Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C++ / Thank you in advance! Use the code developed below and: Implement a function to apply the Quicksort algorithm. Driver Program: (1) Modify the
C++ / Thank you in advance!
Use the code developed below and:
Implement a function to apply the Quicksort algorithm.
Driver Program:
(1) Modify the classes code (if needed) and write a program to sort the collection of Items based on their total price.
(2) After the collection is sorted, use a loop to print the name, department and total price of each element in the Items list.
The code:
#include#include #include #include using namespace std; //abstract class Item class Item { public: virtual double GetTotalPrice() = 0; }; //Enum for discount type enum Discount_Type { amount, percentage }; //to store all the department id to check for duplication set departmentID; //Department class which contains id, name,room phone number and parent department class Department { string id; string name; int room; string phoneNumber; Department* parent; public: //Empty constructor Department() {} //parametrized constructor Department(string id, string name, int room, string phoneNumber, Department* parent) { //check for duplication of id if (departmentID.count(id) > 0) { cout << "Oject Not created Due to Department ID already exist "; return; } else { departmentID.insert(id); } this->id = id; this->name = name; this->room = room; this->phoneNumber = phoneNumber; this->parent = parent; } //this will return all the values of the object for printing string toString() { string output = " "; output += "\tId: " + id + " "; output += "\tName: " + name + " "; output += "\tRoom: " + to_string(room) + " "; output += "\tPhoneNumber: " + phoneNumber + " "; if (parent != NULL) { output += "Parent Department: " + parent->toString(); } return output; } }; //Product class whih Contains name, description category, department, price, discount,discount type class Product :public Item{ protected: string name; string description; string category; Department department; double price; double discount; int discount_type; public: //empty constructor Product() {} //parametrized constructor Product(string name, string description, string category, Department department, float price, float discount, Discount_Type discount_type) { //if price value is negative then it returns with a messsage; if (price < 0) { cout << "No object create due to negative value of price "; return; } //if discount value is negative then it returns with a messsage; if (discount < 0) { cout << "No object create due to negative value of discount "; return; } //if every thing fine then we just simply add values to the variables this->name = name; this->description = description; this->category = category; this->department = department; this->price = price; this->discount = discount; this->discount_type = discount_type; } //this will return the total price after neglect the discount virtual double GetTotalPrice() { //if discount is of type amount if (discount_type == amount) { double finalP = price - discount; if (finalP < 0) { cout << "Total Price cannot be negative"; return 0; } else { return finalP; } } else { //if the discount is of the type percentage double finalP = price - (price * discount / 100); if (finalP < 0) { cout << " Total Price cannot be negative "; return 0; } else { return finalP; } } } //return the values of each variable to print in string form virtual string toString() { string output = ""; output += "Name: " + name + " "; output += "Description: " + description + " "; output += "Category: " + category + " "; output += "Department: " + department.toString() + " "; double totalPrice = this->GetTotalPrice(); output += "Total Price " + to_string(totalPrice) + " "; return output; } }; //Service class which contains duration and it import the class Product in public accessor mode class Service : public Product { double duration; //Call the product i.e. super class constructor public: Service(string name, string description, string category, Department department, double duration, double rate, double rate_discount, Discount_Type discount_type) :Product(name, description, category, department, rate, rate_discount, discount_type) { this->duration = duration; } //this will return all the variables value virtual string toString2() { string output = ""; output += toString(); output += "Duration: " + to_string(duration) + " "; return output; } }; int main() { //create vector of all the class vector department; //Remove the Products and Services vectors /*vector product; vector service;*/ //Add a new vector called Items, //which contains pointers to Item instances vector - items; // add 3 objects of departments Department d1("ABCD-EFGH-1234", "Electronics", 260, "0888123456", NULL); Department d2("ABCD-EFGH-8965", "Mechanical", 452, "0963258741", NULL); Department d3("ABCD-EFGH-7412", "IT", 153, "0258777412", &d1); //add department object to the vector department.push_back(d1); department.push_back(d2); department.push_back(d3); //Add at least 3 products and 3 services to the Items collection //create object of products Product *p1= new Product("PC Tools", "Tools used to design, repair or develop an PC", "Games", d3, 185.99, 30, amount); Product *p2= new Product("Car Tools", "Tools used to repair cars i.e. open and closing", "Repair", d2, 199.99, 15, percentage); Product *p3 = new Product("Bulb", "Led bulb operate on very low power", "Electonics", d1, 400.56, 80, amount); //add product objects to the vector items.push_back(p1); items.push_back(p2); items.push_back(p3); //Create objects of service Service *s1 = new Service("Repairing Cars", "Repairing of Cars and automobile", "Cars", d2, 2, 20.20, 10, percentage); Service *s2= new Service("Web Developer", "crete any web app or android app", "IT", d3, 12, 500, 50, amount); Service *s3 = new Service("Repairing of Electronics tools", "Repairing of CPU,montior", "Electornics", d1, 1.5, 8.50, 30, percentage); //add service objects to vector items.push_back(s1); items.push_back(s2); items.push_back(s3); //Use a loop to calculate and display the total //prices of all elements in the Items collection double totalPrice = 0; for (int i = 0; i < items.size(); i++) { totalPrice += items[i]->GetTotalPrice(); } cout << "Total Price: $" << totalPrice << endl; //print product vector data using for loop //cout << "Products "; //cout << "================================ "; //for (int i = 0; i < items.size(); i++) { // if (Product* p = dynamic_cast
(items[i])) { // cout << dynamic_cast (items[i])->toString(); // cout << endl; // cout << "======================================== "; // } //} ////print service vector using for loop //cout << " Services "; //cout << "================================ "; //for (int i = 0; i < items.size(); i++) { // if (Service* s = dynamic_cast (items[i])) { // cout << dynamic_cast (items[i])->toString2(); // cout << endl; // cout << "======================================== "; // } //} //check duplication of id //cout << " Check duplication of id "; //Department d4("ABCD-EFGH-7412", "IT", 153, "0258777412", &d1); ////check for negative price entered //cout << " check for negative price entered "; //Product p4("PC Tools", "Tools used to design, repair or develop an PC", "Games", d3, -185.99, 30, amount); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
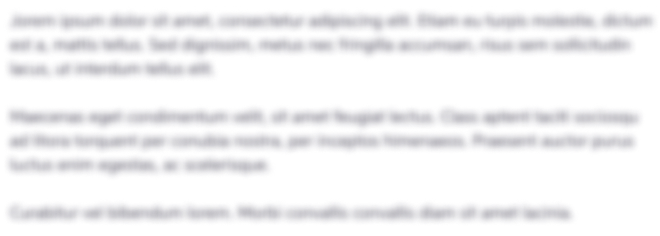
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started