Question
class DoubleHashTable: def __init__(self, size=7, secondary_hash_value=5): self.size = size self.slots = [None] * size self.secondary_hash_value = secondary_hash_value def __str__(self): return str(self.slots) def get_hash_index(self, key): return
class DoubleHashTable: def __init__(self, size=7, secondary_hash_value=5): self.size = size self.slots = [None] * size self.secondary_hash_value = secondary_hash_value
def __str__(self): return str(self.slots)
def get_hash_index(self, key): return key % self.size
def clear(self): self.slots = [None] * self.size
def is_empty(self): return self.__len__() == 0
def __len__(self): return sum([1 if item != None else 0 for item in self.slots])
def put(self, key): original_index = index = self.get_hash_index(key) step = 1 step_size = self.get_step_size(key) while self.slots[index] != None: index = (original_index + step * step_size) % self.size step += 1 self.slots[index]=key
def get_step_size(self, key): return self.secondary_hash_value - (key % self.secondary_hash_value)
def primes(n): n_plus_1 = n + 1 num_list = list(range(n_plus_1)) num_list[1] = 0 square_root_n = int(round(n**0.5)) for i in range(2, square_root_n + 1): if num_list[i]: num_list[i*i: n_plus_1: i] = [0] * len(range(i*i, n_plus_1, i)) return list(filter(lambda x: x >= 1,num_list))
Consider the DoubleHashTable class definition in the answer box below, which implements a hash table with double hashing. The method get_hash_index (self, key) implements the first hash function using the variable size. The method get_step_size (self, key) implements the second hash function using the variable secondary_hash_value, and defines the step size for the probe sequence. Write a method named secondary_hash_value_test (size, table_values) which tests how many collisions occur for all possible secondary hash values when inserting the items of the list table_values into an initially empty hash table. The method should return a list of tuples where the first element of each tuple is the secondary hash value (all primes smaller than the table size), and the second element is the number of collisions occurring when using this value for the second hash function. NOTE: You might want to modify the put () method to return the number of collisions. Example: The result of secondary_hash_value_test (7,[11,15,43,9,45]) is [(2,4),(3,2),(5,3)], since the prime numbers smaller than 7 are 2, 3, and 5 , and when using these primes for the secondary hash function and inserting [11,15,43,9,45] into a hash table of size 7 , then the number of collisions are 4,2 , and 3 , respectively. For example: Answer: (penalty regime: 0,0,5,10,15,20,25,30,35,40,45,50% )Step by Step Solution
There are 3 Steps involved in it
Step: 1
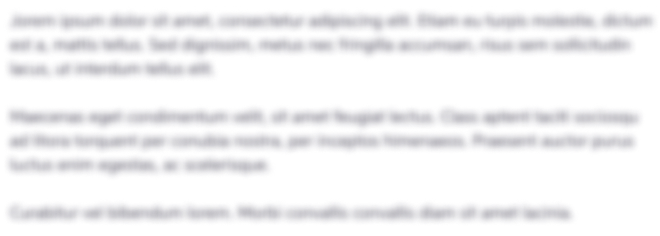
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started