Question
***code in PYTHON*** Assignment: Write a program called Equations.py that represents linear and quadratic equations as classes of objects. You must define methods that allow
***code in PYTHON***
Assignment:
Write a program called Equations.py that represents linear and quadratic equations as classes of objects. You must define methods that allow one to perform basic algebraic operations on them, such as addition, subtraction, multiplication, and composition, as well as methods for evaluating an equation for a given value of x, and printing an equation in an appropriate format.
Getting Started:
Write a class called LinearEquation that includes the following methods:
- __init__(self,m,b), which takes as arguments m, the slope of the line, and b, the y-intercept of the line, and creates a new LinearEquation object.
- __str__(self), which returns a string of the form mx + b suitable for printing.
- evaluate(self,x), which takes as an argument a number x, and returns the value of the equation after substituting in the value.
- __add__(self,otherLE), which returns the sum of two linear equations. That is, if y = ax + b and z = cx + d, then y + z = (a+c)x + (b+d). Note that the__add__ method will return a new LinearEquation object.
- __sub__(self,otherLE), which returns the difference of two linear equations. That is, if y = ax + b and z = cx + d, then y - z = (a-c)x + (b-d). Note that the__sub__ method will return a new LinearEquation object.
- __mul__(self,otherLE), which returns the product of two linear equations. That is, if y = ax + b and z = cx + d, then y * z = (ac)x2 + (ad+bc)x + (bd). Note that the __mul__ method will return a new QuadraticEquation object (see below).
- compose(self,otherLE), which calculates the composition of two linear equations. That is, if y = x + 1 and z = 2x + 5, then y(z) = 2x + 6. Note that the compose operation is not commutative, and that the compose method will return a new LinearEquation object.
Similarly, write a class called QuadraticEquation that includes the following methods:
- __init__(self,a,b,c).
- __str__(self).
- evaluate(self,x).
- __add__(self,otherQE).
- __sub__(self,otherQE).
You do not need to implement a __mul__ method or a compose method for the QuadraticEquation class.
Input and Output
There is no input file for this assignment. Instead, I have provided you with a main program to go with your class definitions. After you have written the classes LinearEquation and QuadraticEquation, append the contents of this file to your class definitions to create a complete program. Run your program, debugging it as necessary, but do NOT change the main program to "make it work". Instead, you should only correct your class definitions to conform to the expectation of the given main program.
If your program is working correctly, it should produce the following output:
f(x) = 5x + 3 f(3) = 18 g(x) = - 2x - 6 g(-2) = -2 h(x) = f(x) + g(x) = 3x - 3 h(-4) = -15 j(x) = f(x) - g(x) = 7x + 9 j(-4) = -19 k(x) = f(g(x)) = - 10x - 27 m(x) = g(f(x)) = - 10x - 12 n(x) = f(x) * g(x) = - 10x2 - 36x - 18 g(x) = 5x - 3 g(-2) = -13 h(x) = f(x) + g(x) = 10x h(-4) = -40 j(x) = f(x) - g(x) = 6 j(-4) = 6 p(x) = x2 + x - 6 p(3) = 6 q(x) = 2x2 + x + 4 q(-3) = 19 r(x) = p(x) + q(x) = 3x2 + 2x - 2 r(-2) = 6 s(x) = p(x) - q(x) = - x2 - 10 s(1) = -11
Some special notes on printing:
- You should never print anything like "y = 2x + -3" or "y = 4x2 + -3x + -2". For linear equations, if b is negative, __str__ should return "mx - b", not "mx + -b". The same is true for negative values of b and c in quadratic equations.
- You should never print anything like "y = 0x + 2" or "y = 0x2 - 3x + 0". If any coefficient (m or b in linear equations, or a, b, or c in quadratic equations) is zero, you should print nothing for that term ("y = 2" or "- 3x" for the examples above).
- Likewise, you should never print a coefficient of 1. For example, "1x2" should be printed as simply "x2".
Step by Step Solution
There are 3 Steps involved in it
Step: 1
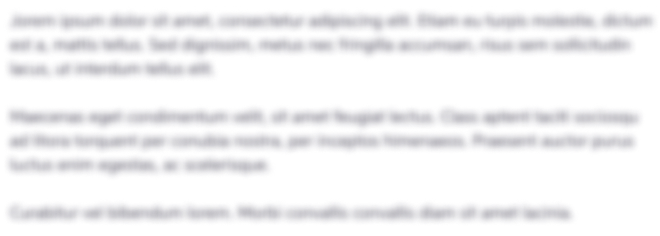
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started