Question
Can anyone convert this Java code to C++ please? import java.util.Random; class Player { private String name; private int score; // when a player is
Can anyone convert this Java code to C++ please?
import java.util.Random;
class Player {
private String name;
private int score;
// when a player is created, it's initial score is set to zero
public Player(String name) {
this.name = name;
this.score = 0;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
class Roll {
Random random = new Random();
public static int getDice1Result() {
Random r = new Random();
return r.nextInt((6 - 1) + 1) + 1;
}
public static int getDice2Result() {
Random r = new Random();
return r.nextInt((6 - 1) + 1) + 1;
}
}
public class Skunk {
private static Player user;
private static Player computer1;
private static Player computer2;
private static Player computer3;
public Skunk() {
user = new Player("user");
computer1 = new Player("computer1");
computer2 = new Player("computer2");
computer3 = new Player("computer3");
}
public void showScores() {
System.out.println("$$$$$$$$$$$$$$$$$$$$$$$ Current Score $$$$$$$$$$$$$$$$$$$$$$$$$$$$$$$$$$");
System.out.println("Score for user is: " + user.getScore());
System.out.println("Score for computer1 is: " + computer1.getScore());
System.out.println("Score for computer2 is: " + computer2.getScore());
System.out.println("Score for computer3 is: " + computer3.getScore() + " ");
}
public static void main(String[] args) {
System.out.println("Welcome to the game of Skunk.. ");
Skunk skunk = new Skunk();
while (true) {
System.out.println("user is rolling.. ");
int counter = 1;
while (true) {
if (user.getScore() >= 100) {
System.out.println("user has won!");
return;
}
System.out.print("Roll " + counter + " for user = ");
int dice1 = Roll.getDice1Result();
int dice2 = Roll.getDice2Result();
System.out.println("Roll " + counter + " [" + dice1 + ", " + dice2 + "]");
// If it is single skunk, points for this roll is not added and
// turn for player ends
if (dice1 == 1 || dice2 == 1) {
System.out.println("user got a single skunk.. ");
break;
}
// If it is double skunk, total points for user is set to zero
// and
// turn for player ends
if (dice1 == 1 && dice2 == 1) {
System.out.println("user got a double skunk.. ");
user.setScore(0);
break;
}
user.setScore(user.getScore() + dice1 + dice2);
counter++;
skunk.showScores();
}
skunk.showScores();
counter = 1; // setting counter back to 1
System.out.println("computer1 is rolling.. ");
while (true) {
if (computer1.getScore() >= 100) {
System.out.println("computer1 has won!");
return;
}
// computer1 ends it's turn after three rolls
if (counter == 4) {
System.out.println("computer1 ended his turn.. ");
break;
}
System.out.print("Roll " + counter + " for Computer1 = ");
int dice1 = Roll.getDice1Result();
int dice2 = Roll.getDice2Result();
System.out.println("Roll " + counter + " [" + dice1 + ", " + dice2 + "]");
// If it is single skunk, points for this roll is not added and
// turn for player ends
if (dice1 == 1 || dice2 == 1) {
System.out.println("computer1 got a single skunk.. ");
break;
}
// If it is double skunk, total points for user is set to zero
// and
// turn for player ends
if (dice1 == 1 && dice2 == 1) {
System.out.println("computer1 got a double skunk.. ");
user.setScore(0);
break;
}
computer1.setScore(computer1.getScore() + dice1 + dice2);
counter++;
skunk.showScores();
}
skunk.showScores();
counter = 1;
System.out.println("computer2 is rolling.. ");
int scoreDuringAturn = 0;
while (true) {
if (computer2.getScore() >= 100) {
System.out.println("Computer2 has won!");
return;
}
if (scoreDuringAturn >= 20) {
System.out.println("computer2 dropped his turn as it earned 20 or more points during his turn ");
break;
}
System.out.print("Roll " + counter + " for Computer2 = ");
int dice1 = Roll.getDice1Result();
int dice2 = Roll.getDice2Result();
System.out.println("Roll " + counter + " [" + dice1 + ", " + dice2 + "]");
// If it is single skunk, points for this roll is not added and
// turn for player ends
if (dice1 == 1 || dice2 == 1) {
System.out.println("computer2 got a single skunk.. ");
break;
}
// If it is double skunk, total points for user is set to zero
// and
// turn for player ends
if (dice1 == 1 && dice2 == 1) {
System.out.println("computer2 got a double skunk.. ");
user.setScore(0);
break;
}
computer2.setScore(computer2.getScore() + dice1 + dice2);
scoreDuringAturn += dice1 + dice2;
counter++;
skunk.showScores();
}
skunk.showScores();
counter = 1;
System.out.println("computer3 is rolling.. ");
while (true) {
if (computer3.getScore() >= 100) {
System.out.println("computer3 has won!");
return;
}
System.out.print("Roll " + counter + " for Computer3 = ");
int dice1 = Roll.getDice1Result();
int dice2 = Roll.getDice2Result();
System.out.println("Roll " + counter + " [" + dice1 + ", " + dice2 + "]");
// If it is single skunk, points for this roll is not added and
// turn for player ends
if (dice1 == 1 || dice2 == 1) {
System.out.println("computer3 got a single skunk.. ");
break;
}
// If it is double skunk, total points for user is set to zero
// and
// turn for player ends
if (dice1 == 1 && dice2 == 1) {
System.out.println("computer3 got a double skunk.. ");
user.setScore(0);
break;
}
computer3.setScore(computer3.getScore() + dice1 + dice2);
scoreDuringAturn += dice1 + dice2;
counter++;
skunk.showScores();
}
skunk.showScores();
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
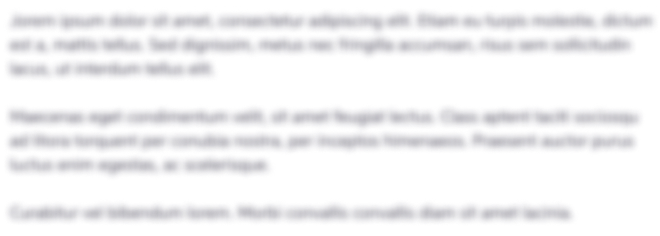
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started