Question
ClassRoll.Java CODE import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.LinkedList; import java.util.List; /** * ClassRoll.java. * A class to illustrate storing and sorting * a
ClassRoll.Java CODE
import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.LinkedList; import java.util.List;
/** * ClassRoll.java. * A class to illustrate storing and sorting * a list of Students. */ public class ClassRoll {
/** Drives execution. */ public static void main(String[] args) {
List
System.out.println("Original Roll: "); roll.forEach(System.out::println); System.out.println();
// Sort the roll in natural order. Collections.sort(roll); System.out.println("Natural Order: "); roll.forEach(System.out::println); System.out.println();
// Shuffle the roll into a random order. // Use the Collections.shuffle() method. // *** Replace this line with your solution. System.out.println("Random Order: "); roll.forEach(System.out::println); System.out.println();
// Sort the roll in ascending order of section Comparator
// Sort the roll in descending order of section. // Use either Collections.reverseOrder() or // Comparator.reversed() in conjunction with // Collections.sort(). // *** Replace this line with your solution. System.out.println("Descending Order of Section: "); roll.forEach(System.out::println); System.out.println(); }
/** * Implement this Comparator so that Student objects are compared * in ascending order of section. That is, compare(s1, s2) must * return a negative integer if s1's section is less than s2's section, * zero if s1 and s2 have the same section, and a postive integer * if s1's section is greater than s2's section. */ public static class CompareStudentsBySection implements Comparator
}
Student.Java CODE:
public class Student implements Comparable
private String fname; private String lname; private int section;
/** Creates a new student. */ public Student(String last, String first, int sec) { lname = last; fname = first; section = sec; }
/** Returns this student's first name. */ public String getFirstName() { return fname; }
/** Returns this student's last name. */ public String getLastName() { return lname; }
/** Returns this student's section. */ public int getSection() { return section; }
/** * Implement compareTo so that students are ordered in the * following way: in ascending order of last name, then in * ascending order of first name, and then in ascending order * of section. */ @Override public int compareTo(Student s) { return -99; }
/** Returns a string representation of this student. */ @Override public String toString() { return section + ", " + lname + ", " + fname; } }
I have this error
Step by Step Solution
There are 3 Steps involved in it
Step: 1
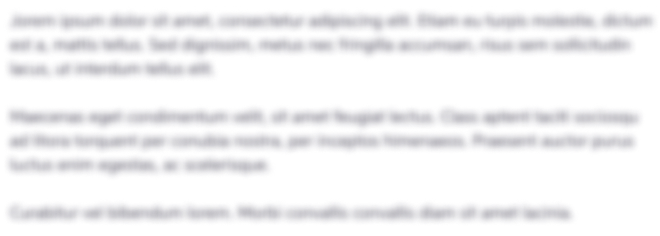
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started