Question
C programming. Modify your program to write the data from the linked list into a file before exiting the program, and then the next time
C programming.
Modify your program to write the data from the linked list into a file before exiting the program, and then the next time the program runs load the linked list data from the file before interacting with the user.
Can you modify this program by using fopen structure to satisfy the following order? I want it to be saved as a text file in shared folder when I type and run on program.
Here is the program :
#include
#include
#include
struct info
{
char title[20];
char author[20];
int year;
int no_pages;
struct info *next;
};
typedef struct info Info;
//declare functions to add t linked list, delete and print an item and print all items
void addItem(Info *list, Info item);
int findItem(Info *list, char *title);
void deleteItem(Info *list, char *title);
void printItem(Info *list,char *s);
void printAllItem(Info *list);
void sort(Info *list);
void add(Info **list, Info item);
int main()
{
Info *list = NULL;
Info item;
char choice = 'A',ch='Y';
char title[20];
do
{
printf("Enter action - (A)dd, (D)elete, (F)ind, (P)rint all ,(S)ort, (Q)uit: ");
//fflush(stdout);
scanf("%c", &choice);
fflush(stdin);
switch (choice)
{
case 'A':
printf("Enter the title to add : ");
//fflush(stdin);
//fflush(stdout);
gets(item.title);
printf("Enter the author : ");
gets(item.author);
printf("Enter the year was it published: ");
scanf("%d", &item.year);
fflush(stdin);
printf("Enter the number of pages : ");
scanf("%d", &item.no_pages);
fflush(stdin);
if (list == NULL)
{
list = (Info*)malloc(sizeof(Info));
strcpy(list->author, item.author);
strcpy(list->title, item.title);
list->year = item.year;
list->no_pages = item.no_pages;
list->next = NULL;
}
else
addItem(list, item);
break;
case 'D':
printf("Enter the title to delete an item : ");
//fflush(stdin);
gets(title);
deleteItem(list, title);
break;
case 'F':
printf("Enter the title to find : ");
fflush(stdin);
gets(title);
if (findItem(list, title))
{
printf("Item found ");
}
else
{
printf("I can't find that book. ");
}
break;
case 'P':
printAllItem(list);
break;
case 'S':
sort(list);
break;
case 'Q':
printf("Quit Application.....");
break;
default:
printf("Invalid option ");
break;
}
} while (choice != 'Q');
}
void add(Info **list, Info item)
{
*list = (Info*)malloc(sizeof(Info));
strcpy((*list)->title, item.title);
strcpy((*list)->author, item.author);
(*list)->year = item.year;
(*list)->no_pages = item.no_pages;
return;
}
void addItem(Info *list, Info item)
{
Info *newitem, *cur = list;
newitem = (Info*)malloc(sizeof(Info));
strcpy(newitem->title, item.title);
newitem->year = item.year;
strcpy(newitem->author, item.author);
newitem->no_pages = item.no_pages;
newitem->next = NULL;
if (list == NULL)
{
add(&list,item);
}
else
{
while (cur->next != NULL)
{
cur = cur->next;
}
cur->next = newitem;
}
}
int findItem(Info *list, char *title)
{
if (list == NULL)
{
printf("Cannt delete an item,list is empty ");
return 0;
}
Info *cur = list;
while (cur != NULL)
{
if (strcmp(cur->title, title) == 0)
{
return 1;
}
cur = cur->next;
}
return 0;
}
void deleteItem(Info *list, char *title)
{
Info *cur = list, *prev , *next = NULL;
if (list == NULL)
{
printf("Cannt delete an item,list is empty ");
return;
}
if (strcpy(list->title, title) == 0)
{
//found at first position delete and assign list next item
list = list->next;
free(cur);
return;
}
while (cur != NULL)
{
prev = cur;
if (cur->next != NULL)
next = cur->next;
if (strcpy(cur->title, title) == 0)
{
prev->next = next;
free(cur);
return;
}
cur = cur->next;
}
}
void printItem(Info *list,char *s)
{
Info *cur = list;
while (cur != NULL)
{
if (strcmp(cur->title, s) == 0)
{
printf("%s(%d) ", cur->title,cur->year);
printf(" %s ", cur->author);
//printf("Year: %d ", cur->year);
printf(" %d Pages ", cur->no_pages);
cur = cur->next;
}
}
}
void printAllItem(Info *list)
{
Info *cur = list;
while (cur != NULL)
{
printf("%s(%d) ", cur->title,cur->year);
printf(" %s ", cur->author);
printf(" %d Pages ", cur->no_pages);
cur = cur->next;
}
}
void sort(Info *list)
{
Info *cur = list,*next;
Info tmp;
while (cur != NULL)
{
if (cur->next != NULL)
{
next = cur->next;
if (strcmp(cur->title, next->title) > 0)
{
strcpy(tmp.title, cur->title);
strcpy(tmp.author, cur->author);
tmp.year = cur->year;
tmp.no_pages = cur->no_pages;
strcpy(cur->title, next->title);
strcpy(cur->author, next->author);
cur->year = next->year;
cur->no_pages = next->no_pages;
strcpy(next->title, tmp.title);
strcpy(next->author, tmp.author);
next->year = tmp.year;
next->no_pages = tmp.no_pages;
}
}
cur = cur->next;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
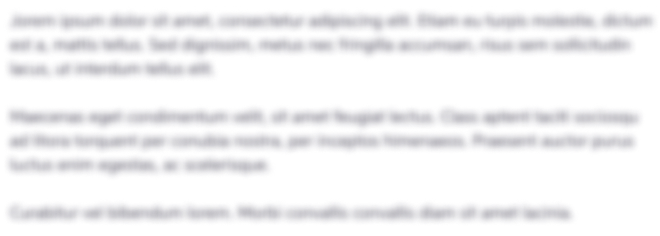
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started