Question
Can someone help me with this in C# and not in Java? I am needing the UML and the code in C#. Here is what
Can someone help me with this in C# and not in Java? I am needing the UML and the code in C#. Here is what I have so far. Can someone help me?
Create OO classes using an object-oriented programming language that contains inherited and polymorphic members from a UML diagram
Create abstracted classes and members using an object-oriented programming language based on a UML diagram
Implement inheritance and polymorphism using an object-oriented programming language based on a UML diagram
To conclude the project, use the UML diagram you created last week and create an application in Visual Studio named School. Once you have written the code, be sure and test it to ensure it works before submitting it. Below is the UML diagram for the basic class we created last week for your reference, but for this project be sure you use the one that you created last week.
Person |
-firstName : String -lastName : String -gender : Char -dateOfBirth : String -idNumber : string |
+Person() +setFirstName(in aFirstName : string) : void +setLastName(in aLastName : string) : void +setGender(in aGender : char) : void +setDateOfBirth(in aDateOfBirth : string) : void +setIdNumber(in aIdNumber : string) : void +GetFirstName() : string +GetLasstName() : string +GetGender() : char +GetDateOfBirth() : string +GetIdNumber() : string +CreateEmailAddress() : void +Display():string |
Student |
-rollNo : String -class : String -division : Char |
+Student() +setRollNo(in aRollNo : string) : void +setClass(in aClass: string) : void +setDivision(in aDivision : char) : void +GetRollNo() : string +GetClass() : string +GetDivision() : char |
Staff |
-designation : String -departmentName : String -salary : integer -perquisiteApplicable : Boolean |
+Staff() +setDesignation(in aDesignation : string) : void +setDepartmentName(in aDepartmentName: string) : void +setSalary(in aSalary : integer) : void +setPerquisiteApplicable(in aPerquisiteApplicable : bool) : void +GetDesignation() : string +GetDepartName() : string +GetSalary() : integer +GetPerquisiteApplicable() : Boolean |
Faculty |
-category : String -departmentName : String -classTeacher : String -subject : array[] -salary : integer -joingDate : Date |
+Faculty() +setCategory(in aCategory : string) : void +setDepartmentName(in aDepartmentName: string) : void +setClassTeacher(in aSalary : String) : void +setSubject(in aSubject : Array[]) : void +setJoiningDate(in aJoiningDate : Date) : Void +GetCategory() : string +GetDepartName() : string +GetSalary() : integer +GetClassTeacher() : string +GetJoiningDate(): Date |
using System;
using static System.Console;
namespace ConsoleApp1
{
class Person
{
public Person ()
{
}
string firstName;
string lastName;
char gender;
string dateOfBirth;
string idNumber;
public void SetfirstName(string aFirstName)
{
this.firstName = aFirstName;
}
public void SetLastName( string aLastName)
{
this.lastName = aLastName;
}
public void SetGender(char aGender)
{
this.gender = aGender;
}
public void SetDateOfBirth(string aDateOfBirth)
{
this.dateOfBirth = aDateOfBirth;
}
public void SetIdNumber(string aIdNumber)
{
this.idNumber = aIdNumber;
}
public string GetFirstName()
{
return this.firstName;
}
public string GetLastName()
{
return this.lastName;
}
public char GetGender()
{
return this.gender;
}
public string GetDateOfBirth()
{
return this.dateOfBirth;
}
public string GetIdNumber()
{
return this.idNumber;
}
public void CreateEmailAddress()
{
}
public string Display()
{
return ("FirstName: " + firstName + ", LastName: "
+ lastName + ", Gender: " + gender + ", DateOfBirth: " +
dateOfBirth + ", IdNumber: " + idNumber);
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace ConsoleApp1
{
class Student:Person
{
//class constructor
public Student()
{
}
// variable declaration
string rollNo;
// here i am using classes instead of class because
// it is same as class keywod in c# so it will conflict
string classes;
char division;
//setter method
public void SetRollNo(string aRollNo)
{
this.rollNo = aRollNo;
}
public void SetClasses(string aClasses)
{
this.classes = aClasses;
}
public void SetDivision(char aDivision)
{
this.division = aDivision;
}
//getter method
public string GetRollNo()
{
return this.rollNo;
}
public string GetClasses()
{
return this.classes;
}
public char GetDivision()
{
return this.division;
}
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace ConsoleApp1
{
class Staff:Person
{
string designation;
string departmentName;
int salary;
Boolean perquisiteApplicable;
//setter method
public void SetDesignation(string aDesignation)
{
this.designation = aDesignation;
}
public void SetDepartmentName(string aDepartmentName)
{
this.departmentName = aDepartmentName;
}
public void SetSalary(int aSalary)
{
this.salary = aSalary;
}
public void SetPerquisiteApplicable(bool aPerquisiteApplicable)
{
this.perquisiteApplicable = aPerquisiteApplicable;
}
//getter method
public string GetDesignation()
{ return this.designation; }
public string GetDepartName()
{ return this.departmentName; }
public int GetSalary()
{ return this.salary; }
public Boolean GetPerquisiteApplicable()
{ return this.perquisiteApplicable; }
}
}
using System;
using System.Collections.Generic;
using System.Text;
using static System.Console;
namespace ConsoleApp1
{
class Faculty:Person
{
//class constructor
public Faculty()
{
}
//variable declaration
string category;
string departmentName;
string classTeacher;
Array[] subject;
int salary;
// in c# DateTime available not only Date
DateTime joiningDate;
//setter method
public void SetCategory(string aCategory)
{
this.category = aCategory;
}
public void SetDepartmentName(string aDepartmentName)
{
this.departmentName = aDepartmentName;
}
public void SetClassTeacher(string aClassTeacher)
{
this.classTeacher = aClassTeacher;
}
public void SetSalary(int aSalary)
{
this.salary = aSalary;
}
public void SetSubject(Array[] aSubject)
{
this.subject = aSubject;
}
public void SetJoiningDate(DateTime aJoiningDate)
{
this.joiningDate = aJoiningDate;
}
//getter method
public string GetCategory()
{
return this.category;
}
public string GetDepartmentName()
{
return this.departmentName;
}
public string GetClassTeacher()
{
return this.classTeacher;
}
public int GetSalary()
{
return this.salary;
}
public DateTime GetJoiningDate()
{
return this.joiningDate;
}
public Array[] GetSubject()
{
return this.subject;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
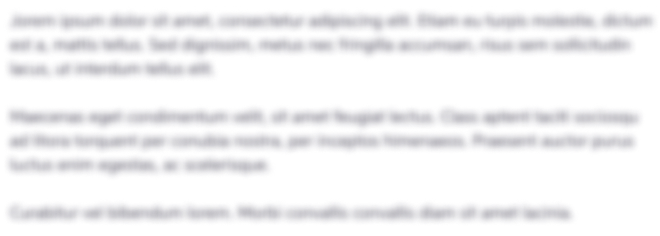
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started