Question
Caesar Cipher C# Csharp Your Program For your lab, you will create a menu-driven program to encrypt and decrypt messages according to a simple Caesar
Caesar Cipher C# Csharp
Your Program
For your lab, you will create a menu-driven program to encrypt and decrypt messages according to a simple Caesar cipher. The Caesar cipher is a simple substitution cipher where each letter in the message is shifted a certain number of places down the alphabet, according to a numeric key. Decrypting these messages is simple if you know the key that encrypted it you simply shift each letter in the opposite direction the same amount.
To start, use a do-while loop to create a program that displays the menu until the user chooses the exit option. Your menu should include 4 options:
1. Encrypt a message
2. Decrypt a message
3. Change the key
4. Exit
Furthermore, after the menu, you should display the value of the currently selected key. Initialize this variable to 0, so the program initially will start with a key of 0. However, if the key is 0, you should display an additional message informing the user that a key of 0 has no noticeable effect and should be changed before encrypting or decrypting a message. This can be seen in the sample output. Test your program with just the menu loop by ensuring the menu loop is working without issue before progressing, you simplify the process of testing this program.
After the menu loop is working, add a switch statement inside the loop to handle the different option selections. Your switch will have 4 cases and a default case, for options 1, 2, 3, 4, and anything else.
If the user selects an invalid option, use the default case to tell them it is an invalid option.
If the user selects 4, do nothing. The program should just exit after the user selects 4.
If the user selects 1, 2, or 3, you will pass control of the program to a separate method for handling the selected behavior. More information on these methods will follow.
Note: To test your program, use case 1, 2, and 3 to display a simple output statement at first. This will allow you to test the switch and make sure it is working before writing all of the methods below. You can go back and add in the method calls as appropriate as you write them.
Next, proceed to writing the methods. The first you should write is a static method to allow the user to select a key. I recommend a name such as select_Key. This method will accept no parameters, and will need to return an integer the value for the key. Inform the user that a valid key is between 0 and 25, and then prompt them to enter a key. If the user enters a larger value, this doesnt have to be an error. We can restrict the larger value to the 0-25 range by using modulus.
Next, write the encrypt and decrypt method. I recommend names such as encrypt and decrypt. These methods will return no values, but will accept the selected key as an integer parameter. Refer to the sample output for formatting and messaging suggestions. Allow the user to enter a message to encrypt or decrypt.
Once you have the message stored in a string variable, you will need to convert it to a char[] array. To do this, declare a new char[], and convert the string using string.ToCharArray(). You can refer to the sample program for the specific syntax on doing this. Then, use a loop (type is up to you, although a for loop is ideal for this for now) to loop through all the array positions. This will start at 0, because arrays start at subscript 0, and continue while the counter is less than the arrays length (obtainable through the Length property).
For each character in the array (identified by each position in the array), you will need to add the key value to the character value to encrypt the message, or subtract the key value to decrypt the message. (These two functions will be very similar to each other). This exploits the ASCII value of the characters for easy encryption.
During the conversion, keep the following hints in mind for a more robust program:
Dont worry about case. Use Char.ToUpper(char) to convert each character to its upper case form before adding or subtracting the key value from it. The output will be in all capitals, but this greatly simplifies the problem.
If the character is not a letter, dont do anything to it. You can use an if statement inside the loop to handle this, and you can check if the character is a letter by seeing if it is between the values of 65 (A) and 90 (Z). This way, punctuation and digits will remain unchanged.
When adding the integer key value to the char from the array, the result is an int. This means assigning it back to the char array position will generate an answer. To resolve this, we can use Convert.ToChar() to convert the integer value back into a char. Assuming an arrayed named msgArray, the syntax would look like msgArray[index] = Convert.ToChar(msgArray[index] + key);
During encryption, if the value after adding the key is GREATER than 90, you will need to subtract 26 from it to bring it back into the alphabet range of ASCII values. This will simulate wrapping around from Z back to A. During decryption, you will add 26 if the value after subtracting the key is LESS than 65.
After the array is converted, in order to simplify printing out the msg, convert it back into a string. The easiest way to do this is to assign a string variable the value of a new string(char[]). Assuming a string named msg and a char[] msgArray, msg = new string(msgArray);
As you write each function, you can change the switch statement in the main() to call that function, and then test your code. By working incrementally, you can handle the errors as they arise, as opposed to having many all at once at the end.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
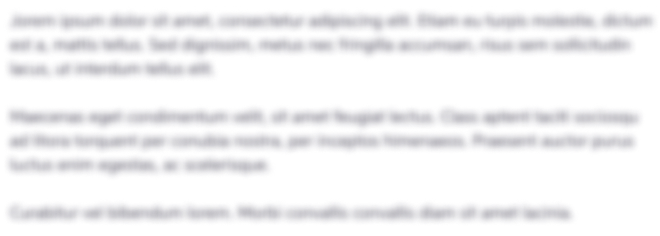
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started