Question
Can I get help with this please? No more info provided in lesson. The psuedocode (below in bold) is included after #4 Steps for completion
Can I get help with this please?
No more info provided in lesson.
The psuedocode (below in bold) is included after #4 "Steps for completion" .
Develop an algorithm to search and remove data from a hash table using the open addressing technique. Aim: Implement a hash table using open addressing with linear probing.
To solve this activity, you have to implement the following methods in the OpenAddrHashTable.java file:
public void put(K key, V value) { //... } private int searchPosition(K key) { //... } public void remove(K key) { //... } public Optional get(K key) { //... }
Steps for Completion
- Study the pseudocode shown in Snippet 3.3 and Snippet 3.4 (shown below) and implement them in Java.
- The container class OpenAddrPair will hold your key and value in the hash table.
- Have a flag on this container to indicate when an item is deleted.
- Use this flag in the put operation to overwrite it if it is deleted. You can use this flag to optimize your get method using the filter method.
insert(key, value, array) s = length(array) hashValue = hash(key, s) i = 0 while (i < s and array[(hashValue + i) mod s] != null) i = i + l if (i < s) array[(hashValue + i) mod s] = (key, value)
Snippet 3.3: Psuedocode for inserting using linear probing
search(key, array) s = length(array) hashValue = hash(key, s) i = 0 while (i < s and array[(hashValue + i) mod s] != null and array[(hashValue + i) mod s].key != key) i = i + 1 keyValue = array[(hashValue + i) mod s] if (keyValue != null && keyValue.key == key) return keyValue.value else return null
Snippet 3.4: Solution pseudocode for searching using linear probing
Tasks
New OpenAddrHashTable objects are empty.
1 Implement the following methods in the OpenAddrHashTable class:
- put
- searchPosition
- get
- remove
Code given:
import java.util.Optional;
public class OpenAddrHashTable implements HashTable { private final HashProvider hashProvider; private Pair[] array;
public OpenAddrHashTable(int capacity, HashProvider hashProvider) { array = new Pair[capacity]; this.hashProvider = hashProvider; }
public void put(K key, V value) { // write your code here }
private int searchPosition(K key) { // write your code here }
public void remove(K key) { // write your code here }
public Optional get(K key) { // write your code here return Optional.empty(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
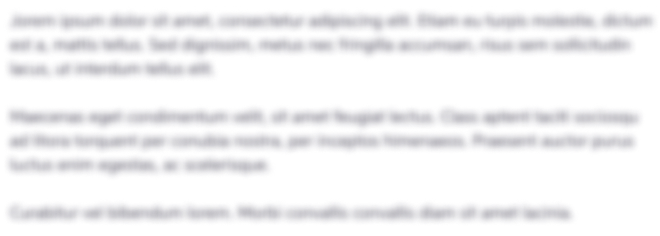
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started