Question
C Programming: Yahtzee consists of 6 rounds. In each round, a player rolls 5 dice with up to two re-rolls. The player chooses which dice
C Programming:
Yahtzee consists of 6 rounds. In each round, a player rolls 5 dice with up to two re-rolls. The player chooses which dice to save and which dice to re-roll. At the end of the round, the player picks a category between 1 and 6 to save their score to. Each die that matches the category adds to the score of that category. Each category is picked and scored exactly once. For example, if a round ends with 1 6 3 4 6 and the player chooses category 6, the score for category 6 is 6 + 6 = 12. If the player had chosen category 3, the score would be 3. If the player had chosen category 5, the score would be 0.
Write the body of functions that are marked with a comment that begins with
// Homework TODO: ...
#include
// Constants used throughout the program.
#define NUM_DICE 5 #define NUM_FACES 6 #define UNSCORED_CATEGORY -1
// Sets up the game. Seed rand and mark every category as unscored void initializeGame(int categoryScores[NUM_FACES]);
// Marks all die as unsaved. Used at the start of a round void resetsavedDice(bool savedDice[NUM_DICE]);
// Play one round of simple yahtzee void playRound(bool savedDice[NUM_DICE], int dice[NUM_DICE]);
// Obtains saved dice from player void getPlayerSaveDice(bool savedDice[]);
// Re-roll all die that are not saved void rollAllUnsavedDice(bool savedDice[NUM_DICE], int dice[NUM_DICE]);
// Ask user for category and score round for that category if available void scoreRound(int dice[NUM_DICE], int categoryScores[NUM_FACES]);
// Prints the current dice rolls and re-rolls remaining void printRoundState(int dice[NUM_DICE], int roll);
// Prints current total score of all saved categories void printTotalScore(int categoryScores[NUM_FACES]);
// Flush stdin buffer void flushStdin();
// Plays simple yahtzee // // Simple yahtzee consists of 6 rounds. In each round, 5 dice are rolled, // and they may be re-rolled twice. The player saves dice they wish to keep // which will not be re-rolled. int main() { int dice[NUM_DICE], categoryScores[NUM_FACES]; bool savedDice[NUM_DICE]; initializeGame(categoryScores); // Play and score all rounds for (int gamesPlayed = 0; gamesPlayed < NUM_FACES; ++gamesPlayed) { playRound(savedDice, dice); scoreRound(dice, categoryScores); } printf("Game over. "); }
////////////////////////////////// //// INITIALIZATION FUNCTIONS ////
// Sets up the game. Seed rand and mark every category as unscored
void initializeGame(int categoryScores[NUM_FACES]) { for (int i = 0; i < NUM_FACES; ++i) { categoryScores[i] = UNSCORED_CATEGORY; } int seed; printf("Enter seed: "); scanf("%d", &seed); // Flush the input buffer after every scanf call. flushStdin();
srand(seed); }
// Marks all die as unsaved. Used at the start of a round void resetsavedDice(bool savedDice[NUM_DICE]) { for (int i = 0; i < NUM_DICE; i++) { savedDice[i] = false; } }
////////////////////////////////////// //// END INITIALIZATION FUNCTIONS ////
/////////////////////////////////// //// PLAYING A ROUND FUNCTIONS ////
// Play one round of simple yahtzee void playRound(bool savedDice[NUM_DICE], int dice[NUM_DICE]) { resetsavedDice(savedDice); int roll = 0; // Initial roll before asking to save dice rollAllUnsavedDice(savedDice, dice); printRoundState(dice, 2); for (int roll = 0; roll < 2; ++roll) { getPlayerSaveDice(savedDice); rollAllUnsavedDice(savedDice, dice); printRoundState(dice, 1 - roll); } }
// Obtains saved dice from player void getPlayerSaveDice(bool savedDice[]) { char option; int die; bool reroll = false; while (!reroll) { printf("Enter dice to save or 'r' for reroll: "); // Read input and clear input buffer afterwards int scanfResult = scanf("%c", &option); flushStdin(); // If some character successfully read if (scanfResult == 1) { // If user requests a reroll if (option == 'r') { reroll = true; continue; } // Else if user toggles saving of a die else if (isdigit(option) && 1 <= (die = atoi(&option)) && die <= NUM_DICE) { savedDice[die - 1] = !savedDice[die - 1]; printf("Die %d is now %s ", die, savedDice[die - 1] ? "saved" : "unsaved"); continue; } } // Otherwise re-prompt for input printf("Error: invalid command. Enter 'r' for re-roll unsaved die or 1-5 to toggle saving die "); } }
// Re-roll all die that are not saved void rollAllUnsavedDice(bool savedDice[NUM_DICE], int dice[NUM_DICE]) { for (int i = 0; i < NUM_DICE; i++) { if (!savedDice[i]) { dice[i] = 1 + rand() % NUM_FACES; } } }
// Ask user for category and score round for that category if available void scoreRound(int dice [NUM_DICE], int categoryScores[NUM_FACES]) { // Homework TODO: write code for this function. // This function should: // 1) Ask the user for a category to save the round score to // 2) Check if the input category is scored or not using UNSCORED_CATEGORY // 3) Score the result for category i in categoryScores[i - 1] // 4) Print the current total score }; /////////////////////////////////////// //// END PLAYING A ROUND FUNCTIONS ////
//////////////////////////// //// PRINTING FUNCTIONS ////
// Prints the current dice rolls and re-rolls remaining void printRoundState(int dice[NUM_DICE], int rerollsLeft) { // Homework TODO: write code for this function. // Example output, if all dice are 1 and 2 rerolls are left: // Dice: 1 1 1 1 1 // Rolls left: 2 }
// Prints current total score of all saved categories void printTotalScore(int categoryScores[NUM_FACES]) { // Homework TODO: write code for this function. // Example output, if the score for category i is 2i except // for category 2, which is unscored: // Category 1 score: 1 // Category 2 score: not scored // Category 3 score: 6 // Category 4 score: 8 // Category 5 score: 10 // Category 6 score: 12 // Total Score: 27 }
//////////////////////////////// //// END PRINTING FUNCTIONS ////
// Flush stdin buffer void flushStdin() { char c; // Skip all characters until end-of-file marker // or new line / carriage return while ( (c = getchar()) != EOF && c != ' ' && c != ' ' ) {}; }
Example Output :
Enter seed: 10 Dice: 5 4 2 5 6 Rolls left: 2
Enter dice to save or r for reroll: 1 Die 1 is now saved Enter dice to save or r for reroll: a Error: invalid command. Enter r for re-roll unsaved die or 1-5 to toggle saving die Enter dice to save or r for reroll: 0
Error: invalid command. Enter r for re-roll unsaved die or 1-5 to toggle saving die Enter dice to save or r for reroll: 4 Die 4 is now saved Enter dice to save or r for reroll: r
Dice: 5 1 1 5 3 Rolls left: 1 Enter dice to save or r for reroll: r Dice: 5 3 2 5 4 Rolls left: 0 Enter category to save score: 5 Category 1 score: not scored Category 2 score: not scored Category 3 score: not scored Category 4 score: not scored Category 5 score: 10 Category 6 score: not scored Total Score: 10 Dice: 4 5 3 1 4 Rolls left: 2 Enter dice to save or r for reroll: 1 Die 1 is now saved Enter dice to save or r for reroll: 5 Die 5 is now saved Enter dice to save or r for reroll: r Dice: 4 5 5 2 4 Rolls left: 1
...
Enter dice to save or r for reroll: 5 Die 5 is now saved Enter dice to save or r for reroll: r Dice: 1 6 5 6 6
Rolls left: 1 Enter dice to save or r for reroll: a Error: invalid command. Enter r for re-roll unsaved die or 1-5 to toggle saving die Enter dice to save or r for reroll: r Dice: 1 6 4 6 6 Rolls left: 0 Enter category to save score: 5 Error: invalid command. Enter 1-6 to save to an unused category Enter category to save score: a Error: invalid command. Enter 1-6 to save to an unused category Enter category to save score: 6 Category 1 score: 2 Category 2 score: 8 Category 3 score: 12 Category 4 score: 8 Category 5 score: 10 Category 6 score: 18 Total Score: 58 Game over.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
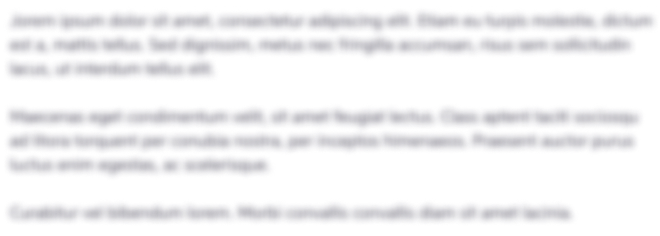
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started