Question
C programming ONLY. Do not do in C++ plaease Problem (complete steps A, B, and C) Take screenshot of compiled output (which should look like
C programming ONLY. Do not do in C++ plaease
Problem (complete steps A, B, and C)
Take screenshot of compiled output (which should look like the sample output provided)
Post all source code with your answer.
Add the member function InputDATE2() shown below and your versions of OutputDATE2() and IsLeapYearDATE() to the DATE source files Date.h and Date.c then use Problem2.c (see below) as a driver program to test your extensions.
(A) You will need to add the following prototypes to Date.h
int InputDATE2(DATE *date,FILE *IN);
void OutputDATE2(const DATE *date,char separator,FILE *OUT);
bool IsLeapYearDATE(const DATE *date);
(B) add the new function definitions to Date.c; and
(C) build a project with Date.h, Date.c, and Problem2.c and execute Problem2.exe. (all files located below; they are labeled)
//--------------------------------------------------------------
int InputDATE2(DATE *date,FILE *IN)
//--------------------------------------------------------------
{
const char DELIMITERS[] = " -/.\t ";
char line[80+2];
if ( fgets(line,80,IN) == NULL )
return( EOF );
else
{
char *pMM = strtok(line,DELIMITERS);
char *pDD = strtok(NULL,DELIMITERS);
char *pYYYY = strtok(NULL,DELIMITERS);
char *pepoch = strtok(NULL,DELIMITERS);
char epoch;
if ( pMM != NULL ) date->MM = atoi(pMM);
if ( pDD != NULL ) date->DD = atoi(pDD);
if ( pYYYY != NULL )
{
date->YYYY = atoi(pYYYY);
if ( pepoch != NULL )
epoch = pepoch[0];
else
epoch = pYYYY[strlen(pYYYY)-1];
if ( toupper(epoch) == 'A' )
date->epoch = 'A';
else if ( toupper(epoch) == 'B' )
date->epoch = 'B';
else
; // DO NOTHING!
}
return( 1 );
}
}
//-------------------------------------------------
// Date.h
//-------------------------------------------------
#ifndef DATE_H
#define DATE_H
//==============================================================
// Data model definitions
//==============================================================
typedef struct DATE
{
int MM;
int DD;
int YYYY;
char epoch;
} DATE;
//==============================================================
// Public member function prototypes
//==============================================================
void ConstructDATE(DATE *date);
void DestructDATE(DATE *date);
void InputDATE(DATE *date,FILE *IN);
void OutputDATE(const DATE *date,FILE *OUT);
void SetMMDATE(DATE *date,const int MM);
void SetDDDATE(DATE *date,const int DD);
void SetYYYYDATE(DATE *date,const int YYYY);
void SetEpochDATE(DATE *date,const char epoch);
int GetMMDATE(const DATE *date);
int GetDDDATE(const DATE *date);
int GetYYYYDATE(const DATE *date);
char GetEpochDATE(const DATE *date);
void ConvertToStringDATE(const DATE *date,char string[]);
//===============================================
// Private utility member function prototypes
//===============================================
(none)
#endif
//-------------------------------------------------
// Date.c
//-------------------------------------------------
#include
#include
#include
#include ".\Date.h"
//--------------------------------------------------------------
void ConstructDATE(DATE *date)
//--------------------------------------------------------------
{
(*date).MM = 2; date->DD = 3;
date->YYYY = 1954; date->epoch = 'A';
// Instrumentation
printf("Construction of DATE = "); OutputDATE(date,stdout); printf(" ");
}
//--------------------------------------------------------------
void DestructDATE(DATE *date)
//--------------------------------------------------------------
{
// Instrumentation
printf("Destruction of DATE = "); OutputDATE(date,stdout); printf(" ");
}
//--------------------------------------------------------------
void InputDATE(DATE *date,FILE *IN)
//--------------------------------------------------------------
{
fscanf(IN,"%d-%d-%d%c",&date->MM,&date->DD,&date->YYYY,&date->epoch);
}
//--------------------------------------------------------------
void OutputDATE(const DATE *date,FILE *OUT)
//--------------------------------------------------------------
{
// Use the format MM-DD-YYYYEE where EE = "AD" or "BC"
fprintf(OUT,"%2d-%2d-%4d%2s",date->MM,date->DD,date->YYYY,
(date->epoch == 'B') ? "BC" : "AD");
}
//--------------------------------------------------------------
void SetMMDATE(DATE *date,const int MM)
//--------------------------------------------------------------
{
date->MM = MM;
}
//--------------------------------------------------------------
void SetDDDATE(DATE *date,const int DD)
//--------------------------------------------------------------
{
date->DD = DD;
}
//--------------------------------------------------------------
void SetYYYYDATE(DATE *date,int YYYY)
//--------------------------------------------------------------
{
date->YYYY = YYYY;
}
//--------------------------------------------------------------
void SetEpochDATE(DATE *date,const char epoch)
//--------------------------------------------------------------
{
date->epoch = epoch;
}
//--------------------------------------------------------------
int GetMMDATE(const DATE *date)
//--------------------------------------------------------------
{
return( date->MM );
}
//--------------------------------------------------------------
int GetDDDATE(const DATE *date)
//--------------------------------------------------------------
{
return( date->DD );
}
//--------------------------------------------------------------
int GetYYYYDATE(const DATE *date)
//--------------------------------------------------------------
{
return( date->YYYY );
}
//--------------------------------------------------------------
char GetEpochDATE(const DATE *date)
//--------------------------------------------------------------
{
return( date->epoch );
}
//--------------------------------------------------------------
void ConvertToStringDATE(const DATE *date,char string[])
//--------------------------------------------------------------
{
sprintf(string,"%2d-%2d-%4d%2s",date->MM,date->DD,date->YYYY,
(date->epoch == 'B') ? "BC" : "AD");
}
//-------------------------------------------------
// Problem2.c
//-------------------------------------------------
#include
#include
#include
#include ".\Date.h"
//-------------------------------------------------
int main()
//-------------------------------------------------
{
DATE date;
ConstructDATE(&date);
printf("date? ");
while ( InputDATE2(&date,stdin) != EOF )
{
if ( IsLeapYearDATE(&date) )
OutputDATE2(&date,'/',stdout), printf(" is leap year ");
else
OutputDATE2(&date,'/',stdout), printf(" not leap year ");
printf(" date? ");
}
DestructDATE(&date);
system("PAUSE");
return( 0 );
}
Sample Program Dialog EACOURSES CS13111Code ADTs DATEProblem2.exe Construction of DATE2- 3-1954AD date? 1-2-2000a 1/2/2000AD is leap year date? 1/2/100b 1/2/100BC not leap year late? 1.2.2010a /2/2010AD not leap year ate? z truction of DATE = 1-2-2010AD Press any key to continue - . - Sample Program Dialog EACOURSES CS13111Code ADTs DATEProblem2.exe Construction of DATE2- 3-1954AD date? 1-2-2000a 1/2/2000AD is leap year date? 1/2/100b 1/2/100BC not leap year late? 1.2.2010a /2/2010AD not leap year ate? z truction of DATE = 1-2-2010AD Press any key to continueStep by Step Solution
There are 3 Steps involved in it
Step: 1
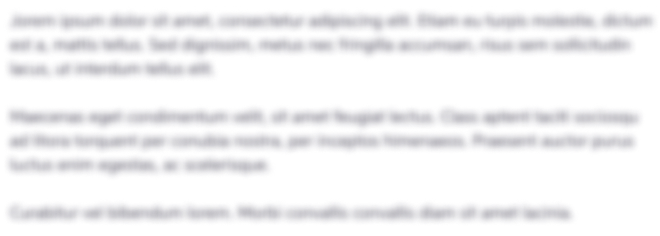
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started