Question
C++ -------------------------------------------------------------------------------------------------------------------------- Song.h -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- MusicLibrary.h -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- PlayList.h -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- PlayListEntry.h -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- Song.cpp -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- MusicLibrary.cpp -------------------------------------------------------------------------------------------------------------------------- -------------------------------------------------------------------------------------------------------------------------- PlayList.cpp #include #include PlayList.h PlayList::PlayList
C++
--------------------------------------------------------------------------------------------------------------------------
Song.h
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
MusicLibrary.h
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
PlayList.h
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
PlayListEntry.h
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
Song.cpp
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
MusicLibrary.cpp
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
PlayList.cpp
#include
PlayList::PlayList () { firstSong=nullptr; lastSong=nullptr; numSongs = 0; playTime = 0; } PlayList::PlayList(PlayList& other) { // implement copy-constructor }
PlayList::~PlayList() { // implement destructor }
int PlayList::getPlayListLength() const { return playTime; } int PlayList::getNumSongs() const { return numSongs; }
void PlayList::printList() const { // implement printList() }
PlayListEntry *PlayList::getSong( const int pos ) { // implement getSong() }
void PlayList::appendSong(Song *song) { // implement appendSong()
}
void PlayList::deleteSong(Song *song) { // implement deleteSong }
void PlayList::moveUp(PlayListEntry *song) { // implement moveUp }
void PlayList::reverseOrder() { // implement function reversing the order of Songs }
--------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------
main.cpp
#include
#include "Song.h" #include "MusicLibrary.h" #include "PlayList.h"
using namespace std;
int main(int argc, char** argv) { string filename; int numsongs; int option=0; cout > filename;
cout > option;
if ( option > 2 ) { cout
MusicLibrary mylibrary(numsongs); mylibrary.readSongsFromFile(filename);
// Playlist 1: create a playlist with all songs if ( option == 0 ) { PlayList list1; for ( int i=0; i
if ( option == 1 ) { // Playlist2: only U2 songs PlayList list2; for ( int i=0; i getArtist() == "U2" ) { list2.appendSong (song); } } list2.printList(); }
if ( option == 2 ) { PlayList list1; for ( int i=0; i
--------------------------------------------------------------------------------------------------------------------------
The purpose of this assignment is to developed the code to manage a music playlist. The playlist has to be implemented as a doubly linked list. The code for the assignment is organized as follows: Class Song This class provides the abstraction for an individual song, including song title, artist, album, year of publication, and play time in seconds. The class further provides a method printSong which displays relevant information for a Song. Code is provided in Song.h and Song.cpp. Students should not modify these files, but use 'as is'. Class MusicLibrary This class provides the abstraction and implementation for a Music Library, including reading Songs from a file and storing them in the library. Code is provided in MusicLibrary.h and MusicLibrary.cpp. Students should not modify these files, but use 'as is'. Class Playlist This class provides the abstraction for the Playlist. A Playlist is managed as a double linked list, with the content being a *pointer to a Song *in the Music Library. The abstraction for the linked list node is in the class PlayListEntry (file PlayListEntry.h). The header file PlayList.h is fully implemented and does not require any changes. All the work is expected to happen in the file PlayList.cpp. Specifically, students are expected to implement the following functionality: implement the method 'appendSong(' which appends a Song to the doubly linked list. This function is expected to allocate a new PlayListEntry object, correctly store the pointer to the Song in the object, update the prev and next pointers in the new PlayListEntry as well as already existing PlayListEntry s (if required), update the number of Songs and the total playtime of the Playlist, as well as the pointers to the first and the last Song in the PlayList (if required). Difficulty level: 3 (scale 1-5) implement the method 'getSong0' which returns a pointer to n-th node in the linked, with n being provided as an input argument. If the linked list does not contain n nodes and no pointer to a Song can be returned, the function is expected to return an error message, e.g. if the playlist contains 4 Songs but the code requests song at Position 5: Cannot return song at position 5 numSongs is 4. Note: the first song in the linked list is at position 0. Difficulty level: 2 (scale 1-5) implement the method 'deleteSong(': the method is expected to identify a PlayListEntry given a pointer to a Song, remove the corresponding PlayListEntry from the linked list correctly (i.e. making sure that all prev and next pointers of nearby elements are correctly updated, and numSongs and playTime is updated correctly). If the playlist is empty, the function is expected to return the error Could not find Song. Playlist is empty. If the song identified by the pointer is not part of the playlist, the function is expected to return the error message: Could not find song in Playlist. Difficulty level: 3 (scale 1-5) implement method 'printList(' which invokes the printSong, method of each Song in the playlist in the same order as stored in the playlist Difficulty level: 2 (scale 1-5) implement the method 'moveUpO' which moves a song identified by a PlayListEntry pointer one sport up in the playlist. If the Song identified by the PlayListEntry is already the first Song in the list, the function is expected to output Already at top of Playlist, cannot move up. and no changes to the linked list are performed. Difficulty level: 4( scale 1-5) implement the copy constructor of the Playlist class. Difficulty level: 4 (scale 1-5) implement the method 'reverseOrder()". This function is expected reverse the order of songs in the playlist, i.e. the last song becomes the first song, the second last song will be the second song..., the first song becomes the last song. Difficulty level: 4.5( scale 1-5) Class PlayListEntry This class provides the abstraction required to manage songs in double linked list implemented in the Playlist class. Code is provided in PlayListentry.h. Students should not modify this files, but use 'as is'. main.cpp A main file providing some examples on how the Playlist class is expected to be used is provided. You will most likely have to make changes to this file as part of the development process (e.g. to test some of the functions that you developed in more details), but please DO NOT upload your modification main.cpp to zybooks. The original main.cpp file is expected as part of the zybook tests. IMPORTANT Classes and methods names must match exactly for unit testing to succeed. Submissions with hard coded answers will receive a grade of 0. 1 #ifndef SONG_H #define SONG_H 2 3 4 #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
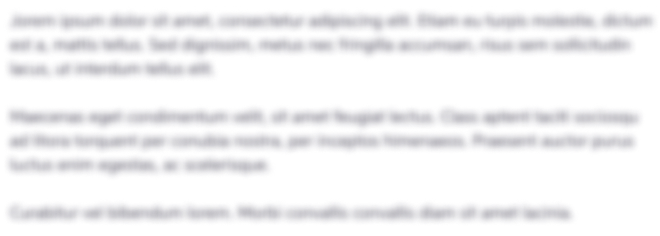
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started