Question
c program Goal: Multithreaded sieve of eratosthenes This program seems to crash when entering ./a.out -t 2 -u 10000 or even just using the default
c program
Goal: Multithreaded sieve of eratosthenes
This program seems to crash when entering ./a.out -t 2 -u 10000 or even just using the default values ./a.out
typedef struct BitBlock {
uint32_t bits;
pthread_mutex_t mutex;
} BitBlock_t;
static uint32_t val = 10240;
static BitBlock_t b[4];
static int num_threads = 1;
static unsigned short isVerbose;
void setbit(BitBlock_t a[], uint32_t k) {
pthread_mutex_lock(&a[k / 32].mutex);
a[k / 32].bits |= 1 << (k % 32);
pthread_mutex_unlock(&a[k / 32].mutex);
}
void clearbit(BitBlock_t a[], uint32_t k) {
a[k / 32].bits &= ~(1 << (k % 32));
}
int testbit(BitBlock_t a[], uint32_t k) {
int test = -1;
pthread_mutex_lock(&a[k / 32].mutex);
test = ((a[k / 32].bits & (1 << (k % 32))) != 0);
pthread_mutex_unlock(&a[k / 32].mutex);
return test;
}
void* sieveoferatosthenes(void* vid) {
long tid = (long)vid;
uint32_t start = tid * (val / num_threads) + 2;
uint32_t end = (tid == num_threads - 1) ? val : start + (val / num_threads) - 1;
for (uint32_t j = start; j <= end; j++) {
for (uint32_t p = 2; p * p <= j; p++) {
if (testbit(b, p) != 1) {
for (uint32_t i = p * p; i <= j; i += p) {
setbit(b, i);
}
}
}
}
pthread_exit(EXIT_SUCCESS);
}
int main(int argc, char* argv[]) {
int opt = -1;
pthread_t* threads = NULL;
long tid = 0;
for (int i = 0; i < 4; i++) {
pthread_mutex_init(&b[i].mutex, NULL);
b[i].bits = 0;
}
while ((opt = getopt(argc, argv, "t:u:hv")) != -1) {
switch (opt) {
case 't':
num_threads = atoi(optarg);
break;
case 'u':
val = atoi(optarg);
break;
case 'h':
printf("%s: -t # -u # -h -v ", argv[0]);
printf("\t-t # for number of threads ");
printf("\t-u # for the upper bound of largest number "); exit(0); break; case 'v': isVerbose = 1; break; default: /* '?' */ exit(EXIT_FAILURE); break; }
}
threads = malloc(num_threads * sizeof(pthread_t)); if(isVerbose) { fprintf(stderr, "number of threads: %d", num_threads); fprintf(stderr, "upper bound: %d", val); }
for (tid = 0; tid < num_threads; tid++) { pthread_create(&threads[tid], NULL, sieveoferatosthenes, (void *) tid); }
for (tid = 0; tid < num_threads; tid++) { pthread_join(threads[tid], NULL); }
for (uint32_t p = 2; p <= val; p++) { if (testbit(b, p) != 1) printf("%u ", p); }
free(threads); for(int i = 0; i < 4; i++) { pthread_mutex_destroy(&b[i].mutex); }
return EXIT_SUCCESS; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
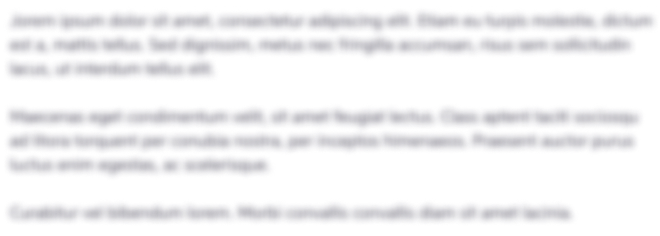
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started