Question
Can some write the JUnit tests to this code that will determine if each of the complex number operations are correct. The tests that should
Can some write the JUnit tests to this code that will determine if each of the complex number operations are correct. The tests that should be included are testAdd, testSubtract, testMult, testDiv, testEqComp (equals and compareTo together), testNorm, and testParse. These seven JUnit tests need to be able to test the methods of the ComplexNumber class. They should test a variety of different situations. Test more than one set of values for each of the methods - one test case is not usually enough to determine if it works correctly.
public class ComplexNumber {
// Variables represent the state of complex Numbers:
private final MyDouble real; // To be initialized in constructors
private final MyDouble imag; // To be initialized in constructors
// Constructors:
public ComplexNumber(MyDouble realIn, MyDouble imagIn){
real = realIn;
imag = imagIn;
}
public ComplexNumber(MyDouble realIn){
MyDouble zero = new MyDouble(0);
real = realIn;
imag = zero;
}
public ComplexNumber(ComplexNumber x){
real = x.real;
imag = x.imag;
}
//Instance methods
public MyDouble getReal(){
return real;
}
public MyDouble getImag(){
return imag;
}
public ComplexNumber add(ComplexNumber num1) {
MyDouble part1 = real.add(num1.getReal());
MyDouble part2 = imag.add(num1.getImag());
ComplexNumber result = new ComplexNumber(part1,part2);
return result;
}
public ComplexNumber subtract(ComplexNumber num1) {
MyDouble part1 = real.subtract(num1.getReal());
MyDouble part2 = real.subtract(num1.getImag());
ComplexNumber result = new ComplexNumber(part1, part2);
return result;
}
public ComplexNumber multiply(ComplexNumber num1) {
MyDouble negative = new MyDouble(-1);
MyDouble part1 = real.multiply(num1.getReal());
MyDouble part2 = imag.multiply(num1.getImag());
MyDouble part3 = real.multiply(num1.getImag());
MyDouble part4 = imag.multiply(num1.getReal());
part2=part2.multiply(negative);
MyDouble part5 = part1.add(part2);
MyDouble part6 = part3.add(part4);
ComplexNumber result = new ComplexNumber (part5,part6);
return result;
}
public ComplexNumber divide(ComplexNumber num1) {
MyDouble part1 = real.multiply(num1.real);
MyDouble part2 = imag.multiply(num1.imag);
MyDouble part3 = part1.add(part2);
MyDouble part4 = num1.real.multiply(num1.real);
MyDouble part5 = num1.imag.multiply(num1.imag);
MyDouble part6 = part4.add(part5);
MyDouble complex1 = part3.divide(part6);
MyDouble part8 = imag.multiply(num1.real);
MyDouble part9 = real.multiply(num1.imag);
MyDouble part10 = part8.subtract(part9);
MyDouble complex2 = part10.divide(part6);
ComplexNumber result = new ComplexNumber (complex1,complex2);
return result;
}
public boolean Equals(ComplexNumber otherNum) {
return real.equals(otherNum.real) && imag == otherNum.imag;
}
public int compareTo(ComplexNumber num1){
if(norm(this).compareTo(norm(num1))==0){
return 0;
}
else if(norm(this).compareTo(norm(num1))<0){
return -1;
}
else{
return 1;
}
}
public String toString() {
MyDouble zero = new MyDouble(0);
if(real.compareTo(zero) != 0){
if(imag.compareTo(zero) < 0){
return real.toString() + "-"+ imag.abs().toString()+"i";
} else if(imag.compareTo(zero) > 0){
return real.toString() + "+" + imag.toString()+"i";
} else{
return real.toString() + "+ 0i";
}
} else{
if(imag.compareTo(zero)<0){
return "0" + "-" + imag.abs().toString() + "i";
} else if(imag.compareTo(zero) > 0){
return "0" + "+" + imag.toString() + "i";
} else{
return "0";
}
}
}
// Public Static Methods:
public static MyDouble norm(ComplexNumber num1) {
MyDouble part1 = num1.real;
MyDouble part2 = num1.imag;
MyDouble part3 = part1.multiply(part1);
MyDouble part4 = part2.multiply(part2);
MyDouble part5 = part3.add(part4);
MyDouble norm = part5.sqrt();
return norm;
}
public static ComplexNumber parseComplexNumber(String string1) {
MyDouble zero = new MyDouble(0);
MyDouble zero1 = new MyDouble(0);
ComplexNumber parsed = new ComplexNumber(zero,zero1);
String string2 = string1;
string2.replaceAll(" ", "");
String string3 = string2;
if(string3.indexOf("+")>0){
int stop1 = string3.lastIndexOf("+");
String realString = string3.substring(0,stop1);
double realDouble = Double.parseDouble(realString);
MyDouble realMyDouble = new MyDouble(realDouble);
String imagString = string3.substring(stop1+1,string3.length()-1);
double imagDouble = Double.parseDouble(imagString);
MyDouble imagMyDouble = new MyDouble(imagDouble);
parsed = new ComplexNumber(realMyDouble,imagMyDouble);
}else{
int stop2 = string3.lastIndexOf("-");
String realString2 = string3.substring(0,stop2);
double realDouble2 = Double.parseDouble(realString2);
MyDouble realMyDouble2 = new MyDouble(realDouble2);
String imagString2 = string3.substring(stop2+1,string3.length()-1);
double imagDouble2 = Double.parseDouble(imagString2);
MyDouble imagMyDouble2 = new MyDouble(imagDouble2);
parsed = new ComplexNumber(realMyDouble2,imagMyDouble2);
}
return parsed;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
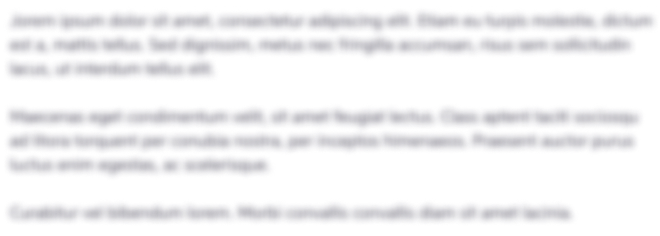
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started