Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C program :Your program will print all Cube Sums with integers 0 . . N in order from smallest to largest. ( Notice there was
C program :Your program will print allCube Sumswith integers N in order from smallest to largest. Notice there was a tie above, figure out how it was broken. You cannot generate all values and sort them. This would require making N values, which would use excessive space. Instead you will use a Heap to generate them in order.
The basic algorithm is described below.
Create a new Heap
For integers i up to N do
Add i i to the Heap
End For
While Heap is not Empty do
Get the Minimum Sum
Print the Sum
If the sum's j value is less than N:
Put a new sum in the heap ij i j
End If
End Do
Delete the Heap
You may make a one assumptions.
The user will always give you a positive integer as input
You must implement the following in your solution.
You must use the following Structure to store theCube Sums.
Stores a sum i j k
typedef struct CubeSum CubeSum;
struct CubeSum
int sum; result of i j
int i; First integer in sum
int j; Second integer in sum
;
You must implement a Heap withat leastthe following methods. You may create additional methods, but youmustuse these.
Create a new heap with starting size
@return the heap that was created.
Heap newHeap;
Delete the heap. Free all memory used.
@param myHeap is the heap to delete
void deleteHeapHeap myHeap;
Determine if the heap is empty.
@param myHeap is the heap to check
@return true if the heap is empty and false otherwise
bool isEmptyHeap myHeap;
Insert a new sum into the heap.
@param myHeap is the heap to insert into
@param sum is a pointer to the sum to insert
void insertHeap myHeap CubeSum sum;
Remove the minimum from the heap.
@param myHeap is the heap to remove from
void removeMinHeap myHeap;
Get the pointer to the smallest CubeSum.
@param myHeap is the heap to get the min of
@return a pointer to the smallest CubeSum
CubeSum getMinHeap myHeap;
Example
Cube Sum Program.
Computes Cube Sums from N
Enter max value of N:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
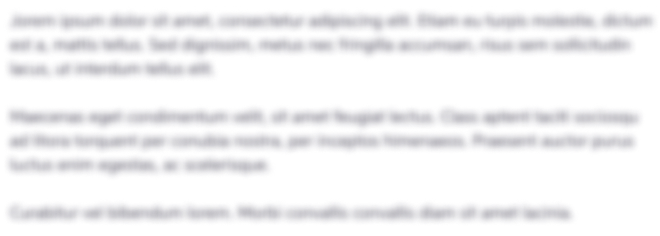
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started