Question
C# Your task is to create a Student class that implements the IComparable interface and has the following public methods: public Student(string firstName, string lastName,
C#
Your task is to create a Student class that implements the IComparable interface and has the following public methods:
public Student(string firstName, string lastName, string degree, int grade)
The constructor for Student provides the first name, last name, degree and grade (on the 1-7 scale) of this student. All of these must be stored in private fields.
public override string ToString()
This method is part of the Object class, which every class inherits from, and which is used whenever an object needs to be converted to a string.
This method should return a string containing all 4 private fields of Student in the following format:
"{last name}, {first name} ({degree}) Grade: {grade}"
For example, Jane Smith (who is studying a Bachelor of IT) and has a grade of 7 will be represented as:
"Smith, Jane (Bachelor of IT) Grade: 7"
public int CompareTo(object obj)
This is the CompareTo() method that is required by the IComparable interface.
It must return a number less than, equal to or greater than zero if obj should compare to less than, equal to or greater than the object that CompareTo() is being called on.
Note that you will need to cast obj to a Student in order to do the comparison. See the paragraph below for the order the fields should be used in.
Once a class is correctly implementing the IComparable interface, that class's objects can now be compared to each other, making methods like Array.Sort() work correctly for those objects.
You are provided with a Main() method that creates an array of Student objects, calls Array.Sort() on them and displays the list. You can use this to test that your CompareTo() method is functioning as required.
The sort order your CompareTo() method should implement is the following:
Last names in descending order, followed by...
First names in descending order, followed by...
Degrees in ascending order, followed by...
Grades in ascending order.
When you run the provided program with your Student class, if you implemented the CompareTo() method correctly, you should see this:
Smith, John (Bachelor of Engineering) Grade: 6 Smith, John (Bachelor of Engineering) Grade: 7 Smith, John (Bachelor of IT) Grade: 6 Smith, John (Bachelor of IT) Grade: 7 Smith, Jane (Bachelor of Engineering) Grade: 6 Smith, Jane (Bachelor of Engineering) Grade: 7 Smith, Jane (Bachelor of IT) Grade: 6 Smith, Jane (Bachelor of IT) Grade: 7 Bloggs, John (Bachelor of Engineering) Grade: 6 Bloggs, John (Bachelor of Engineering) Grade: 7 Bloggs, John (Bachelor of IT) Grade: 6 Bloggs, John (Bachelor of IT) Grade: 7 Bloggs, Jane (Bachelor of Engineering) Grade: 6 Bloggs, Jane (Bachelor of Engineering) Grade: 7 Bloggs, Jane (Bachelor of IT) Grade: 6 Bloggs, Jane (Bachelor of IT) Grade: 7
Press enter to exit.
Sample code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace StudentGradeOrder { class Student : IComparable { // Implement the Student class here // ... } class Program { static void Main(string[] args) { Student[] students = new Student[] { new Student("Jane", "Smith", "Bachelor of Engineering", 6), new Student("John", "Smith", "Bachelor of Engineering", 7), new Student("John", "Smith", "Bachelor of IT", 7), new Student("John", "Smith", "Bachelor of IT", 6), new Student("Jane", "Smith", "Bachelor of IT", 6), new Student("John", "Bloggs", "Bachelor of IT", 6), new Student("John", "Bloggs", "Bachelor of Engineering", 6), new Student("John", "Bloggs", "Bachelor of IT", 7), new Student("John", "Smith", "Bachelor of Engineering", 6), new Student("Jane", "Smith", "Bachelor of Engineering", 7), new Student("Jane", "Bloggs", "Bachelor of IT", 6), new Student("Jane", "Bloggs", "Bachelor of Engineering", 6), new Student("Jane", "Bloggs", "Bachelor of Engineering", 7), new Student("Jane", "Smith", "Bachelor of IT", 7), new Student("John", "Bloggs", "Bachelor of Engineering", 7), new Student("Jane", "Bloggs", "Bachelor of IT", 7), };
Array.Sort(students); foreach (Student student in students) { Console.WriteLine("{0}", student); }
Console.WriteLine(" Press enter to exit."); Console.ReadLine(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
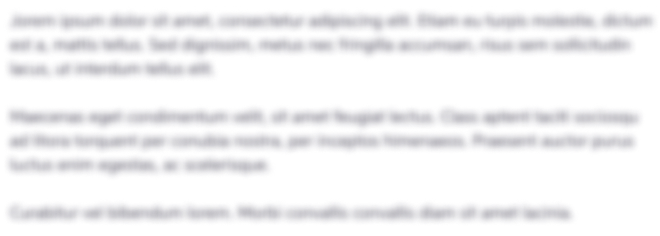
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started