Question
Code of Trackerservice.js file var events = require(events); var Exercise = require(Exercise); //requires weight in lbs Time in minutes distance in miles var tracker =
Code of Trackerservice.js file
var events = require("events");
var Exercise = require("Exercise");
//requires weight in lbs Time in minutes distance in miles
var tracker = function(exercise, weight, distance, time) {
try{
this.exercise=new Exercise(exercise);
this.weight = Number(weight);
this.distance = Number(distance);
this.time = Number(time);
events.EventEmitter.call(this);
} catch (err){
console.log("Error recieved during service creation");
throw err;
}
//updated to accomodate swimming
this.calculate = function() {
return this.exercise.calculate(this.weight, this.distance, this.time);
};
this.calcSpeed = function(){
return this.distance/(this.time/60);//miles per hour
};
this.setExercise = function(exercise){
this.exercise=new Exercise(exercise);
this.emit('exerciseChanged');
};
this.setWeight =function(weight){
this.weight=weight;
this.emit('weightChanged');
};
this.setTime = function(time){
this.time=time;
this.emit('timeChanged', time);
};
this.setDistance = function(distance){
this.distance=distance;
this.emit('distanceChanged', distance);
};
};
tracker.prototype.__proto__=events.EventEmitter.prototype;
module.exports = tracker;
//var test = new tracker("Walking", 200, 4, 45);
//console.log(test.calculate());
Code of Activitytracker.js file:
var tracker = require("tracker");
var events = require("events");
var readline = require("readline");
const reader = readline.createInterface({
input: process.stdin,//use std in as this reader's input/readable stream
output: process.stdout // std out as the reader's output/writable stream
});
var response = function(){
reader.question('What actvity did you perform? (Walking/Running/Swimming)', act => {
reader.question('For how long? (in minutes)', time =>{
reader.question('How far? (in miles)', distance => {
reader.question('What is your weight today? (in pounds)', weight =>
{
console.log(act);
var current = new tracker(act.trim(),weight, distance, time);
console.log(`Calories Burned: ${current.calculate()}`);
//"every time you catch the exerciseChanged event, run this function
current.on('exerciseChanged', () => {
console.log("Exercise Changed!");
console.log(`Calories Burned: ${current.calculate()}`);
});
reader.question('Do you want to (s)tart over, (c)hange your current entry, or exit?', answer=>{
var letter = answer.toLowerCase()[0];
switch (letter){
case "s":
response(); //recall this function and start over again
//Note that I call the function inside the function,
//similar to recursion
break;
case "c":
//change the current activity //Your job: add some code that will allow a user to change any member of the
//tracker. Add some cases too. You'll need to add listeners that are listening
//for the custom tracker events (provided for you).
reader.question('What is your new activity (Swimming/Walking/Running)', rep=>{
current.setExercise(rep); //setExercise emits the event 'exerciseChanged'
process.exit(0); // see the Exercise module for more info
});
break; //Without break, this falls through and exits BEFORE setExercise completes
default:
console.log("Bye!");
process.exit(0);
}
})
})
})
})
})
}
//Chaining callbacks for this effect can sometimes be necessary //because of how Node manages asynchronous function calls, pushing
/ew requests onto the Event queue and running them when it's "their turn". // This means that, depending on a variety of factors, things may run out of // "order" as opposed to fully synchronous languages that you are used to, //where everything in a file is executed in sequential order
//This makes more sense when you recognize that apps are often NOT //synchronized and functions are called only when controls are activated
//Sometimes multiple controls are activated at once, and you don't want //one of them to be held up by the other
response(); //start the response function
//In a typical, syncronous program, you could assume "current" exists here.
//Not so with JS.
//current.calculate() //throws error
Exercise.js code
//This calorie calculations for walking and running are
//implemented as Function Objects. These can be assigned
//to this.exercise at runtime. Defined at class level
//Analagous to static methods.
var walking = function(){
this.calculate = function (weight, distance, time){
return 0.3 * weight * distance;
}
};
//requires weight in lbs, and distance in miles
var running = function(){
this.calculate = function (weight, distance, time){
return 0.63 * weight * distance;
}
};
var swimming = function(){
this.calculate = function (weight, distance, time){
//(6 * Weight in KG * 3.5) / 200; is calories PER MINUTE
kg= weight/2.2;
// console.log("kg " + kg);
// console.log((6 * kg *3.5)/200 * time);
return (((6 * kg *3.5)/200) * time);
}
};
var Exercise = function(type){
if (type.toString().toLowerCase() === "walking"){
this.type = type;
this.calculation = new walking();
} else if (type.toString().toLowerCase() === "running"){
this.type = type;
this.calculation = new running();
} else if (type.toString().toLowerCase() === "swimming"){
this.type=type;
this.calculation = new swimming();
}else {
console.err("Error Exercise Constructor");
throw ({message: "Unknown Exercise. Cannot Create"});
}
}
Exercise.prototype = {
calculate: function(weight, distance, time){
return this.calculation.calculate(weight, distance, time);
}
};
module.exports = Exercise;
Please follow the instruction in the given image and provide the code with steps.
README.md X es U. I WAWANI EU 6 9 13 b3 > lab_activity > README.md 1 These are the instructions for lab activity 3. 2 3 If you inspect the new files for the trackerservice, you'll see 4. that this time I created the tracker with all of its functions 5 directly in the constructor instead of using either prototypes or classes. This is an equally valid of writing the code. 7 8 You'll also notice that a few setters have been added. The setters emit events that indicate a change has occurred. 10 In the main ActivityTracker/activityTracker.js file you'll 11 see that I have added an event handler for the exerciseChanged event 12 Your activity is relatively simple: 14 15 Add a nested switch statement to the change option that allows 16 the user to change ANY of the current trackerservice properties ( allow 17 them to change exercise, weight, distance, or time) 18 and recalculates the speed and/or calories depending on which needs 19 to be updated. Only recalculate what needs to be recalculated. 20 21 The tracker module includes setters for each propery, and each setter 22 already emits events that signal the change. Note that events for time and 23 distance also emit parameters (but you don't need them for this activity). 24. 25 In the main activityTracker.js file, follow the same strategy that was used for 26 changing the exercise (lines 55-58) for changing the other tracker properties. 27 28 To do this, you'll need to add handlers for each event (using either the .on 29 or the .addlistener functions) and you'll need to be able to write and pass 30 some callback functions as the handlers. 31 32 33 34 35 36 README.md X es U. I WAWANI EU 6 9 13 b3 > lab_activity > README.md 1 These are the instructions for lab activity 3. 2 3 If you inspect the new files for the trackerservice, you'll see 4. that this time I created the tracker with all of its functions 5 directly in the constructor instead of using either prototypes or classes. This is an equally valid of writing the code. 7 8 You'll also notice that a few setters have been added. The setters emit events that indicate a change has occurred. 10 In the main ActivityTracker/activityTracker.js file you'll 11 see that I have added an event handler for the exerciseChanged event 12 Your activity is relatively simple: 14 15 Add a nested switch statement to the change option that allows 16 the user to change ANY of the current trackerservice properties ( allow 17 them to change exercise, weight, distance, or time) 18 and recalculates the speed and/or calories depending on which needs 19 to be updated. Only recalculate what needs to be recalculated. 20 21 The tracker module includes setters for each propery, and each setter 22 already emits events that signal the change. Note that events for time and 23 distance also emit parameters (but you don't need them for this activity). 24. 25 In the main activityTracker.js file, follow the same strategy that was used for 26 changing the exercise (lines 55-58) for changing the other tracker properties. 27 28 To do this, you'll need to add handlers for each event (using either the .on 29 or the .addlistener functions) and you'll need to be able to write and pass 30 some callback functions as the handlers. 31 32 33 34 35 36Step by Step Solution
There are 3 Steps involved in it
Step: 1
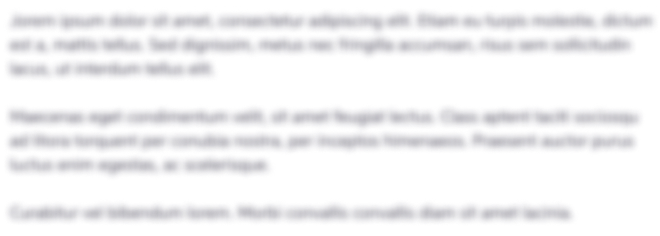
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started