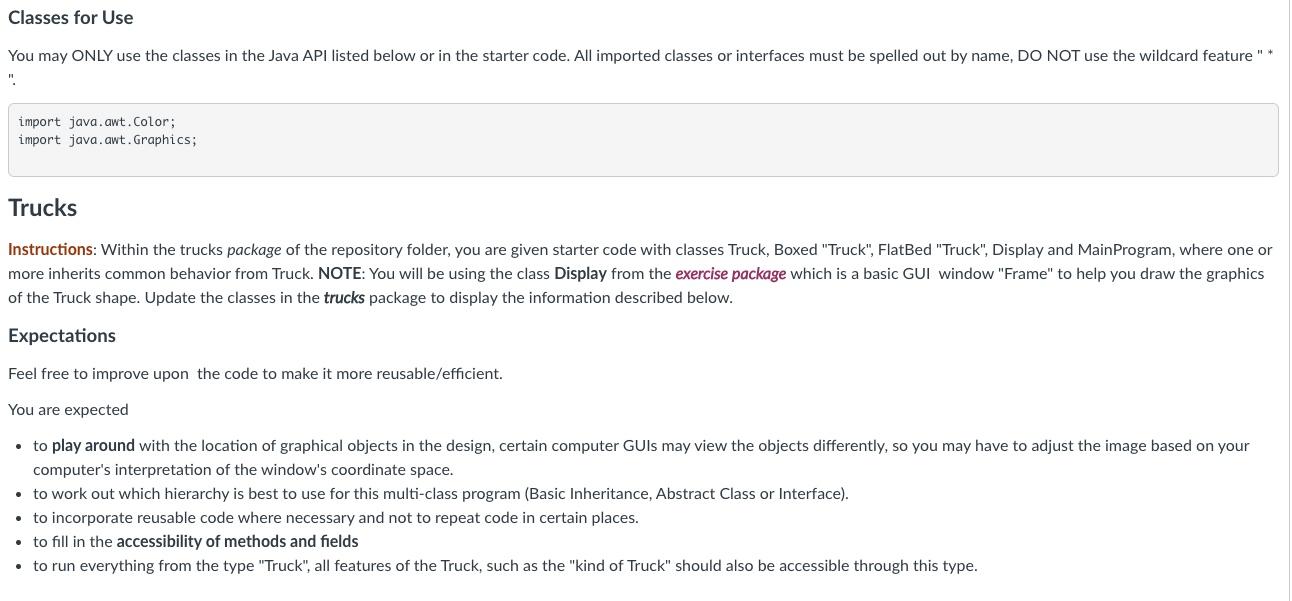
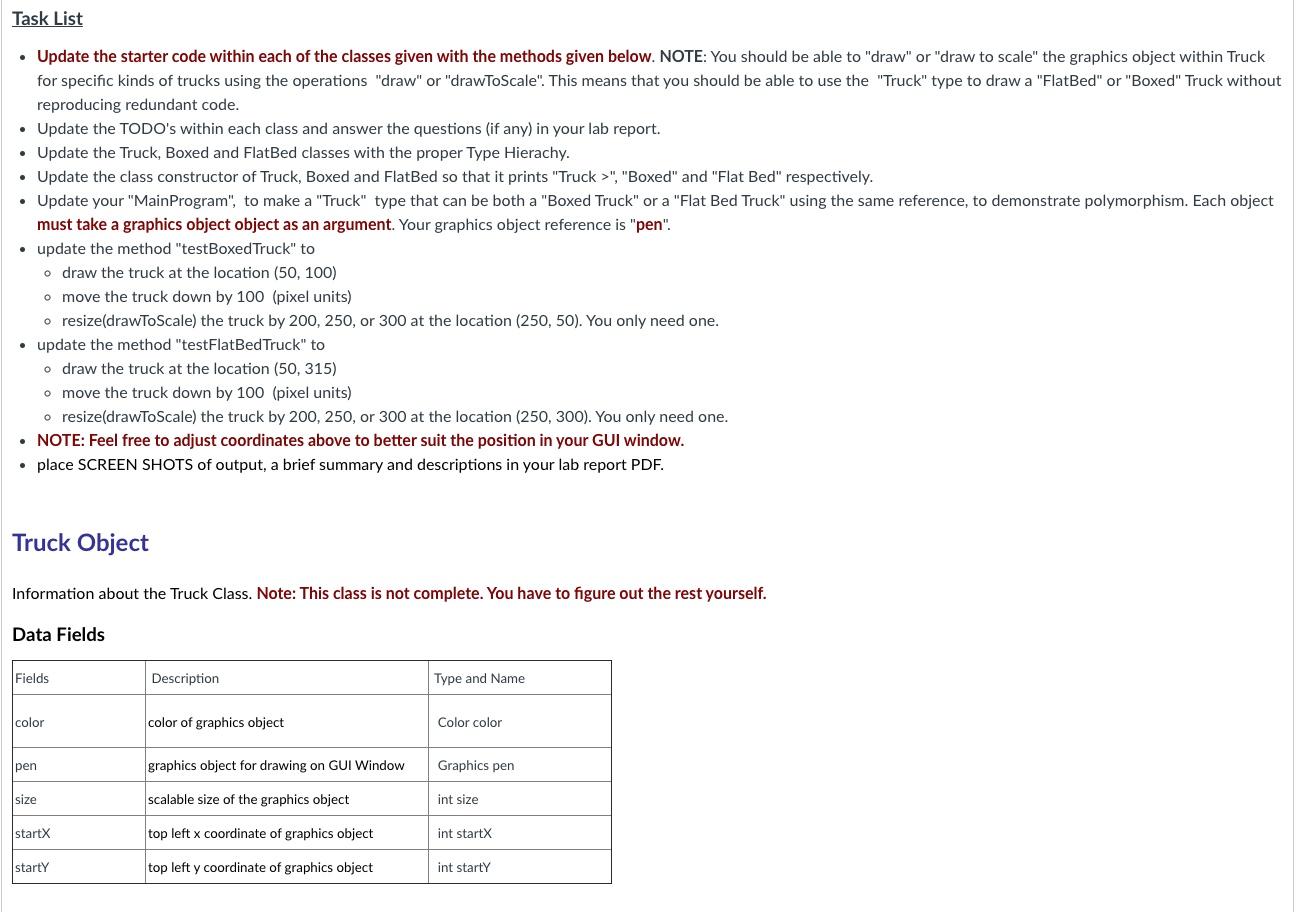
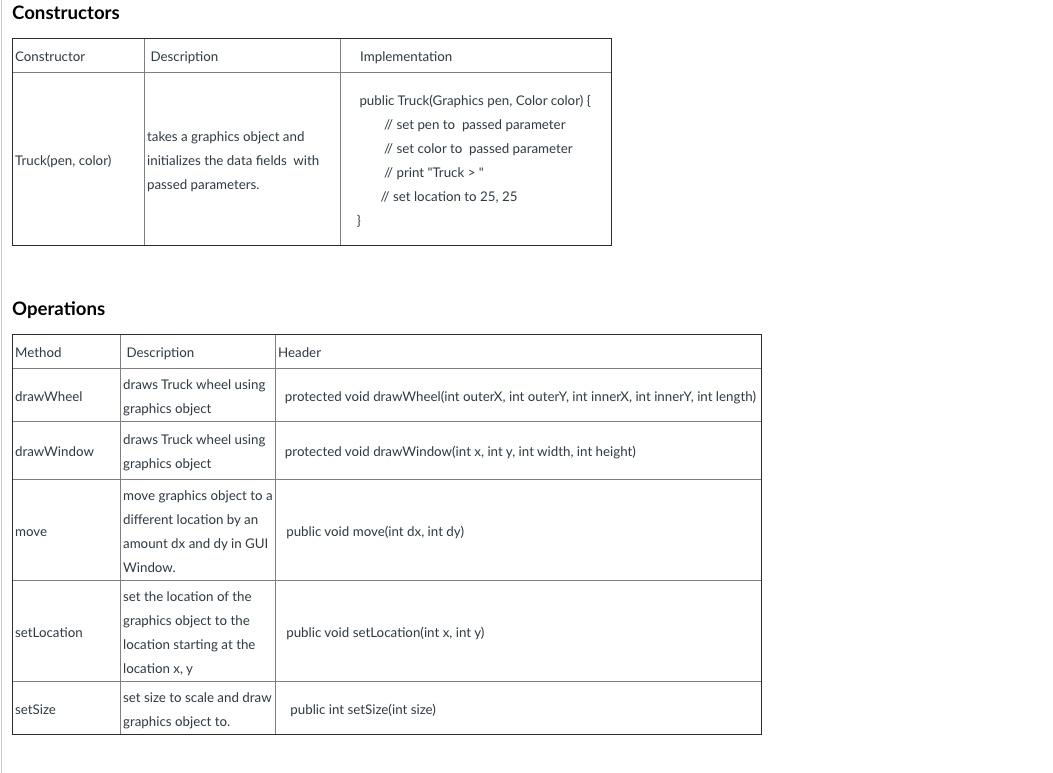
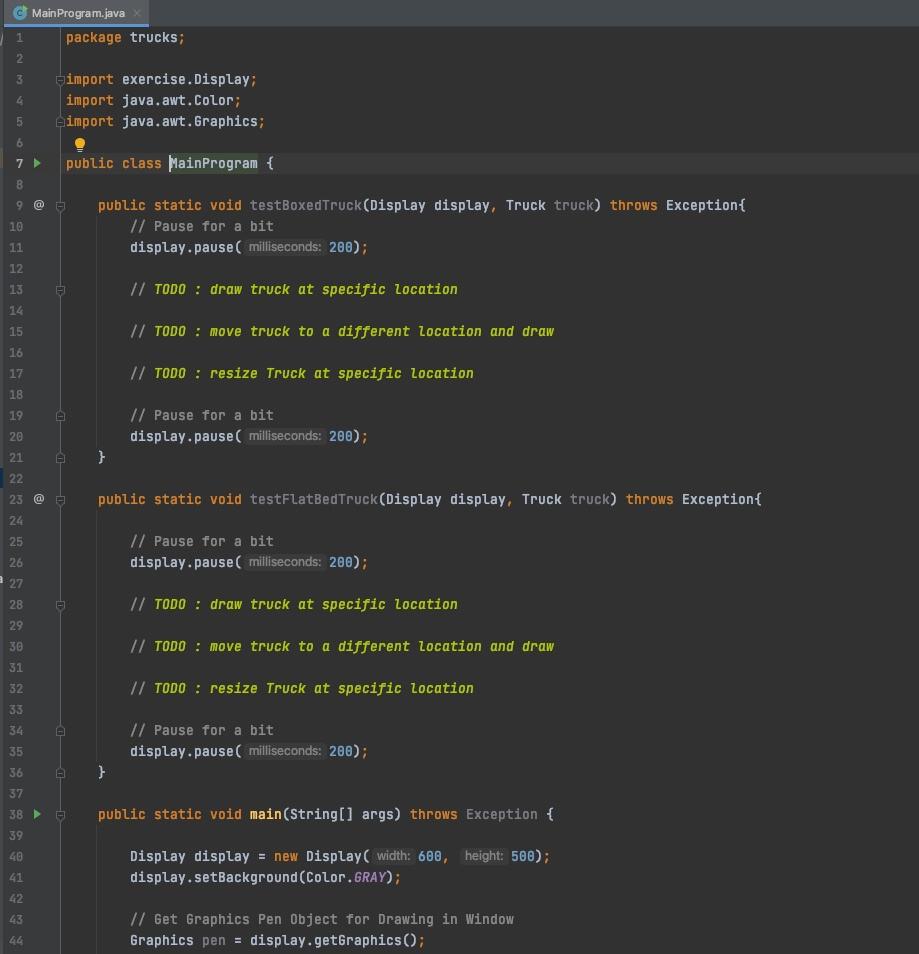
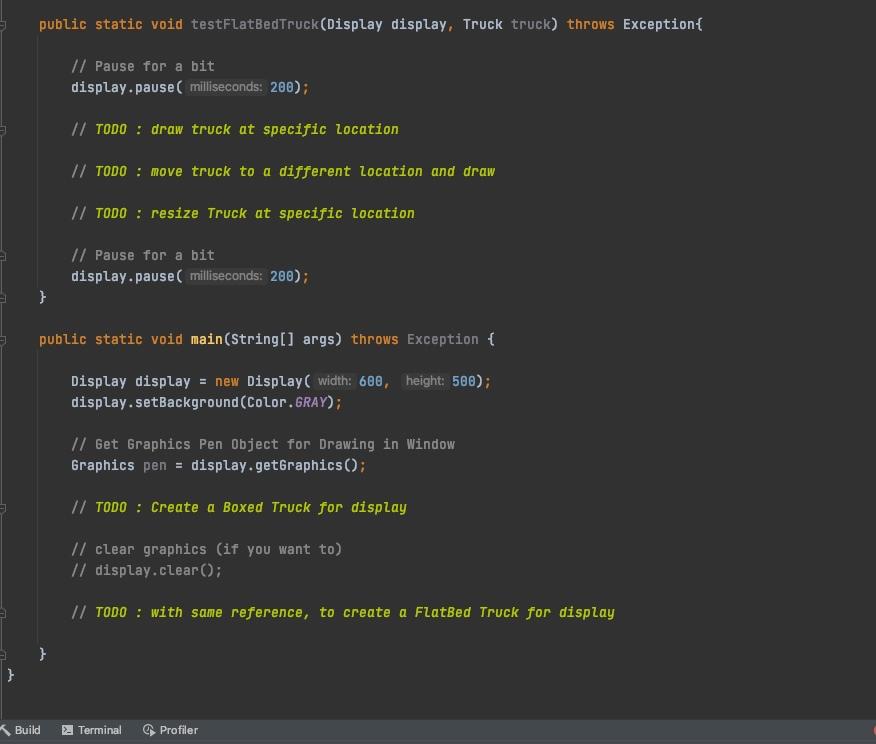
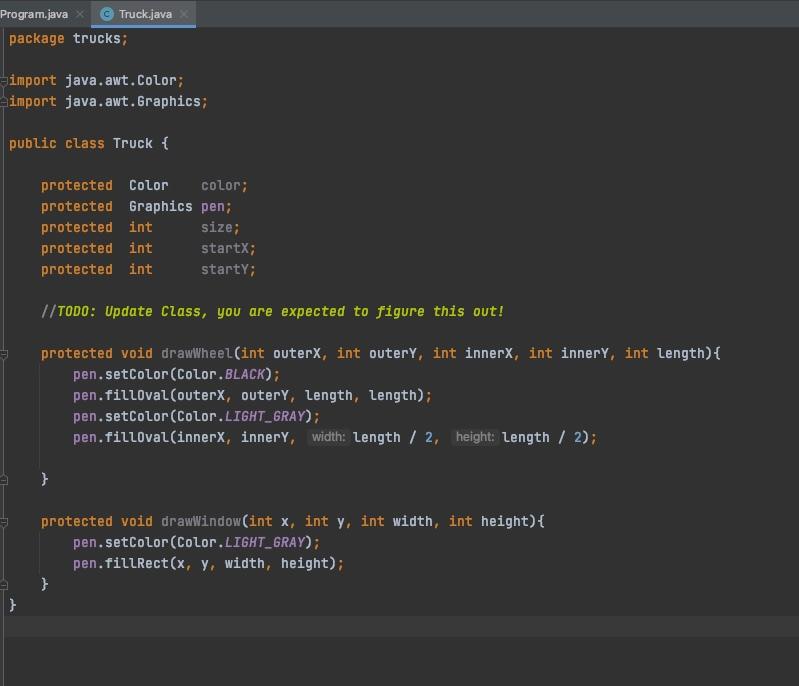
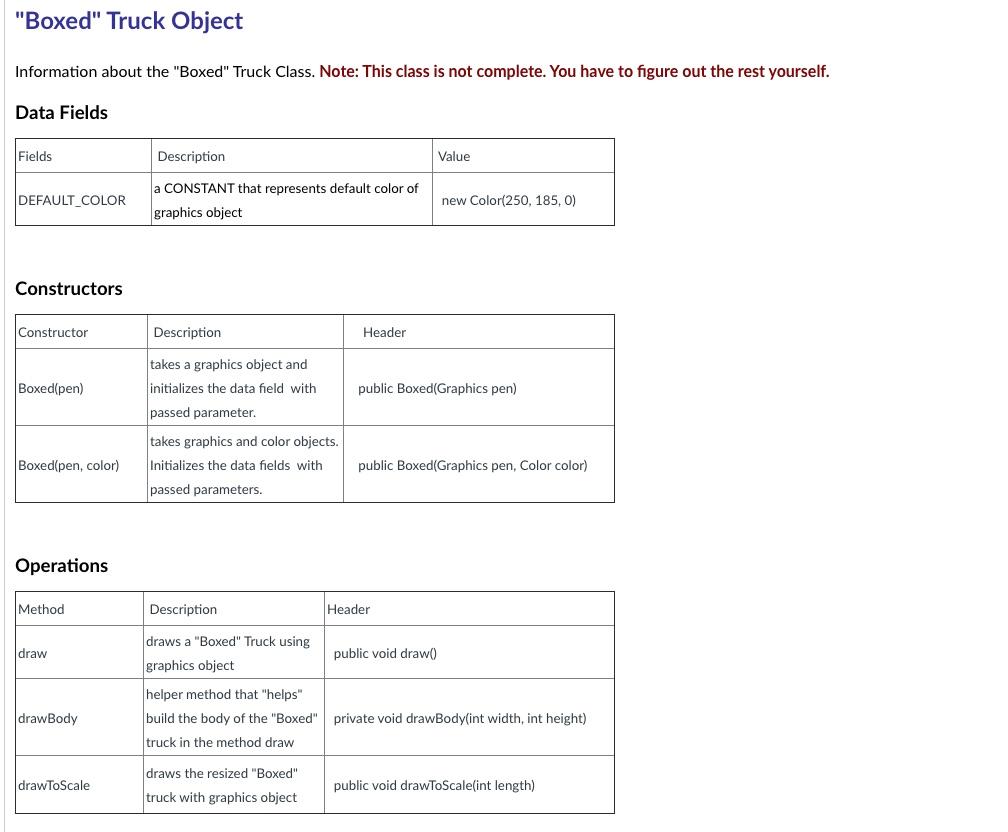
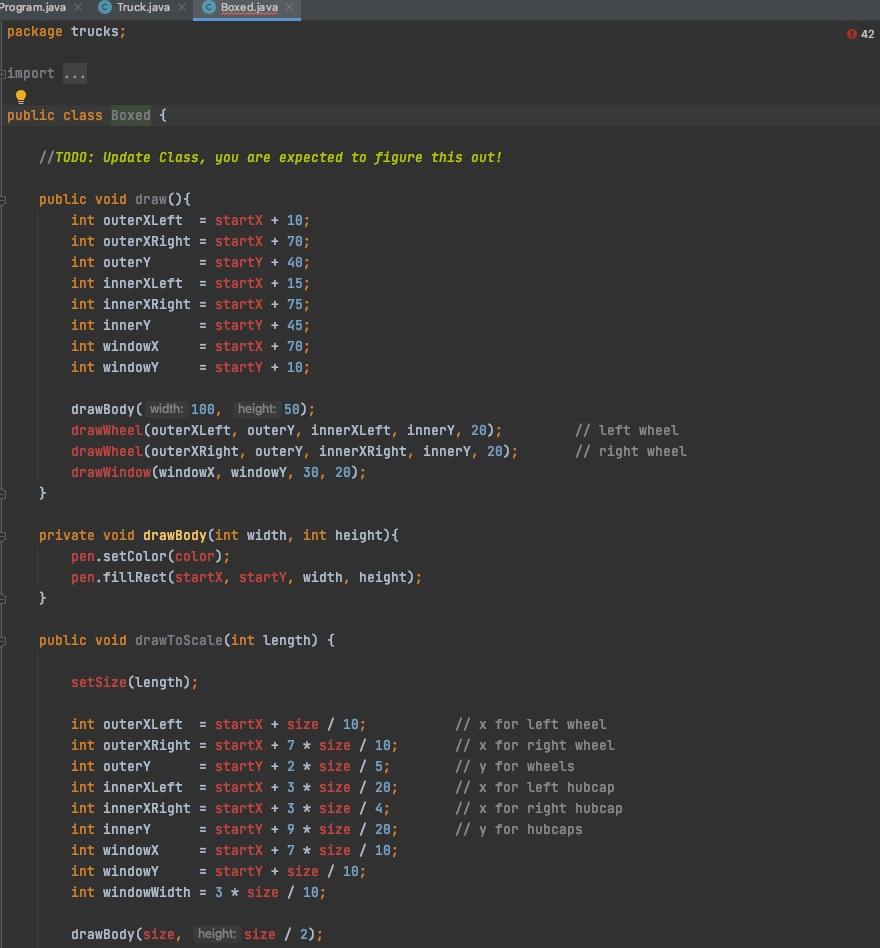
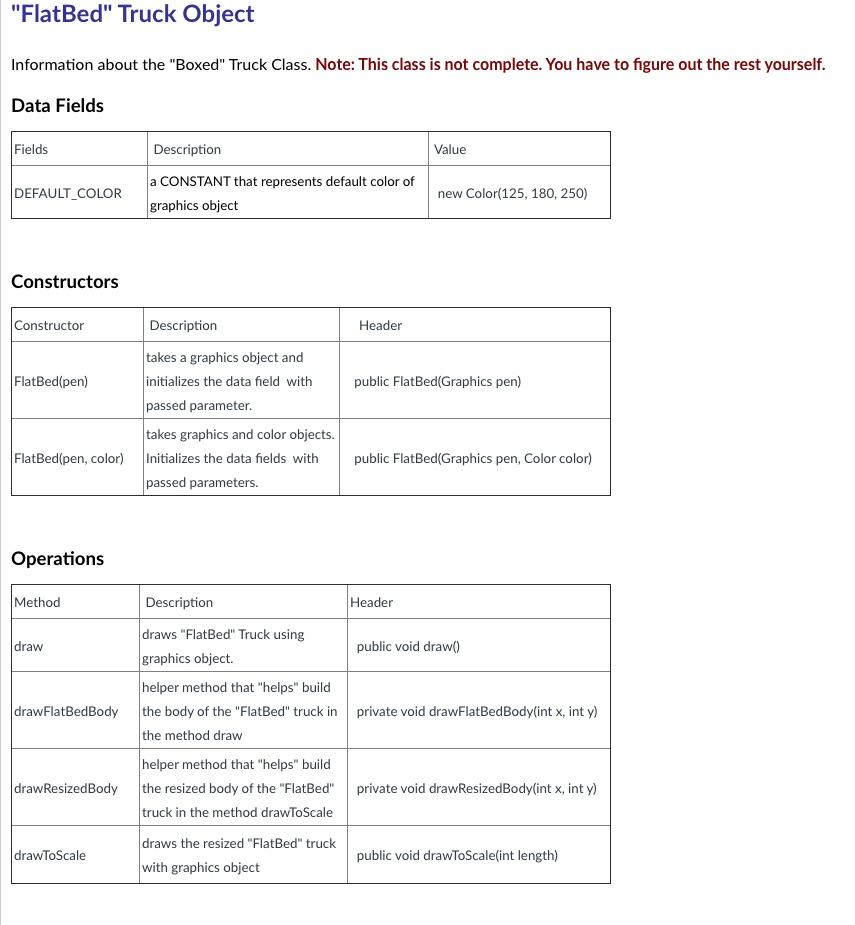
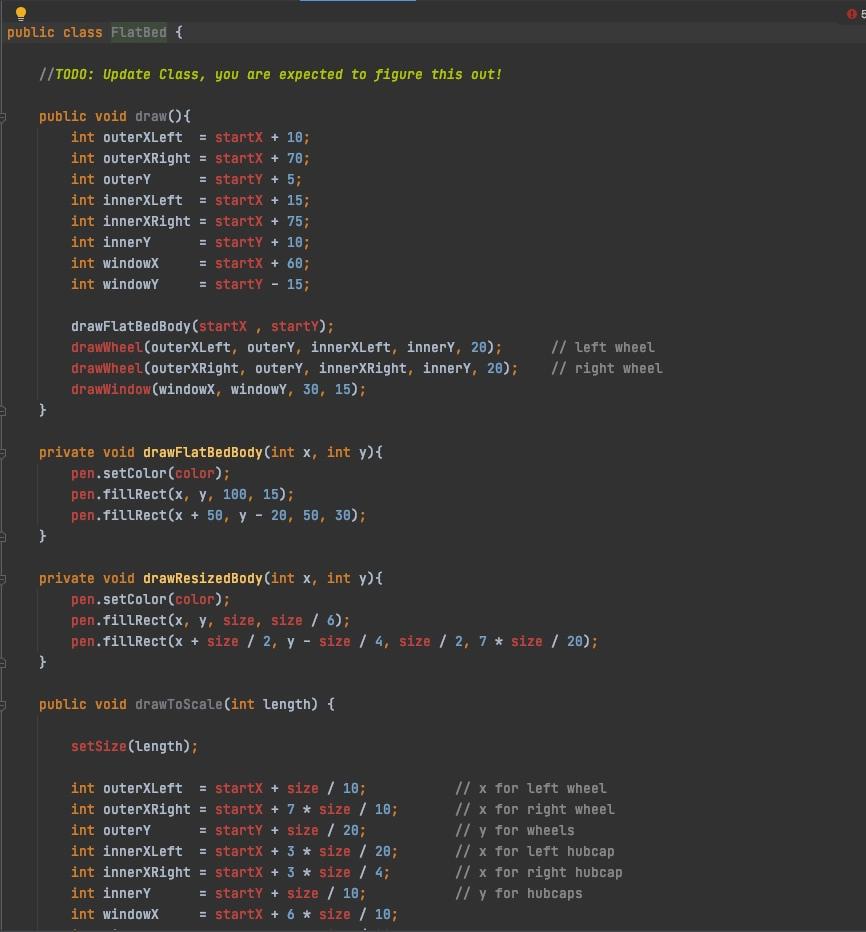
Classes for Use You may ONLY use the classes in the Java API listed below or in the starter code. All imported classes or interfaces must be spelled out by name, DO NOT use the wildcard feature" import java.awt.Color; import java.awt.Graphics; Trucks Instructions: Within the trucks package of the repository folder, you are given starter code with classes Truck, Boxed "Truck", FlatBed "Truck", Display and Main Program, where one or more inherits common behavior from Truck. NOTE: You will be using the class Display from the exercise package which is a basic GUI window "Frame" to help you draw the graphics of the Truck shape. Update the classes in the trucks package to display the information described below. Expectations Feel free to improve upon the code to make it more reusable/efficient. You are expected to play around with the location of graphical objects in the design, certain computer GUls may view the objects differently, so you may have to adjust the image based on your computer's interpretation of the window's coordinate space. to work out which hierarchy is best to use for this multi-class program (Basic Inheritance, Abstract Class or Interface). to incorporate reusable code where necessary and not to repeat code in certain places. to fill in the accessibility of methods and fields to run everything from the type "Truck", all features of the Truck, such as the "kind of Truck" should also be accessible through this type. Constructors Constructor Description Implementation public Truck(Graphics pen, Color color) { // set pen to passed parameter // set color to passed parameter // print "Truck > // set location to 25, 25 takes a graphics object and initializes the data fields with passed parameters. Truck(pen, color) Operations Method Description Header draw Wheel draws Truck wheel using graphics object protected void drawWheel(int outerX, int outery, int innerX, int innery, int length) draw Window draws Truck wheel using graphics object protected void drawWindow(int x, int y, int width, int height) move graphics object to a different location by an move public void move(int dx, int dy) amount dx and dy in GUI Window. setLocation set the location of the graphics object to the location starting at the location x, y public void setLocation(int x, int y) setSize set size to scale and draw graphics object to public int setSize(int size) Main Program.java package trucks; 1 2 3 4 -import exercise.Display: import java.awt.Color; import java.awt.Graphics; 5 6 7 public class MainProgram { 9 @ HT 10 public static void testBoxedTruck (Display display, Truck truck) throws Exception{ // Pause for a bit display.pause( milliseconds: 200); 11 12 13 // TODO : draw truck at specific location 14 15 // TODO : move truck to a different location and draw 16 17 // TODO : resize Truck at specific Location 18 19 // Pause for a bit display.pause( milliseconds: 200); 20 21 22 23 @ public static void testflatBedTruck(Display display, Truck truck) throws Exception 24 25 // Pause for a bit display.pause( milliseconds: 200); 26 27 28 // TODO : draw truck at specific location 29 30 // TODO : move truck to a different location and draw 31 32 // TODO : resize Truck at specific location 33 34 // Pause for a bit display.pause milliseconds: 200); 35 36 37 38 public static void main(String[] args) throws Exception { 39 40 Display display = new Display( width: 600, height: 500); display.setBackground(Color.GRAY); 41 42 43 // Get Graphics Pen Object for Drawing in Window Graphics pen = display.getGraphics(); 44 public static void testFlatbedTruck(Display display, Truck truck) throws Exception // Pause for a bit display.pause( milliseconds: 200); // TODO : draw truck at specific location // TODO : move truck to a different location and draw // TODO : resize Truck at specific location // Pause for a bit display.pause( milliseconds: 200); } public static void main(String[] args) throws Exception { Display display = new Display( width: 600, height: 500); display.setBackground(Color.GRAY); // Get Graphics Pen Object for Drawing in Window Graphics pen = display.getGraphics(); // TODO : Create a Boxed Truck for display // clear graphics (if you want to // display.clear(); // TODO : with same reference, to create a Flatbed Truck for display Build Terminal Profiler Program.java Truck.java package trucks; import java.awt.Color; import java.awt.Graphics; public class Truck { protected Color color; protected Graphics pen; protected int size; protected int startX; protected int starty; //TODO: Update class, you are expected to figure this out! protected void drawWheel(int outerx, int outery, int innerx, int innery, int length) { pen.setColor(Color.BLACK); pen.filloval(outerx, outery, Length, Length); pen.setColor(Color.LIGHT_GRAY); pen.filloval(innerx, innery, width: Length / 2, height: Length / 2); protected void drawWindow(int x, int y, int width, int height) { pen.setColor(Color.LIGHT_GRAY); pen.fillRect(x, y, width, height); "Boxed" Truck Object Information about the "Boxed" Truck Class. Note: This class is not complete. You have to figure out the rest yourself. Data Fields Fields Description Value DEFAULT_COLOR a CONSTANT that represents default color of graphics object new Color(250, 185, 0) Constructors Constructor Description Header takes a graphics object and initializes the data field with Boxed(pen) public Boxed(Graphics pen) passed parameter. Boxed(pen, color) takes graphics and color objects. Initializes the data fields with passed parameters. public Boxed(Graphics pen, Color color) Operations Method Description Header draw draws a "Boxed" Truck using graphics object public void drawl drawBody helper method that "helps" build the body of the "Boxed" truck in the method draw private void drawBody(int width, int height) drawToScale draws the resized "Boxed" truck with graphics object public void drawToScale(int length) Boxed.java Program.java Truck.java package trucks; 42 import public class Boxed { //TODO: Update Class, you are expected to figure this out! public void draw() { int outerXLeft = startX + 10; int outerXRight = startX + 70; int outery = starty + 40; int innerXLeft = startX + 15; int innerXRight = startX + 75; int innery = starty + 45; int window = startX + 70; int window = starty + 10; drawBody( width: 100,height: 50); drawWheel(outerXLeft, outery, innerXLeft, innery, 20); drawWheet(outerXRight, outery, innerXRight, innery, 20); drawWindow(windowx, windowy, 30, 20); // left wheel // right wheel } private void drawBody(int width, int height) { pen.setColor(color); pen.fillRect(startx, starty, width, height); } public void drawToScale(int length) { setSize(Length); int outerXLeft = startX + size / 10; int outerXRight = startx + 7 * size / 10; int outery = starty + 2 * size / 5; int innerXLeft = startX + 3 * size / 20; int innerXRight = startX + 3 * size / 4; int innery = starty + 9 * size / 20; int windowX = startX + 7 * size / 10; int window = starty + size / 10; int windowWidth = 3 * size / 10; // x for left wheel // x for right wheel // y for wheels // x for left hubcap // x for right hubcap // y for hubcaps drawBody(size, height: size / 2); "FlatBed" Truck Object Information about the "Boxed" Truck Class. Note: This class is not complete. You have to figure out the rest yourself. Data Fields Fields Value Description a CONSTANT that represents default color of graphics object DEFAULT_COLOR new Color(125, 180, 250) Constructors Constructor Header Flated(pen) public FlatBed(Graphics pen) Description takes a graphics object and initializes the data field with passed parameter. takes graphics and color objects. Initializes the data fields with passed parameters. FlatBed(pen, color) public FlatBed(Graphics pen, Color color) Operations Method Description Header draws "Flatbed" Truck using draw public void drawn graphics object. helper method that "helps" build drawFlatBedBody the body of the "FlatBed" truck in private void drawFlatBedBody(int x, int y) the method draw helper method that "helps" build drawResizedBody the resized body of the "FlatBed" private void drawResizedBody(int x, int y) truck in the method drawToScale draws the resized "Flat Bed" truck drawToScale public void drawToScale(int length) with graphics object OE public class Flatbed { //TODO: Update Class, you are expected to figure this out! public void draw({ int outerXLeft = startX + 10; int outerXRight = startX + 70; int outery = starty + 5; int innerXLeft = startX + 15; int innerXRight = startX + 75; int innery = starty + 10; int windows = startX + 60; int window = starty - 15; drawFlatbedBody(start, starty); drawWheel (outerXLeft, outery, innerXLeft, innery, 20); drawWheelCouterXRight, outery, innerXRight, innery, 20); drawWindow(windowx, windowy, 30, 15); // left wheel // right wheel } private void drawFlatbedBody(int x, int y){ pen.setColor(color); pen.fillRect(x, y, 100, 15); pen.fillRect(x + 50, y - 20, 50, 30); } private void drawResizedBody(int x, int y){ pen.setColor(color); pen.fillRect(x, y, size, size / 6); pen.fillRect(x + size / 2. y - size 1 4, size / 2. 7 * size / 20); } public void drawToScale(int length) { setSize(Length); int outerXLeft int outerXRight int outery int innerXLeft int innerXRight int innery int windows = startX + size / 10; = startX + 7 * size / 10; = starty + size / 20; = startX + 3 * size / 20; = startX + 3 * size / 4; = starty + size / 10; = startX + 6 * size / 10; // x for left wheel // x for right wheel // y for wheels // x for left hubcap // x for right hubcap // y for hubcaps Classes for Use You may ONLY use the classes in the Java API listed below or in the starter code. All imported classes or interfaces must be spelled out by name, DO NOT use the wildcard feature" import java.awt.Color; import java.awt.Graphics; Trucks Instructions: Within the trucks package of the repository folder, you are given starter code with classes Truck, Boxed "Truck", FlatBed "Truck", Display and Main Program, where one or more inherits common behavior from Truck. NOTE: You will be using the class Display from the exercise package which is a basic GUI window "Frame" to help you draw the graphics of the Truck shape. Update the classes in the trucks package to display the information described below. Expectations Feel free to improve upon the code to make it more reusable/efficient. You are expected to play around with the location of graphical objects in the design, certain computer GUls may view the objects differently, so you may have to adjust the image based on your computer's interpretation of the window's coordinate space. to work out which hierarchy is best to use for this multi-class program (Basic Inheritance, Abstract Class or Interface). to incorporate reusable code where necessary and not to repeat code in certain places. to fill in the accessibility of methods and fields to run everything from the type "Truck", all features of the Truck, such as the "kind of Truck" should also be accessible through this type. Constructors Constructor Description Implementation public Truck(Graphics pen, Color color) { // set pen to passed parameter // set color to passed parameter // print "Truck > // set location to 25, 25 takes a graphics object and initializes the data fields with passed parameters. Truck(pen, color) Operations Method Description Header draw Wheel draws Truck wheel using graphics object protected void drawWheel(int outerX, int outery, int innerX, int innery, int length) draw Window draws Truck wheel using graphics object protected void drawWindow(int x, int y, int width, int height) move graphics object to a different location by an move public void move(int dx, int dy) amount dx and dy in GUI Window. setLocation set the location of the graphics object to the location starting at the location x, y public void setLocation(int x, int y) setSize set size to scale and draw graphics object to public int setSize(int size) Main Program.java package trucks; 1 2 3 4 -import exercise.Display: import java.awt.Color; import java.awt.Graphics; 5 6 7 public class MainProgram { 9 @ HT 10 public static void testBoxedTruck (Display display, Truck truck) throws Exception{ // Pause for a bit display.pause( milliseconds: 200); 11 12 13 // TODO : draw truck at specific location 14 15 // TODO : move truck to a different location and draw 16 17 // TODO : resize Truck at specific Location 18 19 // Pause for a bit display.pause( milliseconds: 200); 20 21 22 23 @ public static void testflatBedTruck(Display display, Truck truck) throws Exception 24 25 // Pause for a bit display.pause( milliseconds: 200); 26 27 28 // TODO : draw truck at specific location 29 30 // TODO : move truck to a different location and draw 31 32 // TODO : resize Truck at specific location 33 34 // Pause for a bit display.pause milliseconds: 200); 35 36 37 38 public static void main(String[] args) throws Exception { 39 40 Display display = new Display( width: 600, height: 500); display.setBackground(Color.GRAY); 41 42 43 // Get Graphics Pen Object for Drawing in Window Graphics pen = display.getGraphics(); 44 public static void testFlatbedTruck(Display display, Truck truck) throws Exception // Pause for a bit display.pause( milliseconds: 200); // TODO : draw truck at specific location // TODO : move truck to a different location and draw // TODO : resize Truck at specific location // Pause for a bit display.pause( milliseconds: 200); } public static void main(String[] args) throws Exception { Display display = new Display( width: 600, height: 500); display.setBackground(Color.GRAY); // Get Graphics Pen Object for Drawing in Window Graphics pen = display.getGraphics(); // TODO : Create a Boxed Truck for display // clear graphics (if you want to // display.clear(); // TODO : with same reference, to create a Flatbed Truck for display Build Terminal Profiler Program.java Truck.java package trucks; import java.awt.Color; import java.awt.Graphics; public class Truck { protected Color color; protected Graphics pen; protected int size; protected int startX; protected int starty; //TODO: Update class, you are expected to figure this out! protected void drawWheel(int outerx, int outery, int innerx, int innery, int length) { pen.setColor(Color.BLACK); pen.filloval(outerx, outery, Length, Length); pen.setColor(Color.LIGHT_GRAY); pen.filloval(innerx, innery, width: Length / 2, height: Length / 2); protected void drawWindow(int x, int y, int width, int height) { pen.setColor(Color.LIGHT_GRAY); pen.fillRect(x, y, width, height); "Boxed" Truck Object Information about the "Boxed" Truck Class. Note: This class is not complete. You have to figure out the rest yourself. Data Fields Fields Description Value DEFAULT_COLOR a CONSTANT that represents default color of graphics object new Color(250, 185, 0) Constructors Constructor Description Header takes a graphics object and initializes the data field with Boxed(pen) public Boxed(Graphics pen) passed parameter. Boxed(pen, color) takes graphics and color objects. Initializes the data fields with passed parameters. public Boxed(Graphics pen, Color color) Operations Method Description Header draw draws a "Boxed" Truck using graphics object public void drawl drawBody helper method that "helps" build the body of the "Boxed" truck in the method draw private void drawBody(int width, int height) drawToScale draws the resized "Boxed" truck with graphics object public void drawToScale(int length) Boxed.java Program.java Truck.java package trucks; 42 import public class Boxed { //TODO: Update Class, you are expected to figure this out! public void draw() { int outerXLeft = startX + 10; int outerXRight = startX + 70; int outery = starty + 40; int innerXLeft = startX + 15; int innerXRight = startX + 75; int innery = starty + 45; int window = startX + 70; int window = starty + 10; drawBody( width: 100,height: 50); drawWheel(outerXLeft, outery, innerXLeft, innery, 20); drawWheet(outerXRight, outery, innerXRight, innery, 20); drawWindow(windowx, windowy, 30, 20); // left wheel // right wheel } private void drawBody(int width, int height) { pen.setColor(color); pen.fillRect(startx, starty, width, height); } public void drawToScale(int length) { setSize(Length); int outerXLeft = startX + size / 10; int outerXRight = startx + 7 * size / 10; int outery = starty + 2 * size / 5; int innerXLeft = startX + 3 * size / 20; int innerXRight = startX + 3 * size / 4; int innery = starty + 9 * size / 20; int windowX = startX + 7 * size / 10; int window = starty + size / 10; int windowWidth = 3 * size / 10; // x for left wheel // x for right wheel // y for wheels // x for left hubcap // x for right hubcap // y for hubcaps drawBody(size, height: size / 2); "FlatBed" Truck Object Information about the "Boxed" Truck Class. Note: This class is not complete. You have to figure out the rest yourself. Data Fields Fields Value Description a CONSTANT that represents default color of graphics object DEFAULT_COLOR new Color(125, 180, 250) Constructors Constructor Header Flated(pen) public FlatBed(Graphics pen) Description takes a graphics object and initializes the data field with passed parameter. takes graphics and color objects. Initializes the data fields with passed parameters. FlatBed(pen, color) public FlatBed(Graphics pen, Color color) Operations Method Description Header draws "Flatbed" Truck using draw public void drawn graphics object. helper method that "helps" build drawFlatBedBody the body of the "FlatBed" truck in private void drawFlatBedBody(int x, int y) the method draw helper method that "helps" build drawResizedBody the resized body of the "FlatBed" private void drawResizedBody(int x, int y) truck in the method drawToScale draws the resized "Flat Bed" truck drawToScale public void drawToScale(int length) with graphics object OE public class Flatbed { //TODO: Update Class, you are expected to figure this out! public void draw({ int outerXLeft = startX + 10; int outerXRight = startX + 70; int outery = starty + 5; int innerXLeft = startX + 15; int innerXRight = startX + 75; int innery = starty + 10; int windows = startX + 60; int window = starty - 15; drawFlatbedBody(start, starty); drawWheel (outerXLeft, outery, innerXLeft, innery, 20); drawWheelCouterXRight, outery, innerXRight, innery, 20); drawWindow(windowx, windowy, 30, 15); // left wheel // right wheel } private void drawFlatbedBody(int x, int y){ pen.setColor(color); pen.fillRect(x, y, 100, 15); pen.fillRect(x + 50, y - 20, 50, 30); } private void drawResizedBody(int x, int y){ pen.setColor(color); pen.fillRect(x, y, size, size / 6); pen.fillRect(x + size / 2. y - size 1 4, size / 2. 7 * size / 20); } public void drawToScale(int length) { setSize(Length); int outerXLeft int outerXRight int outery int innerXLeft int innerXRight int innery int windows = startX + size / 10; = startX + 7 * size / 10; = starty + size / 20; = startX + 3 * size / 20; = startX + 3 * size / 4; = starty + size / 10; = startX + 6 * size / 10; // x for left wheel // x for right wheel // y for wheels // x for left hubcap // x for right hubcap // y for hubcaps