Question
Class Person: Constructor: A Person can have any name, but the age needs to be a value between 1 and 150 (inclusive). Throw an IllegalArgumentException
- Class Person:
- Constructor: A Person can have any name, but the age needs to be a value between 1 and 150 (inclusive). Throw an IllegalArgumentException if an invalid age is provided and include an error message that states that age needs to be from the range [1, 150].
- The method toString should return a string of the following format: {name}({age}) {name}, and {age} stand for the corresponding field values. Something similar is true whenever you see a reference to a field in curly braces throughout this assignment. E.g.: Chloe(24)
- Class Friend:
- The method chill should return a string of the following format {name} is chilling E.g., Ben is chilling
- The method play returns a string that depends on the number of friends in the array that is passed as an argument
- In case of an empty list (no friend): playing {hobby}
- In the case of one friend (list with one element): playing {hobby} with {friend}
- In the case of two friends (list with two friends): playing {hobby} with {friend1} and {friend2}
- If the argument list contains more than two friends: playing {hobby} with {friend1}, {friend2}, . . , and {friendN} Notice that the friends are separated by comma and space plus the word and before the last friend
In all cases {hobby} should be replaced with the corresponding field value, and the individual friends should be represented by their names. E.g., playing music E.g., playing games with Alex E.g., playing music with Chris and Chloe E.g., playing sports with Dan, Lisa, and Ben
-
- The method toString should return the same string as class Person plus a space and the hobby : {name}({age}) {hobby} E.g.: Chloe(24) GAMES
- Class Telemarketer:
- The method giveSalesPitch should return a string of the following format {name} pressures others to buy stuff E.g., Chap pressures others to buy stuff
- The overridden method annoy should return a string of the following format {name} annoys by giving a sales pitch E.g., Chap annoys by giving a sales pitch
- Class Insect:
- The method toString should return a string of the following format: {className}: {species} E.g.: Insect: wasp
- Class Butterfly:
- Class Butterfly has two constructors. One initializes the fields based on the individual field values that are provided, the other initializes the fields based on an existing Butterfly object. Keep in mind the requirement that all classes in this assignment need to be immutable, and avoid code duplication.
- The field colors is a list of colors. The type of the list should be interface List
(Links to an external site.), not a class like ArrayList , which implements interface List . This provides flexibility and allows the clients to choose which type of list works best in their context. You'll need the following import statement: import java.util.List; - The method toString should return a string of the following format: {species} [{color1}, {color2}, . . , {colorN}] {color1}, {color2}, {colorN} are substituted with the individual colors in the list. If no color has been provided, the empty brackets are added to the string. E.g.: Monarch [yellow, orange, black] E.g.: Morpho [blue] E.g.: Phoebis []
- Class Mosquito:
- The method buzz should return the string of the following format: {species} buzzing around E.g.: Culex tarsalis buzzing around
- The overridden method annoy should return the string buzz buzz buzz
- Class PeskyMosquito:
- The method bite should return the string sucking blood
- Overriding the method equals: The method equals is not included in the UML class diagram. That's because I want you to determine where to override it in order to provide the following desired behavior:
- Desired behavior: I want to be able to determine whether two friends are equal at runtime. In other words: When I create two Friend objects, and both friends have the same name, the same age, and the same hobby, then those two friends should be equal. Otherwise, they should not be equal.
Add a class named NuisanceApp to the project. It is the test client and includes the main method. For details like titles, descriptions, empty lines to group the output, refer to the Expected Output below. As the name suggests, this is how your output should look like.
- Test Persons:
- Create an array that includes three friends: Juan 27 hobby music, Kate 27 hobby sports, and Alex 21 hobby music.
- Print a title. Then use a loop to print the three array elements.
- Compare friend1 with friend2 and friend 1 with friend3 and print the results
- Create a new friend: Kate 27 hobby sports Compare friend2 with the new friend and print the result
- Create a telemarketer: Fritz 25 and print the telemarketer
- Test Insects:
- Create a mosquito: species Aedes vexans Create a pesky mosquito: species Anopheles walkeri Create a butterfly: species Swallowtail and colors yellow, black, blue Use the copy constructor to create a second butterfly that is also a Swallowtail with the same colors.
- Create an array of insects and initialize it with the mosquito, the pesky mosquito, and both butterflies
- Print a title. Then use a loop to print the insects
- Test interface Nuisance
- Create an array of type Nuisance. It includes the telemarketer, the mosquito, and the pesky mosquito.
- Print a title. Then loop through the array elements. For each of the array elements do the following:
- if it is a mosquito, buzz
- if it is a pesky mosquito, bite
- regardless, which nuisance it is, annoy
Sample Output:
3 Friends: friend1: Juan(27) MUSIC friend2: Kate(27) SPORTS friend3: Alex(21) MUSIC friend1 equals friend2: false friend1 equals friend3: false friend2 equals new friend: true telemarketer: Fritz(25) 4 Insects: Mosquito: Aedes vexans PeskyMosquito: Anopheles walkeri Swallowtail [red, blue, yellow] Swallowtail [red, blue, yellow] 3 Nuisances: Fritz annoys by giving a sales pitch Aedes vexans buzzing around buzz buzz buzz Anopheles walkeri buzzing around sucking blood buzz buzz buzz
Implementation of the UML class diagram Implement the classes and the interface as specified in this UML class diagram. All classes should be immutable. All public classes, constructors, and methods need a doc comment. No public class members should be added, modified or removed. Person -name: String - o:int + Person[name : String, age: int) + getName(): String + getAge(): int + toString(): String a interface Nuisance annoyo): String - species : String + Insect species : String) + getSpecies): String + toString(): String Mosquito Telemarketer + buzz): String + give Sales Pitch(): String Butterfly - colors: ListStep by Step Solution
There are 3 Steps involved in it
Step: 1
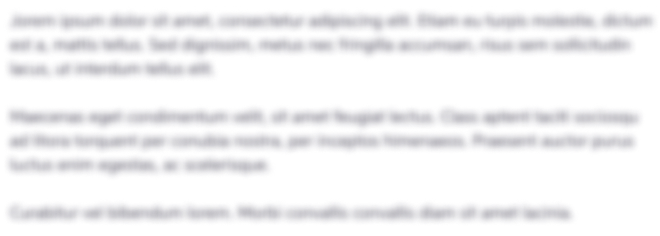
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started