smallest to largest. You may use either selection sort or insertion sort. Include a comment as the first line of the function saying which sort you implemented. - FYI: We cannot name the function sort because there is already a function with that name in the standard library. Unfortunately, that function is awkward and using it requires concepts beyond the score of this course (e.g. function pointers). 3. Write an implementation for the issorted function. It should return the true if the array is in sorted order and false otherwise. An array with zero or one elements is always sorted. - Hint: If any element is smaller than the previous one, the array is not in sorted order. Otherwise, it is sorted. Remember not to check elements outside the beginning or end of the array. 4. Write an implementation for the sortedFindsmallest function. It should return the index of the smallest value in the sorted array passed as the parameter named data in. If there is more than one copy of that value in data in, you may return the index of any of them. Add an assert that ensures the array is sorted; your assert should call the issorted function. Then add another assert that ensures the array contains at least one element. - Reminder: In order to use asserts, you must in netude ccassert> at the top of the source (. cpp ) flle. If you include it in the header (.h) file, the program will compile but may report the errors as being in the header flle. - Reminder: An assert statement contains an expression that evaluates to a bool, just like an if statement does. The following assert: assert in > 0): means: If (n>0) else keepRunningProgram (1) everything is good stopprogramitithEr rorkessage ();U something is wrong - Reminder: asserts that are used for checking preconditions should be placed at the beginning of a function. - Reminder: asserts that are used for checking preconditions should be placed at the beginning of a function. - Reminder: Use a separate assert for each precondition. This will let you know which precondition failed, which will make debugging easier. - Hint: Finding the index of the smallest element in a sorted array is very simple. If you have more than one line of code after the asserts, you are doing more work than you need to. 5. Write an implementation for the sortedFindLargest function. It will be more complex than sortedFindSmallest, but not much more complex. Add asserts to ensure the array is sorted and contains at least one element. 6. Write an implementation for the binarysearch function. It should use a binary search to return the index of value value in in sorted array data in. If there is no such value in the array, the function should return the VALUE_NOT_FOUND HO_SUCH VAIUE constant. If there is more than one copy of value in in data_ in, you may return the index of any of them. Use an assert to ensure that the array is in sorted order. - Reminder: There are online notes for binary search at http:/lwww2,ss.uregina,ca/ anima/115/Notes/l6-Searching-and-Sorting/6a: searching,html\#binary-search. 7. Write an implementation for the binarysearchEirst function. It is similar to binarysearch except that it always returns the index of the first copy of value. Use an assert to ensure that the array is in sorted order. - Hint: Start by copying your binarysearch function. - Hint: The only thing you should have to change is the if check for whether the element at the current index has the value given in value_in. It should also require that (a) this is the first element in the array or (b) the previous element has a different value. Make sure you perform these checks in this order. If you put them backwards, you might try to examine the element at index 1, which would cause your program to crash. 8. Optional: Add an assert to the unsortedFindSma1lest function to ensure the array contains at least one element. Add a similar assert to the unsortedFindLargest function. 9. Test your Search module with the TestSearch 2. copp program provided. You will need the TestHelperih and TestHelper. apg files. Run the resulting program. It should give you full marks (example output). - Hint: g++Search.cpp TestSearch2.cpp TestHelper,cpp -o parta In Part B, you will update your main function to sort the player moves and check them using your new functions. Perform the following steps: 1. In main. cpp, after the end of the main loop, sort the array of player moves using your mysort function. 2. Change your program to use your new sortedFindsmallest, sortedFindLargest, and binarysearch functions in place of the corresponding Assignment 1 functions. Your program should act the same. - Hint: g++ PlaceString.cpp BoardSize.cpp Search.cpp main.cpp -o game In Part C, you will develop a simple module to store the possible values that can be on the game board. These values will be represented as chars. By the end of Part C, your Boardvalse module will have one function with the following prototype: bool isBoardvaluevalid (char value_in); Pertorm the following steps: 1. Add a header file for your Boardvalue module. Add whatever upragma, Hinclude, and using lines you need. Add the function prototype. 2. Add three symbolic constants of the char type to represent the possible states of a place on the board. BOARD VALUE EMPTY should have a value of " " BOARD VALUE BLACK should have a value of " O ", and BOARD_VALUE_WHITE should have a value of " e ". - Reminder: A char value (e.g.H) is written in single quotes. A string literal (e.g. "He110") is written in double quotes. You can have a string containing only one character (e.g. "H"), but it is not the same as a char value. 3. Add a source file to your project for your soardvalue module. Add whatever \#include and us ing lines you need. 4. Add an implementation for the isBoardValveVai id function. It should retum true if the parameter is equal to any of your three constants. Otherwise it should return false. 5. Test your Boardvalue module with the Ioatsoandralse2 _ con program provided. You will need the Iestilelpet,h and text llelest. foc files. The resulting program should give you full marks (example oulpuit. - Hint? q++ BoardValue.epp TentBoardValue2. epp Tenthelper.cpp -o parte Part D: The Board Module [45\% test program, includes 5\%s for Part F and 5\% for Part G] In Part D. you will develop a module to represent the game board. The module will contain a record (struct) named Board and functions to manipulate it. By the end of Part D, your Board module will have functions with the following prototypes: char boardGetht (const Boards board_in, int row_in, int colunn_in) t void boardsetAt (Boardt board_in, int rovin, int eoluen_in, ehar value_in) : void boardClear (Boarde board in ) z void boardload (Boardt board_in, const std: stringt filename_in); int boardCountitithValue (const Boards board_in, char value_in) ? void boardprint (eonst Board board_in) ; Perform the following steps: 1. Add a header file for the Board module. Add pragma line and an in include for Boardsize , h. - Hint: You will add the function prototypes in Step 3, after declaring the Board type. 2. Declare a record (struct) named Board to represent the game board. It should contain a 2-dimensional array of chars as its only member fieid. The array dimensions should be BOARD_SI2E rows and BOARD_SI SE columns. 3. Add the function prototypes. They must be after the declaration for Board because they use the Board type. 4. Add a source file for your Board module. Add lines to tinciude the and libraries as well as your Boardsize. h, Board. h, and BoardValue , hheaders. Also add the using statement. 5. In the Board. . cpp source file, add an implementation for the boardGetht function. It should start with an assert to ensure that the row and column specify a place on the board. Then the board'etAt function should access the array in the board in record to determine the board value at that place and return it. 5. In the Board. cpp source file, add an implementation for the boardGetAt function. It should start with an assezc to ensure that the row and column specily a place on the board. Then the boardGetAt function should access the array in the board in record to determine the board value at that place and return it. - Hint: Call your inonBoard function from the Joardsize module. - Hint about Syntax: If the array in your Board struct were named places and you wanted to access the element in row 3, column 4 to print it (you dont thoughl), you would use cout board_In.places [3][4] end1; 6. Add an implementation for the boardset.At function. It should start with two asserts. The first should ensure that the row and column specily a place on the board. The second should ensure that the new board value is valid. Then it should set the appropriate element of the array in board_in to the specified value. - Hint: Call the isBoazdval uevalid function. 7. Add an implementation for the boardclear function. It should set every element of the board_in array to BOARD__ALUE_EMPTY. - Hint: The boardiclear function does not need any asserts. 8. Add an implementation for the boardCountwithValue function. It should return the number of elements in the array in board_in that have value value_in. Add an assert to ensure that the value you are counting is a valid value. 9. Add an implementation for the boardprint function. For now, it should print every element of the array. After each element, print a space (" "or " "). After each row, print a newline (end1). - Note: You will improve the boardprint tunction in Parts E and F. Testing [Optional section, added January 28, 2023]: Make your own test program named mainboard . cpp to test your Board functions before trying the official test program. The otticial one is intended to evaluate your software and it may not help you determine the causes of your errors. Copy your main. cpp fle as mainBoard. cpp, In mainBoard. cpp, delete all the lines inside the main function, except the return 0 at the end. Add tinclude statements for Boardvalue, th and Board. h. At the beginning of the main function, declare a variable of type Board. Call your boardClear function on your variable. Pass it the variable from the previous step as a parameter (in parentheses). Call your boardprint function on your variable. See the list of prototypes at the beginning of this section to determine the number and types of its parameters. Compile and run the result. After each the next steps, compile and run again. Hint: g+4 mainBoard. cpp Board. cpp. Boardsize.cpp Boardvalue.cpp o testd Call your boardseckt. function to set row 3, column 7 to BOAD_ mutrE_ Mat1E. then call boardprint again. See the list of prototypes at the beginning of this section to determine the number and types of the parameters for bosrdsetht. When checking the result, remember rows and columns are numbered from 0 . Add at least three more calls to boardsetht to set various legal positions in the array to BOARD_VALUE_WHITE, then call boardPrint again. Repeat the step above for BOARD_VALUE_BLACK. Call your boardGetat function for some position on the board with a white stone. Remember that when you call a function that returns a value, you should store or print the result. For example: char val = boardGet.At \& fin in the arguments for the function call i: Print a message showing the value that is returned. Call your boardGe cat function for several other locations on the board that are empty or have black or white stones. Print messages describing the results. Call your boardCountwi hValue function with BOARD_ VaLUE_ warte. Piemember to store the int value that it retumns. Print a message showing the count of white stones. Repeat the above step with black stones and empty board values. 10. Copy in the implementation for the ame board from a file. Change the line 0. Copy in the implementation for the beardisad function from the course website. It will load the state of a game board from a file.-Change the line by replacing places with the name of your own member afray. - Note: The boardLoad function uses stream-based file input (ifstream), which is taught in CS 110 but is not used elsewhere in CS 115 (this course). 11. Test your Board module with the TestBoard2 , crp program provided. It should give you a mark of 35/45 (example output). Make sure that you have downloaded empty.txt, ear.txt, and shapes. txt from the course website. These files need to be present in the same folder as your program when you execute your program. Hint: If your program does not score well on the loaded files, follow the optional testing instructions between steps 9 and 10 to find problems in your code. Hint:g++ Board.cpp BoardSize.cpp BoardValue.cpp Testhelper.cpp TestBoard2.cpp partd In Part E, you will add a game board to your game. You will also determine the score for each player and print the winner. Only manipulate the Board by calling the functions in the Board module. In the main function, do not use dot notation to access the member array. Perform the following steps: 1. Near the start of your ma in function, create a variable of the Board type. Immediately after, call a function from the Board module to clear the board. 2. At the start of your main loop, print the board by calling a function. 3. Previously, the game allowed black to play using any well-formed place-string that represented a position on the game board. Add another check to determine if that board place is empty by calling a function. If not, print "Forbidden: Place row RRR, column CCC is not empty". 4. If the place was empty, also set the board there to BOARD_VALUE_BLACK by calling a function. 5. After the end of the main loop, also print out the game board followed by a blank line. 6. Calculate the score for the black player by calling a function. For now, the score is the number of black stones on the board. 7. Calculate the score for the white player in a similar manner. White also gets 7.5 additional points as compensation for having to play second. - FYI: These extra points are called komi. There is ongoing debate about the ideal value, but 7.5 works well in most cases. Surprisingly, the same komi works well for most board sizes. 8. Print a message saying which player won (black or white) and how many points each player got. 9. Make sure that main. cpp never accesses the data inside the Board record (struct) using dot notation. If it ever does, change your program to call a function from the Board module instead. If the main. cpp file you hand in accesses the Board data with dot notation, you will lose marks. - Hint: Use the Find... command in replit. If your Board variable is named my_board, you could search for my_board. (with the dot). 10. Test your game. - Hint: g++ PlaceString.cpp BoardSize.cpp BoardValue.cpp Board.cpp Search.cpp main.cpp - - game In Part F, you will add nine asterisks/stars ( s) to the board display to mark the traditional star points on empty cells. The star points are arranged in a 33 grid with one at the board center. The spacing between them is 6 places, and thus the outer points are 3 places from the board edge. You will work out which rows and columns have star points independently. The star points are wherever both the row and column are suitable. The board is symmetrical and therefore you will be able to use the same function to check both the rows and the columns. By the end of Part F, your Board module will have functions with the following prototypes: Perform the following steps: 1. Add an int constant to the Board header file named STAR_POINT_SPACING and with a value of 6 . 2. Next, add the boardisAlignedForstarpoint function. It has a precondition that the index_in parameter is at least 0 . It should return true if the specified row (or column) has star points and false otherwise. The row has star points if the index modulus STAR_POINT_SPACING equals half the board size modulus STAR_POINT_SPACING. 3. Update your boardPrint function to print the star points. When printing each place on the board, check whether (a) it is empty, (b) there are star points on that row, and (c) there are star points on that column. If all three are true, print a. Otherwise, print the array value for that place as before. - Note: If there is a stone on a star point, that star point is not displayed. 4. Test your Board module with the Testboard2, ane program again. The 19 by 19 grid should now have the 9 star points marked on it. The test program should now give you a mark of 40/45 (example eutput). - Reminder: g++ Board.cpp BoardSize.cpp BoardValue.cpp TestHelper.cpp TestBoard2.cpp 0 partd The row numbers will be displayed on the left and right and the column letters will be displayed on the top and bottom. By the end of Part G, your Board module will have functions with the following prototypes: char boardGetAt (const Boards board_in, int row_in, int column_in); void boardSetAt (Board board_in, int row_in, int column_in, char value_in); void boardclear (Boards board_in); void boardioad (Boards board_in, const std: strings filename_in); int boardCountwithValue (const Board; board_in, char value_in); void boardprint (const Boards board_in); boardisAlignedForStarpoint (int index_in); void boardprintrownumber (int row_in); void boardprintColumnLetters (const Boards board_in); Perform the following steps: 1. Add the boardPrintRownumber function. It should print the row number using exactly two characters. If the number only contains one digit, print a space as the first character. - Note: This will make the numbers appear to be right-aligned. - Note: You can do this using the formatting functions in the library. Of you can do it using if-else statements. Both approaches work. 2. Update your boardprint function to print the row numbers on the left and right side of the game board. On the left side, print a space between the number and the board. On the right side, you are already printing a space. 3. Add the boardPrintcolumnietters function. It should print a row of letters naming the columns. The column letters should appear directly above the corresponding columns. You will have to print a space between each letter. - Remember: There are no columns named ' I' and ' 0 '. The letters go directly from ' H' to ' L' and from ' N ' to 'P'. - Hint: Remember to leave space for the row numbers before the first column. 4. Update your boardprint function to print the column letters on the top and bottom of the game board. 5. Test your Board module with the TestBoard2, cop program again. There should now be numbers and letters around the board. The test program should now give you full marks (example output). - Reminder:g++ Board. cpp Boardsize.cpp Boardvalue.,cpp Testhelper,cpp TestBoard2,cpp -o partd 6. Test your game again before handing it in. Make sure it still works after the changes you made in Parts F and G. - Reminder:g++ placestring.epp BoardSize.cpp Boardvalue.cpp Board.cpp Search.cpp main.cpp -o game 








Step by Step Solution
There are 3 Steps involved in it
Step: 1
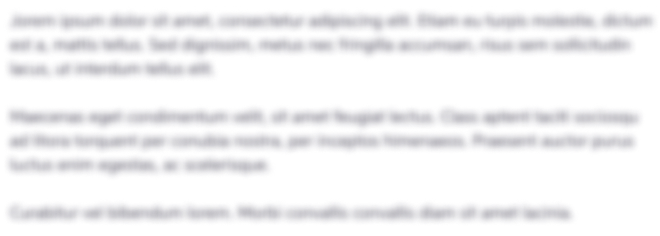
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started