Question
Can I get some help on this program? In this program you will explore arrays in C and how to use command-line parameters. You will
Can I get some help on this program?
In this program you will explore arrays in C and how to use command-line parameters. You will also write a C program(not C++) to implement a bubble sort algorithm on an array of integers, and use command-line parameters to populate an array with data. Make sure you read this page first about using command-line parameters:
Command-line arguments in C/C++Links to an external site.
You cannot simply "run" a program in repl.it and pass command-line parameters to the executable. To do so you need to use the "terminal" window (where you normally view output) on the right. You will need to "run" your program any time you make a change to make sure it compiles and generate it the executable, then you can use the terminal to run it with command-line arguments. Here's a sample repl which shows the number and values of all the command-line arguments, with output on the right. The first 3 lines (up to > ./main is the output after hitting "run"). I then ran the program again with 7 command-line parameters
./main this is a test 1 2 3
Which as you can see are available in the program as *argv. Note: argv[0] will always be the executable name. You must start accessing user-provided command-line parameters at argv[1].
https://replit.com/@gschulbe/C-Command-Line-Parameters
You can also fork the above replLinks to an external site. to play with this on your own or use it as a starting point for your program.
Assignment Description
In this assignment you will learn about C arrays and how to make your program interact with the Unix (i.e. Linux) command line. You will then write a simple sorting program using C.
Arrays in C
Arrays in C are declared as follows:
int foo[10]; //Declares an array called 'foo' with space for 10 ints.
and accessed as follows:
int i; i = foo[4]; /* This gets the element from 'foo' at index 4. */
Note that array elements are not initialized to be anything, so they should be assumed to hold garbage until you assign a value to them. Also, if you try to access an element "off the end" of the array (e.g. the 100th element of the ten-element array foo in the code above), you will get no compiler warnings, but you'll probably get a core dump when the program runs.
Arrays can also be two-dimensional, three-dimensional, etc. The syntax for a 2D array is:
int foo[10][5]; foo[4][2] = 999; //assign 999 to element 4,2
Arrays can be initialized when they are declared:
int foo[5] = { 1, 2, 3, 4, 5 }; int bar[2][3] = { { 1, 2, 3 }, { 4, 5, 6 } };
Finally, you can pass arrays to a function like you would pass a normal variable:
void munge(int array[]) { /* code that uses the values in array[] */ } int main(void) { int stuff[10]; /* Pass the 'stuff' array to the function 'munge'. */ munge(stuff); }
Note that you are not passing a copy of the array to the function, but instead you are passing the array itself. That means that if you modify the array in the function it will remain modified when you return from the function. The reason for this behavior is due to the fact that C arrays are actually represented as pointers. This is the same behavior as arrays in Java.
Requirements
Write a program which behaves as follows:
If there are no command-line arguments at all when the program is run, the program should print out instructions on its use (a "usage message". There should only be one usage message, and it must follow the standard conventions.
The program will accept an A or D as the second command line argument. This letter will tell you whether the bubble sort should sort in ascending or descending fashion.
The program will be able to accept up to 32 numbers (integers) on the command line.
If there are more than 32 numbers on the command line, or no numbers at all, the program should print out the usage message and exit with a non-zero value from main. This means 32 values not including the program name (argv[0]) and sort type.
You may assume that the command line arguments (except for the sort type) are all integers.
Sort the numbers using the bubble sort algorithm. Do not use a global array to hold the integers; use a locally-defined array in main and pass the array to the sorting function along with the type of sort (ascending or descending).
Print out the sorted numbers, one per line.
Your bubble sort function must be in its own separate file, along with any other functions that you create. The main() function should be in a file called main.c, and should contain ONLY the main() function.
This program must be written in C (not C++). Note that if you're compiling on an OS, use the gcc command, not g++. Note also that since your program is in C, your source files should have a .c extension, not .cpp or .cxx.
Notes and Hints
Your sorting functions should operate on an an array of integers, not characters. See the sample output below for how it should work. You will need to create an array of integers to store the values, and convert each value (after the sort order character) in argv to an integer and store it in the array. The easiest way to do this in C is with the atoiLinks to an external site.function, which is part of stdlib.h
... #include... ... = atoi(argv[i]); // inside of a loop
You won't be able to "run" your code in replit using the button, though you can use that button to compile your code. If it compiles correctly, run the program using the command-line shell in the right pane in replit. See the sample output below for examples.
Don't forget to comment your code create a header at the top. Reminder: the Programming Assignment Guidelinespage describes the guidelines for all programs.
Don't forget to put your bubble sort code in a separate file (use the "add file" button in the repl.it file pane). You will need to include a function prototype for the functions in main.c. See the Function Prototypes page for more details.
Feel free to create a separate header file for your functions in your repl.it project.
Sample Output
> ./main A 12 -88 4 19 1 42 -88 1 4 12 19 42 > ./main D 12 -88 4 19 1 42 42 19 12 4 1 -88 > ./main G 5 2 Incorrect usage Sort given data in ascending or descending order. First argument must be A or D to indicate ascending or descending sort order, followed by up to 32 integers separated by spaces Usage: ./main [A|D] n1 n2 ... n31 n32 > ./main A Incorrect usage Sort given data in ascending or descending order. First argument must be A or D to indicate ascending or descending sort order, followed by up to 32 integers separated by spaces Usage: ./main [A|D] n1 n2 ... n31 n32 > ./main A 3 4 5 1 2 3 4 5 6 3 3 4 5 6 6 4 5 6 6 7 9 4 3 54 5 4 3 4 5 5 5 5 5 6 7 2 3 4 5 6 6 7 Incorrect usage Sort given data in ascending or descending order. First argument must be A or D to indicate ascending or descending sort order, followed by up to 32 integers separated by spaces Usage: ./main [A|D] n1 n2 ... n31 n32
Step by Step Solution
There are 3 Steps involved in it
Step: 1
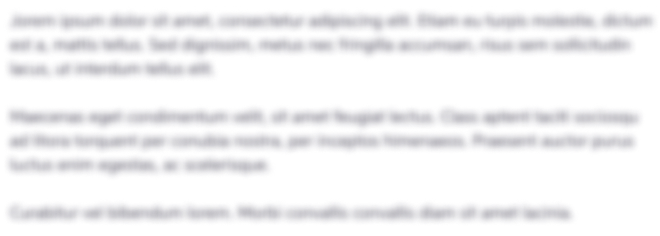
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started