Question
class Node: def __init__(self, init_data): self.data = init_data self.next = None def get_data(self): return self.data def get_next(self): return self.next def set_data(self, new_data): self.data = new_data
class Node: def __init__(self, init_data): self.data = init_data self.next = None
def get_data(self): return self.data
def get_next(self): return self.next
def set_data(self, new_data): self.data = new_data
def set_next(self, new_next): self.next = new_next
class LinkedListIterator: def __init__( self, head): self.current = head
def __next__( self ): if self.current != None: item = self.current.get_data() self.current = self.current.get_next() return item else : raise StopIteration class UnOrderedList: def __init__(self): self.head = None
def is_empty(self): return self.head == None
def size(self): curr = self.head count = 0 while curr != None: count = count + 1 curr = curr.get_next() return count def add(self, item): new_node = Node(item) new_node.set_next(self.head) self.head = new_node
def search(self,item): current = self.head while current != None: if current.get_data() == item: return True else: current = current.get_next() return False
def remove(self,item): current = self.head previous = None found = False while not found: if current.get_data() == item: found = True else: previous = current current = current.get_next()
if previous == None: self.head = current.get_next() else: previous.set_next(current.get_next())
def __iter__(self): return LinkedListIterator(self.head)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
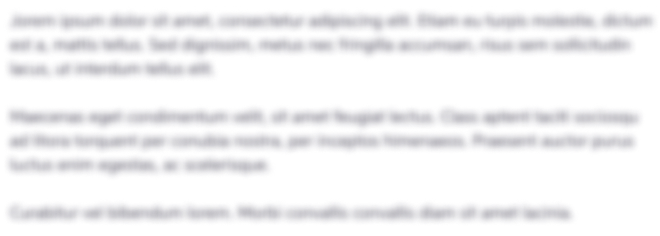
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started