Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C + + Revise the Student Roster Queue class to a template version, utilizing the provided files. Implement exception handling in the code. / /
C Revise the Student Roster Queue class to a template version, utilizing the provided files. Implement exception handling in the code.
Drivercpp
#include
#include
#include "StudentRosterQueue.h
int main
StudentRosterQueue sr StudentRosterQueue;
ifstream inputFile;
inputFile.openStudentstxt;
StudentNode s;
while inputFile.eof
Student s;
getlineinputFile sname, t;
inputFile sssn sgrade;
inputFile ws;
srenqueues;
inputFile.close;
srprintListcout;
Student temp;
srdequeuetemp;
srprintListcout;
return ;
StudentRosterQueueh
#pragma once
#include
using namespace std;
struct Student
string name;
int ssn;
char grade;
;
struct StudentNode
Student item;
StudentNode next;
;
class EmptyStack : public exception
public:
const char what
return "Stack is Empty, cannot dequeue!!
;
;
class FullStack : public exception
public:
const char what
return "Stack is Full, or not enough memory, cannot enqueue!!
;
;
class StudentRosterQueue
private:
StudentNode qFront;
StudentNode qRear;
public:
StudentRosterQueue;
~StudentRosterQueue;
void dequeueStudent& x;
void enqueueStudent x;
bool isFull;
bool isEmpty;
void printListostream&;
;
StudentRosterQueue::StudentRosterQueue
qFront NULL;
StudentRosterQueue::~StudentRosterQueue
while isEmpty
Student temp;
dequeuetemp;
bool StudentRosterQueue::isEmpty
if qFront NULL
return true;
else
return false;
bool StudentRosterQueue::isFull
StudentNode test;
try
test new StudentNode;
delete test;
return false;
catch std::badalloc exception
return true;
void StudentRosterQueue::enqueueStudent s
if isFull
throw FullStack;
StudentNode currPos new StudentNode;
currPositem s;
currPosnext NULL;
if isEmpty
qFront currPos;
else
qRearnext currPos;
qRear currPos;
void StudentRosterQueue::dequeueStudent& s
if isEmpty
throw EmptyStack;
s qFrontitem;
StudentNode oldTop qFront;
qFront qFrontnext;
delete oldTop;
void StudentRosterQueue::printListostream&
StudentNode currPtr qFront;
while currPtr NULL
cout currPtritem.name currPtritem.ssn currPtritem.grade endl;
currPtr currPtrnext;
cout endl;
Studentstxt
Betsy A
Sam Spade B
Annie Smith A
Thomas J Jones A
Allan Adaire C
Jennifer Smallville A
Step by Step Solution
There are 3 Steps involved in it
Step: 1
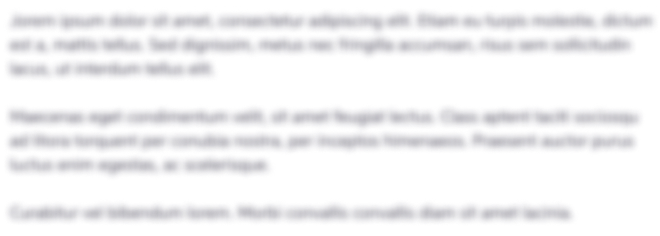
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started