Question
Code for question 2 public class CoffeeMaker { private double waterLevel; private boolean isOn; private CoffeeBeans loadedCoffee; private double tankCapacity; private CoffeeGrinder grinder; public CoffeeMaker(CoffeeBeans
Code for question 2 public class CoffeeMaker { private double waterLevel; private boolean isOn; private CoffeeBeans loadedCoffee; private double tankCapacity; private CoffeeGrinder grinder; public CoffeeMaker(CoffeeBeans loadedCoffee,double tankCapacity) { this.loadedCoffee=loadedCoffee; this.tankCapacity=tankCapacity; waterLevel=0; isOn=false; grinder=new CoffeeGrinder(); } public CoffeeMaker(double tankCapacity) { this.tankCapacity=tankCapacity; this.loadedCoffee=null; waterLevel=0; isOn=false; grinder=new CoffeeGrinder(); } public double getWaterLevel() { return waterLevel; } public void switchPower() { isOn=!isOn; } public boolean isOn() { return isOn; } public boolean hasCoffee() { return loadedCoffee!=null; } public boolean loadCoffee(CoffeeBeans beans) { if(beans==null) return false; this.loadedCoffee=beans; return true; } public void clean() { if(isOn()) switchPower(); loadedCoffee=null; waterLevel=0; } public boolean brewCoffee(int cups) { if(!hasCoffee()) return false; if(loadedCoffee.isGround()==false) grinder.grindCoffee(loadedCoffee); if(getWaterLevel()
test case for to string method is wrong
public static boolean toStringMethod(){ CoffeeBeans someBeans = new CoffeeBeans("Kicking Horse","Horse 427", CoffeeBeans.Roast.DARK); CoffeeMaker machine = new CoffeeMaker(someBeans, 2750); String expected = "isOn: false water left: 0.00 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean"; if(!expected.replaceAll("\\s+","").equalsIgnoreCase(machine.toString().replaceAll("\\s+",""))){ System.out.println("toString method is not returning the correct string."); System.out.println(); System.out.println("Expected: " + expected); System.out.println("Actual: " + machine.toString()); return false; } machine.addWater(1000); machine.switchPower(); expected = "isOn: true water left: 1000.00 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean"; if(!expected.replaceAll("\\s+","").equalsIgnoreCase(machine.toString().replaceAll("\\s+",""))){ System.out.println("toString method is not returning the correct string."); System.out.println(); System.out.println("Expected: " + expected); System.out.println("Actual: " + machine.toString()); return false; } machine.addWater(5000); expected = "isOn: true water left: 2750.00 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean"; if(!expected.replaceAll("\\s+","").equalsIgnoreCase(machine.toString().replaceAll("\\s+",""))){ System.out.println("toString method is not returning the correct string."); System.out.println(); System.out.println("Expected: " + expected); System.out.println("Actual: " + machine.toString()); return false; } machine.brewCoffee(2); expected = "isOn: true water left: 2276.82 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK ground"; if(!expected.replaceAll("\\s+","").equalsIgnoreCase(machine.toString().replaceAll("\\s+",""))){ System.out.println("toString method is not returning the correct string."); System.out.println(); System.out.println("Expected: " + expected); System.out.println("Actual: " + machine.toString()); return false; } machine.clean(); expected = "isOn: true water left: 0.00 no coffee loaded."; if(!expected.replaceAll("\\s+","").equalsIgnoreCase(machine.toString().replaceAll("\\s+",""))){ System.out.println("toString method is not returning the correct string."); System.out.println(); System.out.println("Expected: " + expected); System.out.println("Actual: " + machine.toString()); return false; } return true; }
output on IJ idea( i do not know why the water left is false)
Expected: isOn: true water left: 2276.82 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK ground Actual: isOn: true Water left: 2750.00 loaded coffee: Brand: Kicking Horse Blend: Horse 427 Roast type: DARK ground bean false
public class CoffeeBeans { private boolean isGround; private Roast roast; private String blendName; private String brandName; public CoffeeBeans(String brandName,String blendName,Roast roast,boolean isGround) { this.blendName=blendName; this.brandName=brandName; this.roast=roast; this.isGround=isGround; } public CoffeeBeans(String brandName,String blendName,Roast roast) { this.blendName=blendName; this.brandName=brandName; this.roast=roast; this.isGround=false; } public CoffeeBeans(String brandName,String blendName) { this.blendName=blendName; this.brandName=brandName; this.roast=Roast.MEDIUM; this.isGround=false; } public boolean isGround() { return isGround; } public Roast getRoast() { return roast; } public String getBlendName() { return blendName; } public String getBrandName() { return brandName; } public boolean grind() { if(isGround) return false; isGround=true; return true; } public String toString() { String str="Brand: "+this.brandName+" "; str+="Blend: "+this.blendName+" "; str+="Roast type: "+this.roast+" "; if(isGround) str+="ground bean"; else str+="whole bean"; return str; }
}
Problem 2 - The Coffee Machine and Coffee Grinder To make coffee, we will also need a CoffeeMaker and a CoffeeGrinder. In this problem, you will create both classe according to the diagrams depicted below. Please note that you don't need to write P2 after P1; you can write both in parallel or even P2 before P1. However, you need to have all P1's tests passing before you can thoroughly test the classes on this problem. Coffee Maker - WaterLevel: double - isOn: boolean - loadedCoffee: Coffee Beans - tank Capacity: double - grinder Coffee Grinder + Coffee Maker(CoffeeBeans, double) + Coffee Maker(double) + getWaterLevel(): double + switchPower(); void + isOn(): boolean + hasCoffee(): boolean + loadCoffee(Coffee Beans): boolean + clean(): void + addWatter(double): void + brewCoffee(int): boolean + toString(): String - cups TOMI(int): double Coffee Grinder + grind Coffee Coffee Beans): bolean See how the coffeeMaker class depends on both CoffeeGrinder and CoffeeBeans classes to function fully? Here is a description of the constructors and methods of CoffeeGrinder Default constructor only grindcoffe (CoffeeBeans): grinds the coffee bean received as a parameter. If the beans are already ground return false. Here is a description of the constructors and methods of CoffeeMaker. The machine is always initialized with no water in the tank and off. CoffeeMaker (CoffeeBeans, double): Create a new coffee maker with the beans already loaded into it and define the size of the water tank in millilitres. CoffeeMaker (double): Create a new coffee maker object with a tank size defined by the double parameter in millilitres. getWaterLevel(): returns the amount of water in the tank switchPower(): toggles the power of the coffee maker on and off hasCoffee(): returns true if the coffee maker has coffee loaded onto it; false otherwise laodCoffee (CoffeBeans): loads coffee into the coffee maker. Returns false if the parameter is null. brewCoffee (int): brews int cups of coffee. If the coffee beans in the machine are not ground, it will first grind the coffee using the internal coffee grinder. The machine cannot brew coffee if there isn't enough water for the requested amount of coffee. The machine must be on to brew. Returns true if the maker successfully brewed the coffee. clean() : removes coffee from the machine and empties the water container. cupsToML (int): helper method (hence private) to convert cups to mililitres. One cup is 236.59 ml toString(): returns a String representing the CoffeeMaker object according to the example below With coffee Without coffee isOn: false isOn: false water left: 0.00 water left: 0.00 loaded coffee: no coffee loaded. Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean. There is no input or output for this question. All tests are unit test cases. Problem 2 - The Coffee Machine and Coffee Grinder To make coffee, we will also need a CoffeeMaker and a CoffeeGrinder. In this problem, you will create both classe according to the diagrams depicted below. Please note that you don't need to write P2 after P1; you can write both in parallel or even P2 before P1. However, you need to have all P1's tests passing before you can thoroughly test the classes on this problem. Coffee Maker - WaterLevel: double - isOn: boolean - loadedCoffee: Coffee Beans - tank Capacity: double - grinder Coffee Grinder + Coffee Maker(CoffeeBeans, double) + Coffee Maker(double) + getWaterLevel(): double + switchPower(); void + isOn(): boolean + hasCoffee(): boolean + loadCoffee(Coffee Beans): boolean + clean(): void + addWatter(double): void + brewCoffee(int): boolean + toString(): String - cups TOMI(int): double Coffee Grinder + grind Coffee Coffee Beans): bolean See how the coffeeMaker class depends on both CoffeeGrinder and CoffeeBeans classes to function fully? Here is a description of the constructors and methods of CoffeeGrinder Default constructor only grindcoffe (CoffeeBeans): grinds the coffee bean received as a parameter. If the beans are already ground return false. Here is a description of the constructors and methods of CoffeeMaker. The machine is always initialized with no water in the tank and off. CoffeeMaker (CoffeeBeans, double): Create a new coffee maker with the beans already loaded into it and define the size of the water tank in millilitres. CoffeeMaker (double): Create a new coffee maker object with a tank size defined by the double parameter in millilitres. getWaterLevel(): returns the amount of water in the tank switchPower(): toggles the power of the coffee maker on and off hasCoffee(): returns true if the coffee maker has coffee loaded onto it; false otherwise laodCoffee (CoffeBeans): loads coffee into the coffee maker. Returns false if the parameter is null. brewCoffee (int): brews int cups of coffee. If the coffee beans in the machine are not ground, it will first grind the coffee using the internal coffee grinder. The machine cannot brew coffee if there isn't enough water for the requested amount of coffee. The machine must be on to brew. Returns true if the maker successfully brewed the coffee. clean() : removes coffee from the machine and empties the water container. cupsToML (int): helper method (hence private) to convert cups to mililitres. One cup is 236.59 ml toString(): returns a String representing the CoffeeMaker object according to the example below With coffee Without coffee isOn: false isOn: false water left: 0.00 water left: 0.00 loaded coffee: no coffee loaded. Brand: Kicking Horse Blend: Horse 427 Roast type: DARK whole bean. There is no input or output for this question. All tests are unit test casesStep by Step Solution
There are 3 Steps involved in it
Step: 1
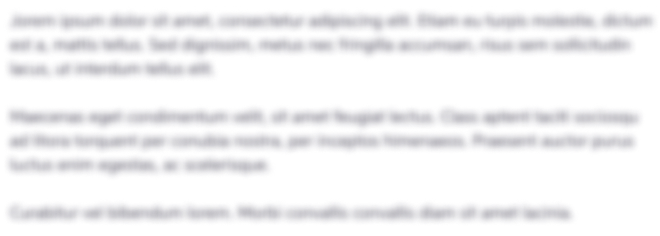
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started