Question
/* Cart * Anderson, Franceschi */ import javax.swing.JFrame; import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat; public class Cart { Item[] items; int itemSize = 3; int
/* Cart * Anderson, Franceschi */ import javax.swing.JFrame; import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat;
public class Cart { Item[] items; int itemSize = 3; int currentItem;
int currentNumberItems; int totalNumberItems; double currentTotal; double exactTotal;
Color background = new Color( 205, 205, 205 );
public Cart( ) { items = new Item[itemSize];
// // Student can modify constructors' arguments below // Check out the 3 constructors // argument represents price // items[0] = new Milk( 2.00 ); items[1] = new Cereal( 3.50 ); items[2] = new OrangeJuice( 3.00 ); // // currentNumberItems = 0; totalNumberItems = 0; currentTotal = 0.0; exactTotal = 0.0; currentItem = -1; }
public void setCurrentItem( int ci ) { currentItem = ci; }
public int getCurrentItem( ) { return currentItem; }
public Item [] getItems( ) { return items; }
public int getItemSize( ) { return itemSize; }
public void updateTotal( double newCurrentTotal ) { currentTotal = newCurrentTotal; }
public void updateNumberItems( ) { currentNumberItems++; }
public int getNumberItems( ) { return currentNumberItems; }
public void updateTotalNumberItems( int newTotalNumberItems ) { totalNumberItems = newTotalNumberItems; }
public int getTotalNumberItems( ) { return totalNumberItems; }
public void setExactTotal( double newExactTotal ) { exactTotal = newExactTotal; }
public Color getBackground( ) { return background; }
public void draw( Graphics g ) { g.setColor( Color.black ); g.drawString( "EXPRESS LANE", 125, 50 ); g.setColor( Color.black ); g.fillRoundRect( 50, 200, 150, 10, 2, 2 ); // belt g.setColor( new Color( 220, 110, 55 ) ); g.fill3DRect( 195, 200, 60, 70, true ); // bag
DecimalFormat money = new DecimalFormat( "$0.00" );
String displayItemNumber = "Item # " + currentNumberItems + " of " + totalNumberItems; String displayStudentTotal = "Your subtotal = " + money.format( currentTotal ); String displayExactTotal = "Correct subtotal = " + money.format( exactTotal );
g.setColor( Color.blue );
g.drawString( displayItemNumber, 220, 80 ); g.drawString( displayStudentTotal, 20, 90 ); g.drawString( displayExactTotal, 20, 115 );
if ( currentItem != -1 ) items[currentItem].draw( g, 50, 200, 200, background ); } }
/* Cashier class Anderson, Franceschi */
import java.awt.Color; import java.awt.Graphics; import javax.swing.JFrame; import javax.swing.JOptionPane; import java.text.DecimalFormat;
public class Cashier extends JFrame { private Cart cart; private Item previousItem; private double currentTotal;
public Cashier() { super("Chapter 6 Programming Activity 2"); cart = new Cart(); previousItem = null; currentTotal = 0.0; getContentPane().setBackground(cart.getBackground()); setSize(325, 300); setVisible(true); }
public void checkout(int numberOfItems) { /* ***** Student writes the body of this method ***** */ // // The parameter of this method, numberOfItems, // represents the number of items in the cart. The // user will be prompted for this number. // // Using a for loop, calculate the total price // of the groceries for the cart. // // The getNext method (in this Cashier class) returns the next // item in the cart, which is an Item object (we do not // know which item will be returned; this is randomly generated). // getNext does not take any arguments. its API is // Item getNext() // // As the last statement of the body of your for loop, // you should call the animate method. // The animate method takes one parameter: a double, // which is your current subtotal. // For example, if the name of your variable representing // the current subtotal is total, your call to the animate // method should be: // animate(total); // // The getPrice method of the Item class // returns the price of the Item object as a double. // The getPrice method does not take any arguments. Its API is // double getPrice() // // After you have processed all the items, display the total // for the cart in a dialog box. // Student code starts here:
// Student code ends here. }
public Item getNext() { if (cart.getTotalNumberItems() > cart.getNumberItems()) { // get next item cart.setCurrentItem ((int) (Math.random() * cart.getItemSize()));
// update previousItem so that we can keep track of the current total previousItem = cart.getItems()[cart.getCurrentItem()];
// update number of items in cart cart.updateNumberItems();
// update currentTotal if ((previousItem != null) && (previousItem.getPrice() >= 0)) currentTotal += previousItem.getPrice(); cart.setExactTotal(currentTotal);
return (cart.getItems())[cart.getCurrentItem()]; } else { JOptionPane.showMessageDialog(null, "Error: getNext() method called when cart is empty", "Logic error", JOptionPane.ERROR_MESSAGE); return null; } }
public void animate(double subtotal) { cart.updateTotal(subtotal);
repaint(); try { Thread.sleep(3000); // wait for the animation to finish } catch (Exception e) { } }
public void paint(Graphics g) { super.paint(g); cart.draw(g); }
public static void main(String [] args) { Cashier app = new Cashier(); app.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); int numItems = 0; boolean goodInput = false; do { String howMany = JOptionPane.showInputDialog(null, "Enter the number of items in the cart (1 - 10)"); if (howMany == null) System.exit(0); try { numItems = Integer.parseInt(howMany); goodInput = true; } catch(NumberFormatException nfe) { // goodInput is still false } } while (!goodInput || numItems < 1 || numItems > 10);
(app.cart).updateTotalNumberItems(numItems); app.checkout(numItems); }
}
/* Cereal * Anderson, Franceschi */ import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat;
public class Cereal extends Item { public Cereal( double p ) { super( p ); }
public void draw( Graphics g, int startX, int endX, int y, Color eraseColor ) { DecimalFormat money = new DecimalFormat( "$0.00" );
String display1 = "Cereal: Unit price = " + money.format( price );
g.setColor( Color.BLUE ); g.drawString( display1, 20, 65 );
for ( int x = startX; x < endX; x += 5 ) { g.setColor( new Color( 255, 255, 51 ) ); g.fillRect( x, y - 68, 48, 65 ); g.setColor( Color.BLACK ); g.drawString("Cereal", x + 5, y - 45 ); g.setColor( Color.RED ); g.drawOval( x + 18, y - 35, 8, 8 ); g.drawOval( x + 13, y - 30, 8, 8 ); g.drawOval( x + 23, y - 30, 8, 8 ); g.fillArc( x + 10, y - 35, 24, 25, 0, -180 ); try { Thread.sleep( ( int )( 100 ) ); } catch ( InterruptedException e ) { e.printStackTrace( ); } // erase g.setColor( eraseColor ); // background g.fillRect( x, y - 70, 50, 70 ); } } }
/** Divider * Anderson, Franceschi */
import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat;
public class Divider extends Item { public Divider( double p ) { super( p ); }
public void draw( Graphics g, int startX, int endX, int y, Color eraseColor ) {
DecimalFormat money = new DecimalFormat( "$0.00");
for ( int x = startX; x < endX; x += 5 ) {
g.setColor( Color.YELLOW ); g.fillRoundRect( x, y - 20, 63, 18, 5, 5 ); g.setColor( Color.BLACK ); g.drawRoundRect( x, y - 20, 63, 18, 5, 5 ); g.drawString("Thank you!", x + 2, y - 5 ); try { Thread.sleep( ( int )( 100 ) ); } catch ( InterruptedException e ) { e.printStackTrace( ); } // erase g.setColor( eraseColor ); g.fillRect( x, y - 70, 65, 70 ); } } }
/* Item * Anderson, Franceschi */ import java.awt.Graphics; import java.awt.Color;
public abstract class Item { protected double price;
public Item( double p ) { price = p; }
public void setPrice( double newPrice ) { price = ( newPrice > 0 ? newPrice : 0 ); }
public double getPrice( ) { return price; }
public abstract void draw( Graphics g, int startX, int endX, int y, Color eraseColor );
}
/* Milk * Anderson, Franceschi */ import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat;
public class Milk extends Item {
public Milk( double p ) { super( p ); }
public void draw( Graphics g, int startX, int endX, int y, Color eraseColor ) { DecimalFormat money = new DecimalFormat( "$0.00" );
String display1 = "Milk: Unit price = " + money.format( price );
g.setColor( Color.BLUE ); g.drawString( display1, 20, 65 );
for ( int x = startX; x < endX; x += 5 ) { g.setColor( Color.WHITE ); g.fillRect( x +1, y - 67, 30, 65 ); g.setColor( Color.LIGHT_GRAY ); g.drawLine( x, y - 55, x + 30, y - 55 ); g.drawRect( x, y - 68, 31, 66 ); g.setColor( Color.BLACK ); g.drawString( "Milk", x + 3, y - 44 ); try { Thread.sleep( ( int )( 100 ) ); } catch ( InterruptedException e ) { e.printStackTrace( ); } // erase g.setColor( eraseColor ); g.fillRect( x, y - 70, 50, 70 ); } } }
/* OrangeJuice * Anderson, Franceschi */ import java.awt.Graphics; import java.awt.Color; import java.text.DecimalFormat;
public class OrangeJuice extends Item { public OrangeJuice( double p ) { super( p ); }
public void draw( Graphics g, int startX, int endX, int y, Color eraseColor ) { DecimalFormat money = new DecimalFormat( "$0.00" );
String display1 = "Orange Juice: Unit price = " + money.format( price );
g.setColor( Color.BLUE ); g.drawString( display1, 20, 65 );
for ( int x = startX; x < endX; x += 5 ) { g.setColor( new Color( 244, 244, 102 ) ); g.fillRect( x, y - 68, 30, 65 ); g.setColor( Color.LIGHT_GRAY ); g.drawLine( x, y - 52, x + 30, y - 52 ); g.setColor( Color.BLACK ); g.drawString("OJ", x + 7, y - 38 ); g.setColor( new Color( 255, 132, 41 ) ); g.fillOval( x + 8, y - 33, 15, 17 ); try { Thread.sleep( ( int )( 100 ) ); } catch ( InterruptedException e ) { e.printStackTrace( ); } // erase g.setColor( eraseColor ); g.fillRect( x, y - 70, 50, 70 ); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
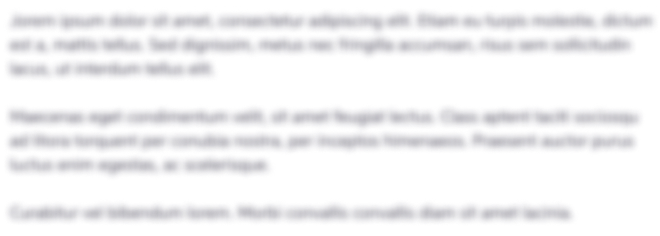
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started