Question
class TEntry { private K key; private V value; public TEntry(K key, V value) { this.key = key; this.value = value; } public K getKey()
class TEntry
{
private K key;
private V value;
public TEntry(K key, V value)
{
this.key = key;
this.value = value;
}
public K getKey() {
return key;
}
public V getValue() {
return value;
}
public String toString() {
return key.toString()+":"+value.toString();
}
}
Write what ever methods you can thank you using the TEntry for Table class
class SymbolTable
{
private TableEntry
private int numE;
private int max;
@SuppressWarnings("unchecked")
public SymbolTable(int size)
{
//Create a hash table where the initial storage
//is size and string keys can be mapped to T values.
//You may assume size is >= 2
storage = new TableEntry[size];
numE = 0;
max = 0;
}
/**
* @return how big the table is
*/
public int getCapacity()
{
return max;
}
/**
* @return the number of elements in table
*/
public int size()
{
return numE;
}
public void put(String k, T v)
{
//Place value v at the location of key k.
//Use linear probing if that location is in use.
//You may assume both k and v will not be null.
//Hint: Make a TableEntry to store in storage
//and use the absolute value of k.hashCode() for
//the probe start.
//If the key already exists in the table
//replace the current value with v.
//If the key isn't in the table and the table
//is >= 80% full, expand the table to twice
//the size and rehash
//Worst case: O(n), Average case: O(1)
TableEntry
int pos = Math.abs(v.hashCode()) % storage.length;
if(storage[pos].getKey() == k)
{
pair = new TableEntry<>(k, v);
storage[pos] = pair;
}
if(storage[pos] == null)
{
storage[pos] = pair;
}
}
public T remove(String k)
{
//Remove the given key (and associated value)
//from the table. Return the value removed.
//If the value is not in the table, return null.
//Hint 1: Remember to leave a tombstone!
//Hint 2: Does it matter what a tombstone is?
// Yes and no... You need to be able to tell
// the difference between an empty spot and
// a tombstone and you also need to be able
// to tell the difference between a "real"
// element and a tombstone.
//Worst case: O(n), Average case: O(1)
return null;
}
public T get(String k)
{
//Given a key, return the value from the table.
//If the value is not in the table, return null.
//Worst case: O(n), Average case: O(1)
return null;
}
// private boolean contains(T v)
// {
// int pos = Math.abs(v.hashCode()) % storage.length;
// if (storage[pos] == null)
// {
// return false;
// }
// if (storage[pos].contains(v))
// {
// return true;
// }
//
// return false;
//
// }
public boolean isTombstone(int index)
{
//this is a helper method needed for printing
//return whether or not there is a tombstone at the
//given index
//O(1)
return false;
}
@SuppressWarnings("unchecked")
public boolean rehash(int size)
{
//Increase or decrease the size of the storage,
//rehashing all values.
//If the new size won't fit all the elements,
//return false and do not rehash. Return true
//if you were able to rehash.
return null;
}
//--------------Provided methods below this line--------------
//Add JavaDocs, but do not change the methods.
public String toString() {
//THIS METHOD IS PROVIDED, DO NOT CHANGE IT
StringBuilder s = new StringBuilder();
for(int i = 0; i < storage.length; i++) {
if(storage[i] != null && !isTombstone(i)) {
s.append(storage[i] + " ");
}
}
return s.toString().trim();
}
public String toStringDebug() {
//THIS METHOD IS PROVIDED, DO NOT CHANGE IT
StringBuilder s = new StringBuilder();
for(int i = 0; i < storage.length; i++) {
if(!isTombstone(i)) {
s.append("[" + i + "]: " + storage[i] + " ");
}
else {
s.append("[" + i + "]: tombstone ");
}
}
return s.toString().trim();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
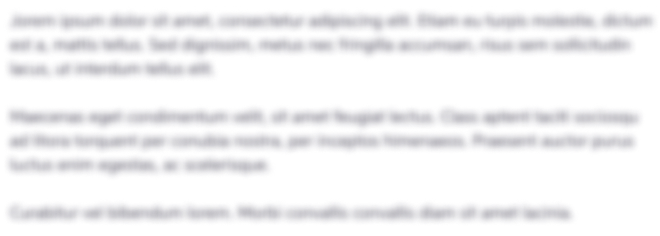
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started