Question
Can somebody help me revise main c please? Task 1: Play the buzzer . Turn on and off the buzzer sound to make the alarm
Can somebody help me revise main c please?
Task 1: Play the buzzer. Turn on and off the buzzer sound to make the alarm sound.
Task 2: Check the pushbuttons. Check the pushbuttons every 10 ms for user inputs; also implements debouncing.
Task 3: Check the motion sensor. Check the motion every periodically to detect human motion.
For Tasks 2 and 3, we have used a programming technique, called I/O polling, to poll the status of the push buttons and the PIR motion sensor (although the term itself was not used). While this technique works, it prevents the microcontroller from sleeping. If we use a battery as the power source of the system, the battery life will be negatively affected. The difference can be very large, because a microcontroller consumes much less power when it sleeps.
To save energy, we will switch to another programming technique, called interruptdriven I/O, to implement Tasks 2 and 3.
Part 0:
Attach the LaunchPad with the Grove base boosterpack, attach the buzzer to jumper J16, and attach the PIR motion sensor to jumper J17, as you have done in Lab 4. Double check that you have used the correct jumpers.
Note the provided main.c does not use your driver functions for the PIR motion sensor, but your final solution code should.
Build and debug the program. The program will behave as follows. It is exactly as the starter code in Lab 4, but the code is different.
Push the SW1 button, the buzzer will start beeping. (It buzzes for 30 milliseconds, idles for 2,970 milliseconds, and then repeating.)
Push the SW2 button, the buzzer will stop beeping.
Note: The buzzer sound can be disturbing in a quite environment. Be mindful of people around you.
In this lab, you may need to use this debugging technique to help debug your program.
Part 1: Interrupts from Motion Detection
First, read through the provided main.c and understand the function pbIntrHandler() and another function setInterrupts(). You will notice that Task 2, checking the pushbuttons, is now implemented as the interrupt handler. The general idea is to enable interrupt on the I/O port and pins used by SW1 and SW2 (Port C, pins 0 and 4). When a user pushes SW1 or SW2, an interrupt signal will be sent to the microcontroller. The pushbutton interrupt handler is executed to check which pushbutton has been pushed, and then it activates or de-activates the buzzer system.
Note that in the while-loop in the main() function, the program executes an WFI (wait for interrupt) assembly instruction. This instruction makes the microcontroller enter a sleep mode and stay in the mode until an interrupt happens. Read ARMv7-M Architecture Reference Manual, page A7-561, for a detailed explanation of the instruction.
Revise main.c such that the microcontroller will also wake up on motion detection. You will need to 1) enable interrupt on the I/O port and pin used by the motion sensor, and 2) add another interrupt handler for the motion sensor. In addition to the functionalities of the starter code, your system should work as follows:
If any human motion is detected, the program should turn on the on-board LED in red color.
If there is no human motion, the program should turn on the on-board LED in green color.
In this part, the motion sensor does not activate or de-activate the buzzer system. You will add that in the next part.
The purpose of this part is to make sure the interrupt from the motion sensor works.
Demo your system to your TA. Then, show your source code to your TA and explain how it works. Each of you may have to answer questions from your TA to test your understanding of the working details. Your answer may affect your lab grade.
Part 2: Motion Detection Alarm by Interrupts
Revise the code in main.c such that your system works as follows (exactly as in Lab 4):
If the user pushes the SW1 button, the system should be activated. If the user pushes the SW2 button, the system will be de-activated. (Similar to Part 0.)
When the system is activated and no human motion is detected, the on-board LED should be turned on and stay in the green color.
When the system is activated and human motion is detected, the buzzer should beep (as in Part 0) and the on-board LED should change to and stay in the red color.
When the system is de-activated, the on-board LED should be turned off, and the buzzer should make no sound.
Note: Like the pushbuttons, the input from the motion sensor may not be stable (i.e. has the bouncing effect). For this lab, it is OK if the bouncing effect shows up in the demo.
Important: Use the C volatile qualifier in the declaration of any global variables whose value will be changed by an interrupt handler. Otherwise, your non-ISR code may not detect those changes. Example:
volatile bool userActivated;
Demo your system to your TA. Then, show your source code to your TA and explain how it works. Each of you may have to answer questions from your TA to test your understanding of the working details. Your answer may affect your lab grade.
Here is main.C
/* * main.c: ECE 266 Lab 5, Fall 2018, Motion Detection Alarm (interrupt-based) */ #include#include #include #include #include #include #include #include #include #include #include "launchpad.h" #include "buzzer.h" #include "motion.h" // Buzzer-related constants #define BUZZER_CHECK_INTERVAL 30 #define BUZZER_ON_TIME 30 #define BUZZER_OFF_TIME (3000 - BUZZER_ON_TIME) /* * The task for playing the buzzer. * * The buzzer state and callback function. The buzzer system can be in four states: * Off: The system is turned off. The buzzer is silent. * On: The buzzer system is turned on. The buzzer will buzz periodically. * SwitchOn: The buzzer system is to be turned on. * SwitchOff: The buzzer system is to be turned off. */ // The buzzer object typedef struct { enum { Off, On, SwitchOff, SwitchOn } state; // the running state of the buzzer system bool buzzing; // if the buzzer is buzzing int32_t timeLeft; // the time left for the buzzer to buzz or not buzz } buzzer_t; static volatile buzzer_t buzzer = { Off, false, 0 }; // The last motion detected time static volatile uint32_t lastMotionDetectedTime = 0; // The buzzer play callback function void buzzerPlay(uint32_t time) { uint32_t delay = BUZZER_CHECK_INTERVAL; // the delay for next callback switch (buzzer.state) { case Off: // the buzzer system is turned off break; case On: // the buzzer system is active, turn buzzer on and off if (buzzer.buzzing) { // If the buzzer has been buzzing for enough time, turn it off if ((buzzer.timeLeft -= BUZZER_CHECK_INTERVAL) <= 0) { buzzerOff(); buzzer.buzzing = false; buzzer.timeLeft = BUZZER_OFF_TIME; } } else { // If the buzzer has been silent for enough time, turn it on if ((buzzer.timeLeft -= BUZZER_CHECK_INTERVAL) <= 0) { buzzerOn(); buzzer.buzzing = true; buzzer.timeLeft = BUZZER_ON_TIME; } } break; case SwitchOff: // De-activate the buzzer system if (buzzer.buzzing) buzzerOff(); buzzer.state = Off; buzzer.buzzing = Off; break; case SwitchOn: // Activate the buzzer system buzzerOn(); buzzer.state = On; buzzer.buzzing = true; buzzer.timeLeft = BUZZER_ON_TIME; break; } // schedule the next callback schdCallback(buzzerPlay, time + delay); } /* * The task for checking push button, implemented as interrupt handler */ // If the user has activated the buzzer system or not static volatile bool userActivated = false; // The interrupt handler for checking the pushbuttons void pbIntrHandler() { // Last time pushbutton was pushed static uint32_t lastTime = 0; // IMPORTANT: Clear interrupt, otherwise the interrupt handler will be executed forever GPIOIntClear(GPIO_PORTF_BASE, GPIO_PIN_0 | GPIO_PIN_4); // Read the pushbutton int code = pbRead(); // Get the current system time uint32_t time = sysTimeGet(); // De-bouncing: If a key was pushed within 150 ms, ignore this push if (time < lastTime + 150) return; // Process the pushbutton input switch (code) { case 1: // SW1: Turn on the buzzer system userActivated = true; buzzer.state = SwitchOn; break; case 2: // SW2: Turn off the buzzer system userActivated = false; buzzer.state = SwitchOff; break; } // record the time to check for de-bouncing next time lastTime = time; } /* * Select a set of interrupts that can wake up the LaunchPad */ void setInterrupts() { // Set interrupt on Port F, pin 0 (SW1) and pin 4 (SW2) GPIOIntRegister(GPIO_PORTF_BASE, pbIntrHandler); // register the interrupt handler GPIOIntTypeSet(GPIO_PORTF_BASE, GPIO_PIN_0 | GPIO_PIN_4, // interrupt on falling edge, note that SW1 and SW2 are active low GPIO_FALLING_EDGE); IntPrioritySet(INT_GPIOF, 0); // set interrupt level to 0 (0 is the highest for programmable interrupts) GPIOIntEnable(GPIO_PORTF_BASE, GPIO_PIN_0 | GPIO_PIN_4); // enable interrupts on SW1 and SW2 input } /* * The main function */ int main(void) { lpInit(); buzzerInit(); pirInit(); setInterrupts(); // Print out a start message uprintf("%s ", "Lab 5 starts"); // Schedule the first callback events schdCallback(buzzerPlay, 200); // Run the callback scheduler while (true) { schdExecute(); // Put Tiva C into sleep until the next interrupt happens __asm(" wfi"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
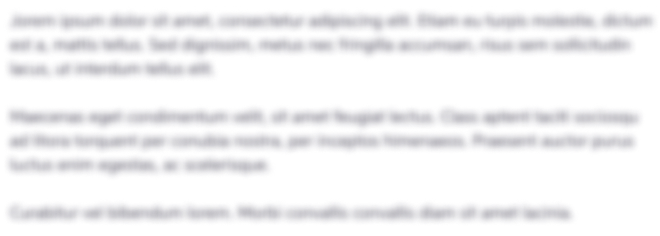
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started