Answered step by step
Verified Expert Solution
Question
1 Approved Answer
class BankAccount { private String customerName; private double savingAccountBalance; private double checkingAccountBalance; / / Constructor public BankAccount ( String newName, double amt 1 , double
class BankAccount
private String customerName;
private double savingAccountBalance;
private double checkingAccountBalance;
Constructor
public BankAccountString newName, double amt double amt
this.customerName newName;
if amount amt is less than
ifamt
amt;
if amount amt is less than
ifamt
amt;
this.checkingAccountBalance amt;
this.savingAccountBalance amt;
methods
public String getCustomerName
return customerName;
public void setCustomerNameString newName
this.customerName newName;
public double getSavingAccountBalance
return savingAccountBalance;
public void setSavingAccountBalancedouble amt
this.savingAccountBalance amt;
public double getCheckingAccountBalance
return checkingAccountBalance;
public void setCheckingAccountBalancedouble amt
this.checkingAccountBalance amt;
add parameter amt to the checking account balance only if positive
public void depositCheckingdouble amt
ifamt
this.checkingAccountBalance this.checkingAccountBalance amt;
add parameter amt to the savings account balance only if positive
public void depositSavingsdouble amt
ifamt
this.savingAccountBalance this.savingAccountBalance amt;
subtract parameter amt from the checking account balance only if positive
public void withdrawCheckingdouble amt
ifamt
ifthischeckingAccountBalanceamt
this.checkingAccountBalance this.checkingAccountBalanceamt;
else
System.out.printlnInsufficient balance";
subtract parameter amt from the savings account balance only if positive
public void withdrawSavingsdouble amt
ifamt
ifthissavingAccountBalanceamt
this.savingAccountBalance this.savingAccountBalanceamt;
else
System.out.printlnInsufficient balance";
subtract parameter amt from the checking account balance and add to the savings account balance only if positive
public void transferToSavingsdouble amt
ifamt
ifthischeckingAccountBalanceamt
this.checkingAccountBalance this.checkingAccountBalance amt;
this.savingAccountBalance this.savingAccountBalance amt;
else
System.out.printlnInsufficient balance";
@Override
public String toString
return "customerName : this.customerName
Saving Account Balance: this.savingAccountBalance
Checking Account Balance: this.checkingAccountBalance;
drive class
public class BankAccountTest
public static void mainString args
create a object of BankAccount class
BankAccount obj new BankAccountAmit;
System.out.printlnAccount Details ;
System.out.printlnobj;
System.out.println
;
obj.depositSavings;
System.out.println
Saving Account Balance after deposit : obj.getSavingAccountBalance;
obj.depositChecking;
System.out.println
Checking Account Balance after deposit : obj.getCheckingAccountBalance;
obj.withdrawSavings;
System.out.println
Saving Account Balance after withdraw : obj.getSavingAccountBalance;
obj.withdrawChecking;
System.out.println
Checking Account Balance after withdraw : obj.getCheckingAccountBalance;
obj.transferToSavings;
System.out.println
Checking Account Balance after transfer to Saving Account: obj.getCheckingAccountBalance;
System.out.printlnSaving Account Balance after transfer : obj.getSavingAccountBalance;
obj.depositSavings;
System.out.println
Saving Account Balance after deposit : obj.getSavingAccountBalance;
obj.depositChecking;
System.out.println
Checking Account Balance after deposit : obj.getCheckingAccountBalance;
obj.withdrawSavings;
System.out.println
Saving Account Balance after withdraw : obj.getSavingAccountBalance;
System.out.print
Withdraw from Checking Account Balance: ;
obj.withdrawChecking;
System.out.printlnChecking Account Balance after withdraw : obj.getCheckingAccountBalance;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
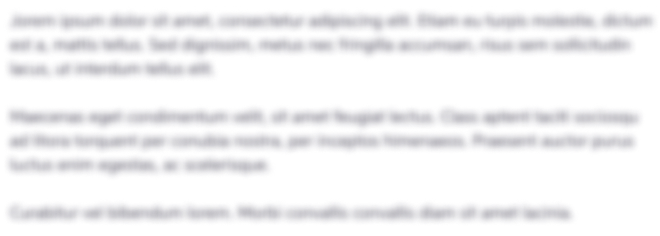
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started