Question
C++ Programming Project: File I/O for Employee Class In this project you will add the ability for objects of your Employee class to write themselves
C++ Programming Project: File I/O for Employee Class
In this project you will add the ability for objects of your Employee class to write themselves out to a file, and be read in from a file. You will also throw an exception if there are any file I/O errors. Get your program working without exception handling first. Then add the exception handling.
The Employee Class
For this project you need to add the following member functions to your Employee class.
Employee read(ifstream&) void write(ofstream&)
The function Employee::read is a static member function. It returns an Employee object based on the data it reads from a file. It must be called as Employee::read(...) since it's job is to create an object. If there is a read error, it throws a std::runtime_error (defined in
The main() function
The major goal of main() is to test the additions to your Employee class. Use the printCheck() function from your previous Employee project. The output of your program will look similar to the output of your previous Employee project.
Your driver will contain a main() function that does the following:
Present the user with a menu of choices, create a data file, or read data from a file and print checks:
This program has two options: 1 - Create a data file, or 2 - Read data from a file and print paychecks. Please enter <1> to create a file or <2> to print checks:
If the user selects the first option, your program should:
- Create an ofstream object using a file name obtained from the user. Pass just the file name as the parameter (no path) so that your program assumes the file to be in the same folder as your executable file.
- Create three employee objects as shown:
Employee joe(37, "Joe Brown", "123 Main St.", "123-6788", 45.00, 10.00)
Employee sam(21, "Sam Jones", "45 East State", "661-9000", 30.00, 12.00)
Employee mary(15, "Mary Smith", "12 High Street", "401-8900", 40.00, 15.00)
- Send messages to each of the three Employee objects to write themselves out to the file.
- Print a message that creation of the file is complete.
- Exit.
If the user selects the second option, your program should:
- Prompt for the name of the file that the program saved.
- Call Employee::read to read in the objects written in step 1, one-by-one.
- Call the printCheck() function for each of the three new objects, just as you did in the previous project.
- Exit.
Run the second option twice. Once with the correct filename, and once with an incorrect one. The second run will test your exception handling. In the error case, throw a std::runtime_error (defined in
In addition, when reading the file for the second part, you need to check that the expected data is there. If there are any errors in the data (there shouldn't be, but you should check anyway), your code should throw a std::runtime_error with a message explaining which data was corrupted.
//main.cpp
#include
#include
int main() { cout.setf(ios::fixed); cout.setf(ios::showpoint); cout.precision(2); Employee joe(37, "Joe Brown", "123-6788", "123 Main St.", 10.00, 45.00); Employee sam(37, "Sam Jones", "661-9000", "45 East State", 12.50, 30.00); joe.printCheck(); sam.printCheck();
}
//employee.cpp
#include
#include
Employee::Employee(void) { employeeNumber = 0; name = "none"; phone = "none"; address = "none"; hourlyWage = 0; hoursWorked = 0; }
Employee::Employee(int empNum,string empName, string empPhone, string empAddress, double wage, double hours) { employeeNumber = empNum; name = empName; phone = empPhone; address = empAddress; hourlyWage = wage; hoursWorked = hours; }
int Employee::getEmployeeNumber() { return employeeNumber; } string Employee::getName() { return name; } string Employee::getAddress() { return address; } string Employee::getPhone() { return phone; } double Employee::getHourlyWage() { return hourlyWage; } double Employee::getHourlyWorked() { return hoursWorked; }
void Employee::setName(string empName) { name = empName; } void Employee::setAddress(string empAddress) { address = empAddress; } void Employee::setPhone(string empPhone) { phone = empPhone; } void Employee::setHourlyWaged(double wage) { hourlyWage = wage; } void Employee::setHourlyWorked(double hours) { hoursWorked = hours; } double Employee::calcPay() { double pay = hoursWorked * hourlyWage; double fedTax = 0.20; double stateTax = 0.075; double overtime = 1.5; int overtimeHours = hoursWorked - 40; if (hoursWorked > 40) { double grossPay = (40 * hourlyWage) + (overtimeHours * (hourlyWage *overtime)); pay = grossPay - ((grossPay * fedTax) + (grossPay * stateTax)); return pay; } else { pay = pay - ((pay * 0.2) + (pay * 0.075)); } return pay; } void Employee::printCheck() { const string DASH1 = ".........."; const string DASH2 = "............"; const string DASH3 = "......"; const string DASH4 = "..........."; const string COMPANY = "UVU Computer Science Dept"; const string PAY = "Pay to the order of "; const string BANK = "United Community Credit Union"; cout << DASH1 << DASH1 << COMPANY << DASH4 << DASH4 << DASH4; cout << endl << endl << endl; cout << PAY << getName() << DASH2 << DASH2 << DASH2 << "$" << calcPay(); cout << endl << endl << endl; cout << BANK << endl; cout << DASH2 << DASH2 << DASH2 << DASH2 << DASH2 << DASH2 << DASH3; cout << endl << endl; cout << "Hours worked: " << getHourlyWorked() << endl; cout << "Hourly wage: " << getHourlyWage(); cout << endl << endl << endl << endl << endl << endl; }
employee.h
#include
#include
class Employee { public: Employee(); Employee(int, string, string, string, double, double); int getEmployeeNumber(); string getName(); string getAddress(); string getPhone(); double getHourlyWage(); double getHourlyWorked(); void setName(string); void setAddress(string); void setPhone(string); void setHourlyWaged(double); void setHourlyWorked(double); double calcPay(); void printCheck();
private: int employeeNumber; string name; string address; string phone; double hourlyWage; double hoursWorked;
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
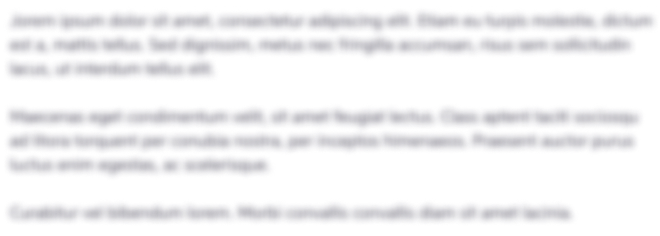
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started