Question
can someone fix the errors in this code? the name needs to be FractionDemo.java //author: Jazmine Tapia //date: 20 February 2023 //file: FractionDemo.java /* Lab
can someone fix the errors in this code? the name needs to be FractionDemo.java
//author: Jazmine Tapia //date: 20 February 2023 //file: FractionDemo.java
/* Lab 13 will also be using demonstation the the multiplication of two factors. */
//import statements for Scanner class import java.util.Scanner;
// Define a public class called Fraction public class FractionDemo {
// Define private instance variables for the numerator and denominator private int numerator; private int denominator;
// Define a constructor for the Fraction class that takes a numerator and denominator as arguments public FractionDemo(int numerator, int denominator) { // Set the denominator using the setDenominator() method defined below setDenominator(denominator); // Set the numerator this.numerator = numerator; // Reduce the fraction reduce(); // Adjust the signs of the numerator and denominator if necessary adjustSigns(); }
// Define a getter method for the numerator public int getNumerator() { return numerator; }
// Define a setter method for the numerator public void setNumerator(int numerator) { this.numerator = numerator; }
// Define a getter method for the denominator public int getDenominator() { return denominator; }
// Define a setter method for the denominator public void setDenominator(int denominator) { // Set the denominator using the setDenominator() method defined below setDenominator(denominator); // Reduce the fraction reduce(); // Adjust the signs of the numerator and denominator if necessary adjustSigns(); }
// Define a private method to set the denominator private void setDenominator(int denominator) { // If the denominator is zero, throw an exception if (denominator == 0) { throw new IllegalArgumentException("Denominator cannot be zero"); } // Set the denominator this.denominator = denominator; }
// Define a private method to calculate the greatest common divisor of two integers private int gcd(int a, int b) { if (b == 0) { return a; } else { return gcd(b, a % b); } }
// Define a public method to reduce the fraction public void reduce() { // Calculate the greatest common divisor of the numerator and denominator int gcd = gcd(numerator, denominator); // Divide both the numerator and denominator by the greatest common divisor to reduce the fraction numerator /= gcd; denominator /= gcd; }
// Define a private method to adjust the signs of the numerator and denominator if necessary private void adjustSigns() { // If the denominator is negative, multiply both the numerator and denominator by -1 to adjust the signs if (denominator < 0) { numerator *= -1; denominator *= -1; } }
// Define a public method to add two fractions public FractionDemo add(FractionDemo other) { public static void main(String[] args) { Scanner input = new Scanner(System.in);
// Get the numerator and denominator for the first fraction System.out.print("Enter the numerator of the first fraction: "); int num1 = input.nextInt(); System.out.print("Enter the denominator of the first fraction: "); int den1 = input.nextInt();
// Get the numerator and denominator for the second fraction System.out.print("Enter the numerator of the second fraction: "); int num2 = input.nextInt(); System.out.print("Enter the denominator of the second fraction: "); int den2 = input.nextInt();
// Create the two Fraction objects Fraction f1 = new Fraction(num1, den1); Fraction f2 = new Fraction(num2, den2);
// Display the fractions System.out.println("f1==" + f1); System.out.println("f2==" + f2);
// Perform arithmetic operations and display the results System.out.println(f1 + " + " + f2 + " = " + f1.add(f2)); System.out.println(f1 + " - " + f2 + " = " + f1.subtract(f2)); System.out.println(f1 + " * " + f2 + " = " + f1.multiply(f2)); System.out.println(f1 + " / " + f2 + " = " + f1.divide(f2)); } FractionDemo.java:110: error: illegal start of expression public static void main(String[] args) ^ FractionDemo.java:139: error: reached end of file while parsing } ^ 2 errors
The code has to be a combination of these two codes
//import statements for Scanner class import java.util.Scanner;
public class FractionDemo { public static void main(String[] args) { // Variables for two fractions int num1, num2, den1, den2;
// Create Scanner object to read keyboard input Scanner keyboard = new Scanner(System.in);
// Get integers for f1 numerator and denominator System.out.print("Enter the numerator of the first fraction: "); num1 = keyboard.nextInt(); System.out.print("Enter the denominator of the first fraction: "); den1 = keyboard.nextInt();
// Get integers for f2 numerator and denominator System.out.print("Enter the numerator of the second fraction: "); num2 = keyboard.nextInt(); System.out.print("Enter the denominator of the second fraction: "); den2 = keyboard.nextInt();
// Instantiate the two fractions using the input values Fraction f1 = new Fraction(num1, den1); Fraction f2 = new Fraction(num2, den2);
// Display the two fractions and their product System.out.println("f1 == " + f1); System.out.println("f2 == " + f2); System.out.println(f1 + " * " + f2 + " == " + f1.multiply(f2)); } }
// Define a class for fractions class Fraction { private int numerator; private int denominator;
// Constructor for Fraction class public Fraction(int num, int den) { this.numerator = num; this.denominator = den; // Check if denominator is zero and set it to 1 if true if (den == 0) { System.out.println("For Fraction: ( " + num + " / " + den + " ):"); System.out.println("Denominator cannot be zero; denominator value is being reset to one."); this.denominator = 1; // Reset denominator to 1 System.out.println("The Fraction is now: " + this); } }
// Define a method for multiplying two fractions public Fraction multiply(Fraction other) { int num = this.numerator * other.numerator; int den = this.denominator * other.denominator; return new Fraction(num, den); }
// Override the toString() method to display the Fraction objects as strings public String toString() { if (denominator == 1) return " ( " + numerator + " ) "; else return " ( " + numerator + " / " + denominator + " ) "; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
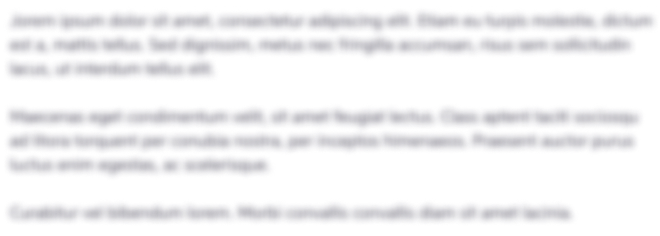
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started