Question
Can someone help me solve this Exception error I am getting? I cannot find out why this is happening: OUTPUT: Team 1: Team Name: Giants
Can someone help me solve this Exception error I am getting? I cannot find out why this is happening:
OUTPUT:
Team 1: Team Name: Giants Owner: Bob
Team 2: Team Name: Cougars Owner: Nate
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1 at FantasyFootballTester.main(FantasyFootballTester.java:35)
FantasyFootballTester.java
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class FantasyFootballTester { public static void main(String[] args) { //String name, String post, String team, int games, int passAtt, int passComp, int touchPass, int totalYardsPass FantasyFootballTeam fft1 = new FantasyFootballTeam("Giants", "Bob", 10); FantasyFootballTeam fft2 = new FantasyFootballTeam("Cougars", "Nate", 10); QuarterBack qb1 = null; QuarterBack qb2 = null; RunningBack rb1 = null; RunningBack rb2 = null; DefensiveBack db1 = null; DefensiveBack db2 = null; System.out.println("Team 1:"); System.out.println(fft1); System.out.println("Team 2:"); System.out.println(fft2); File footballFile = new File("input.txt"); try { Scanner scanner = new Scanner(footballFile); while(scanner.hasNextLine()){ String Line = scanner.nextLine(); String contents[] = Line.split(", "); String name = contents[0]; String post = contents[1]; //ERROR String team = contents[2]; int games = Integer.parseInt(contents[3]); if(post.equals("Quarter Back")){ int passAtt = Integer.parseInt(contents[4]); int passComp = Integer.parseInt(contents[5]); int touchPass = Integer.parseInt(contents[6]); int td = Integer.parseInt(contents[7]); //puts it in first then second team if(qb1 == null){ qb1 = new QuarterBack(); qb1.setPName(name); qb1.setPost(post); qb1.setTeam(team); qb1.setGames(games); qb1.setPassAtt(passAtt); qb1.setPassComp(passComp); qb1.setTouchPass(td); qb1.setTotalPass(touchPass); }else{ qb2 = new QuarterBack(); qb2.setPName(name); qb2.setPost(post); qb2.setTeam(team); qb2.setGames(games); qb2.setPassAtt(passAtt); qb2.setPassComp(passComp); qb2.setTouchPass(td); qb2.setTotalPass(touchPass); } }else if(post.equals("Running Back")) { int runningAttempts = Integer.parseInt(contents[4]); int totalRunning = Integer.parseInt(contents[5]); int touchDowns = Integer.parseInt(contents[6]); //puts it in first then second team if(rb1 == null){ rb1= new RunningBack(); rb1.setPName(name); rb1.setPost(post); rb1.setTeam(team); rb1.setGames(games); rb1.setRunAtt(runningAttempts); rb1.setTotalRun(totalRunning); rb1.setTouchDown(touchDowns); }else{ rb2 = new RunningBack(); rb2.setPName(name); rb2.setPost(post); rb2.setTeam(team); rb2.setGames(games); rb2.setRunAtt(runningAttempts); rb2.setTotalRun(totalRunning); rb2.setTouchDown(touchDowns); } }else if(post.equals("Defensive Back")){ int tackles = Integer.parseInt(contents[4]); int interceptions = Integer.parseInt(contents[5]); int forcedFumbles = Integer.parseInt(contents[6]); //puts it in first then second team if(db1 == null){ db1 = new DefensiveBack(); db1.setPName(name); db1.setPost(post); db1.setTeam(team); db1.setGames(games); db1.setTackles(tackles); db1.setInterceptions(interceptions); db1.setForcedFumbles(forcedFumbles); }else{ db2 = new DefensiveBack(); db2.setPName(name); db2.setPost(post); db2.setTeam(team); db2.setGames(games); db2.setTackles(tackles); db2.setInterceptions(interceptions); db2.setForcedFumbles(forcedFumbles); } } } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } //adds each player to teams fft1.addPlayer(qb1); fft1.addPlayer(rb1); fft1.addPlayer(db1); fft2.addPlayer(qb2); fft2.addPlayer(rb2); fft2.addPlayer(db2); System.out.println(" "); //prints players info System.out.println(qb1); System.out.println(qb2); System.out.println(rb1); System.out.println(rb2); System.out.println(db1); System.out.println(db2); System.out.println(" "); //test System.out.println("Testing Finding player by position "+fft1.findPlayerbyPosition("Quarter Back")); //System.out.println(" Testing comparing two players rating"); } }
QuarterBack.java
public class QuarterBack extends FootballPlayer{ //Stores statistics specific to Quarterbacks private int passAtt; private int passComp; private int touchPass; private int totalYardsPass; public QuarterBack(){ super(); passAtt = 0; passComp = 0; touchPass = 0; totalYardsPass = 0; } public QuarterBack(String name, String post, String team, int games, int passAtt, int passComp, int touchPass, int totalYardsPass){ super(name, post, team, games); this.passAtt = passAtt; this.passComp = passComp; this.touchPass = touchPass; this.totalYardsPass = totalYardsPass; } public int getPassAtt(){ return passAtt; } public void setPassAtt(int passAtt){ this.passAtt = passAtt; } public int getPassComp(){ return passComp; } public void setPassComp(int passComp){ this.passComp = passComp; } public int getTouchPass(){ return touchPass; } public void setTouchPass(int touchPass){ this.touchPass = touchPass; } public int getTotalPass(){ return touchPass; } public void setTotalPass(int totalYardsPass){ this.totalYardsPass = totalYardsPass; } //calc methods public double completionPercentage(){ return passComp/passAtt; //Formula: passes completed / pass Attempts } public double averagePassingYardsPerGame(){ return totalYardsPass/this.games; //Formula: total yards passing/games Played } public double averageTouchDownsPerGame(){ return touchPass/this.games; //Formula: touch Downs Passing/games Played } @Override public int compareTo(int rating) { return playerRating() - rating; } @Override public int playerRating(){ int rating = (int) (this.averageTouchDownsPerGame()+(this.completionPercentage()*100)+(this.averagePassingYardsPerGame()/5)); return rating; } @Override public String toString(){ //returns a String representation of the object return super.toString()+ "Completion Percentage: "+completionPercentage()+ " Average Passing Yards Per Game: "+averagePassingYardsPerGame()+ " Average Touch Downs Per Game: "+averageTouchDownsPerGame()+ " Player's Rating: "+this.playerRating() + " "; } }
input.txt
Bobby Greg,Quarter Back,Giants,10,39,10,15,200 Tanner Morn,Running Back,Gators,17,67,55,6 Ricky Garret,Defensive Back,Stallions,15,20,8,18
Step by Step Solution
3.35 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
The error javalangArrayIndexOutOfBoundsException Index 1 out of bounds for length 1 occurs when you ...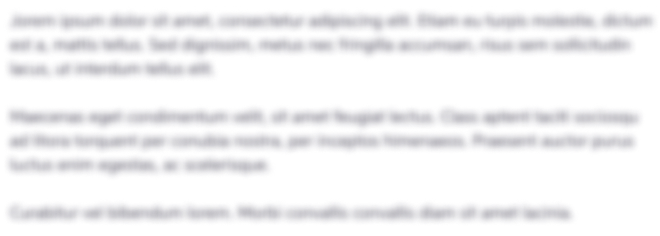
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started