Question
Can be done in Python 3 or C++: Main code in Python: def BinaryProduct(multiplicand, multiplier, nbits, representation): # Write your code here if __name__ ==
Can be done in Python 3 or C++:
Main code in Python:
def BinaryProduct(multiplicand, multiplier, nbits, representation):
# Write your code here
if __name__ == '__main__':
fptr = open(os.environ['OUTPUT_PATH'], 'w')
multiplicand = input()
multiplier = input()
nbits = int(input().strip())
representation = input()
result = BinaryProduct(multiplicand, multiplier, nbits, representation)
fptr.write(' '.join([' '.join(x) for x in result]))
fptr.write(' ')
fptr.close()
Main code in C++:
vector> BinaryProduct(string multiplicand, string multiplier, int nbits, string representation) {
}
int main()
{
ofstream fout(getenv("OUTPUT_PATH"));
string multiplicand;
getline(cin, multiplicand);
string multiplier;
getline(cin, multiplier);
string nbits_temp;
getline(cin, nbits_temp);
int nbits = stoi(ltrim(rtrim(nbits_temp)));
string representation;
getline(cin, representation);
vector> result = BinaryProduct(multiplicand, multiplier, nbits, representation);
for (int i = 0; i
for (int j = 0; j
fout
if (j != result[i].size() - 1) {
fout
}
}
if (i != result.size() - 1) {
fout
}
}
fout
fout.close();
return 0;
}
string ltrim(const string &str) {
string s(str);
s.erase(
s.begin(),
find_if(s.begin(), s.end(), not1(ptr_fun(isspace)))
);
return s;
}
string rtrim(const string &str) {
string s(str);
s.erase(
find_if(s.rbegin(), s.rend(), not1(ptr_fun(isspace))).base(),
s.end()
);
return s;
}
Language Python 3 Autocomplete Ready O c 1. Computer Arithmetic - Binary Multiplication # # Implement a function Binary Product(multiplicand, multiplier, nbits, representation) to calculate the product of unsigned 'nbits' integers given in the octal or hexadecimal representation using the following hardware. 11 16 # Multiplicand 64 bits 18 # 64-bit ALU 24 1 > #!/bin/python3... 10 11 # Complete the 'Binary Product' function below. 12 13 # The function is expected to return a 2D_STRING_ARRAY. 14 # The function accepts following parameters: 15 1. STRING multiplicand 2. STRING multiplier 17 3. INTEGER nbits 4. STRING representation 19 # 20 21 def Binary Product (multiplicand, multiplier, nbits, representation): 22 # Write your code here 23 vif __name__ == '__main__': fptr = open(os.environ['OUTPUT_PATH'], 'w') 25 26 multiplicand = input 27 28 multiplier = input() 29 30 nbits = int(input().strip) 31 32 representation = input() 33 34 result = Binary Product(multiplicand, multiplier, nbits, representation) 35 36 fptr.write(' '.join([' '.join(x) for x in result])) 37 fptr.write(' ') 38 39 fptr.close() 40 Product Shift right Write Control test 129 bits The function should return a nested list as a representation of the table shown below: 2x 3 (4-bits unsigned integers) Iteration Multiplicand Product 0 0010 0 0000 0011 1 0010 0 0001 0001 2 0010 0 0001 1000 3 0010 0 0000 1100 4 0010 0 0000 0110 Expected Output: 0 0010 000000011 1 0010 000010001 2 0010 000011000 3 0010 000001100 4 0010 000000110 Line: 23 Col: 27 Language Python 3 Autocomplete Ready O c 1. Computer Arithmetic - Binary Multiplication # # Implement a function Binary Product(multiplicand, multiplier, nbits, representation) to calculate the product of unsigned 'nbits' integers given in the octal or hexadecimal representation using the following hardware. 11 16 # Multiplicand 64 bits 18 # 64-bit ALU 24 1 > #!/bin/python3... 10 11 # Complete the 'Binary Product' function below. 12 13 # The function is expected to return a 2D_STRING_ARRAY. 14 # The function accepts following parameters: 15 1. STRING multiplicand 2. STRING multiplier 17 3. INTEGER nbits 4. STRING representation 19 # 20 21 def Binary Product (multiplicand, multiplier, nbits, representation): 22 # Write your code here 23 vif __name__ == '__main__': fptr = open(os.environ['OUTPUT_PATH'], 'w') 25 26 multiplicand = input 27 28 multiplier = input() 29 30 nbits = int(input().strip) 31 32 representation = input() 33 34 result = Binary Product(multiplicand, multiplier, nbits, representation) 35 36 fptr.write(' '.join([' '.join(x) for x in result])) 37 fptr.write(' ') 38 39 fptr.close() 40 Product Shift right Write Control test 129 bits The function should return a nested list as a representation of the table shown below: 2x 3 (4-bits unsigned integers) Iteration Multiplicand Product 0 0010 0 0000 0011 1 0010 0 0001 0001 2 0010 0 0001 1000 3 0010 0 0000 1100 4 0010 0 0000 0110 Expected Output: 0 0010 000000011 1 0010 000010001 2 0010 000011000 3 0010 000001100 4 0010 000000110 Line: 23 Col: 27Step by Step Solution
There are 3 Steps involved in it
Step: 1
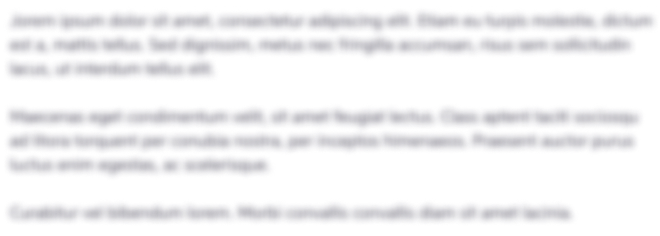
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started