Question
C programming. I need help changing the function vehicle* allocVehicle ( int t, int num, int row, int col ) in the program below to
C programming.
I need help changing the function vehicle* allocVehicle ( int t, int num, int row, int col ) in the program below to this description:
vehicle* allocVehicle(char* model, char* plate, VType type, double curFuel, double maxFuel ) - dynamically allocates space to store one vehicle record with the given model, plate, type, curFuel and maxFuel then initialize all its values sensibly (note: this function does not assign the vehicle a parking stall)
For three types of vehicles (cars, trucks and SUVs), use the following enum construct to enforce that a vehicle must be one of three specific types:
enum VType { CAR, TRUCK, SUV };
/* Vehicle parking program */
//assignment2//
#include
#include
#include
#define CAR 1
#define TRUCK 2
#define SUV 3
#define CARFUEL 55
#define TRUCKFUEL 100
#define SUVFUEL 70
/* to store vehicle number, and its
row-col position in an array */
struct vehicle
{
int num ;
int row ;
int col ;
int type ;
} ;
int parkinfo[6][10] ; //number of vehicle parked
int vehcount ; // total vehicle parked
int carcount ; // total cars
int truckcount ; /* total trucks */
int suvcount ; /* total suv*/
float carfuel[20];
float truckfuel[20];
float suvfuel[20];
int vehicle[20];
float vehiclefuel[20] = {10,53,40,34,30,11,12,41,23,32,39,37,32,54,21,2,19,29,49,5};
float car[20];
float truck[20];
float suv[20];
float curfuel[20] = {10,53,40,34,30,11,12,41,23,32,39,37,32,54,21,2,19,29,49,5};
int numberplate = 0;
float fuel = 0; // fuel in percentage
bool g = false;
void display( ) ;
void changecol ( struct vehicle * ) ; // no need
struct vehicle * allocVehicle( int, int, int, int ) ;
void deallocVehicle ( struct vehicle * ) ;
void getfreerowcol ( int, int * ) ;
void getrcbyinfo ( int, int, int * ) ;
double calcFuel(struct vehicle* v);
int refuelVehicle(int, int);
void displayfuel(int,int);
void printRefuelReport();
void show( ) ;
void displayFuels();
/* decrements the col. number by one
this fun. is called when the data is
shifted one place to left */
void changecol ( struct vehicle *v )
{
v -> col = v -> col - 1 ;
}
void printRefuelReport()
{
int j= 0;
for( j = 0; j < 20; j++)
{
if (vehiclefuel[j] < 50)
{
if(vehicle[j] != 0)
{
g = true;
printf("Total fuel in percent is = %f",vehiclefuel[j]);
printf(" for Vehicle Number = %d ", vehicle[j] );
printf(" ");
}
else
{
if ( g == true)
{
break;
}
else
{
printf("The number of vehicles with less than 50% fuel tank is: ", 0.0);
}
}
}
}
}
void displayfuel(int numPlate, int t)
{
int j = 0;
for( j = 0; j < 20; j++)
{
if(vehicle[j] == numPlate)
{
if (t == 1)
{
printf("Vehicle Type: Car");
printf(" Total fuel in percent is = %f: ",vehiclefuel[j]);
printf(" for Vehicle %d =", vehicle[j] );
}
if (t == 2)
{
printf("Vehicle Type: Truck");
printf(" Total fuel in percent is = %f: ",vehiclefuel[j]);
printf(" for Vehicle %d =", vehicle[j] );
}
if (t == 3)
{
printf("Vehicle Type: SUV");
printf(" Total fuel in percent is = %f: ",vehiclefuel[j]);
printf(" for Vehicle %d =", vehicle[j] );
}
else
{
printf("Vehicle Does not exist");
break;
}
}
}
}
int refuelVehicle(int t, int n)
{
int i = 0 ;
for( i = 0; i < 20; i++)
{
if(vehicle[i] == n)
{
if(t == 1)
{
vehiclefuel[i] = 100;
}
if(t == 2)
{
vehiclefuel[i] = 100;
}
if(t == 3)
{
vehiclefuel[i] = 100;
}
}
}
displayfuel(t,n);
return 0;
}
double calcFuel(struct vehicle* v)
{
if(v -> type == CAR)
{
fuel = (curfuel[carcount-1]/CARFUEL) * 100;
vehicle[carcount-1] = numberplate;
vehiclefuel[carcount-1] = fuel;
}
else if (v -> type == TRUCK)
{
fuel = (curfuel[truckcount-1]/TRUCKFUEL) * 100;
vehicle[carcount-1] = numberplate;
vehiclefuel[carcount-1] = fuel;
}
else
{
fuel = (curfuel[suvcount-1]/SUVFUEL) * 100;
vehicle[carcount-1] = numberplate;
vehiclefuel[carcount-1] = fuel;
}
return 0;
}
/* adds a data of vehicle */
struct vehicle* allocVehicle ( int t, int num, int row, int col )
{
struct vehicle *v ;
v = ( struct vehicle * ) malloc ( sizeof ( struct vehicle ) ) ;
v -> type = t ;
v -> row = row ;
v -> col = col ;
if ( t == CAR )
{
carcount++ ;
}
else if(t == TRUCK)
{
truckcount++ ;
}
else
{
suvcount++ ;
}
vehcount++ ;
numberplate = num;
parkinfo[row][col] = num ;
calcFuel(v);
return v ;
}
/* deletes the data of the specified
car from the array, if found */
void deallocVehicle( struct vehicle *v )
{
int c ;
for ( c = v -> col ; c < 9 ; c++ )
parkinfo[v -> row][c] = parkinfo[v -> row][c+1] ;
parkinfo[v -> row][c] = 0 ;
if ( v -> type == CAR )
carcount-- ;
else if ( v -> type == TRUCK)
truckcount-- ;
else
suvcount-- ;
vehcount-- ;
}
/* get the row-col position for the vehicle to be parked */
void getfreerowcol ( int type, int *arr )
{
int r, c, fromrow = 0, torow = 2 ;
if ( type == TRUCK )
{
fromrow += 2 ;
torow += 2 ;
}
if(type == SUV)
{
fromrow += 4 ;
torow += 4 ;
}
for ( r = fromrow ; r < torow ; r++ )
{
for ( c = 0 ; c < 10 ; c++ )
{
if ( parkinfo[r][c] == 0 )
{
arr[0] = r ;
arr[1] = c ;
return ;
}
}
}
if ( r == 2 || r == 4 )
{
arr[0] = -1 ;
arr[1] = -1 ;
}
}
/* get the row-col position for the vehicle with specified number */
void getrcbyinfo ( int type, int num, int *arr )
{
int r, c, fromrow = 0, torow = 2 ;
if ( type == TRUCK )
{
fromrow += 2 ;
torow += 2 ;
}
if(type == SUV)
{
fromrow += 4 ;
torow += 4 ;
}
for ( r = fromrow ; r < torow ; r++ )
{
for ( c = 0 ; c < 10 ; c++ )
{
if ( parkinfo[r][c] == num )
{
arr[0] = r ;
arr[1] = c ;
return ;
}
}
}
if ( r == 2 || r == 4 )
{
arr[0] = -1 ;
arr[1] = -1 ;
}
}
/* displays list of vehicles parked */
void display( )
{
int r, c ;
printf ( "\xdb\xdb Cars => " ) ;
for ( r = 0 ; r < 5 ; r++ )
{
if ( r == 2 )
printf ( "\xdb\xdbTrucks => " ) ;
if ( r == 4)
printf( "\xdb\xdbSUVs => ");
for ( c = 0 ; c < 10 ; c++ )
printf ( "%d\t", parkinfo[r][c] ) ;
printf ( " " ) ;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
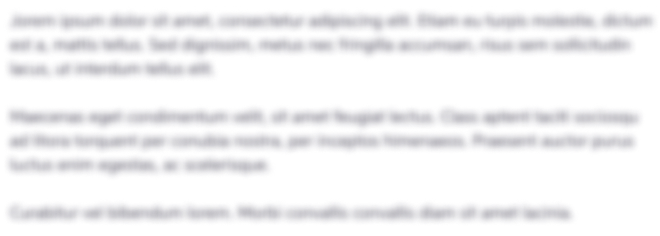
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started