Question
Code using java streams and lambas 1. In the class OfficeHourSchedule, implement the following methods (documentation for each method is provided in the code). a)
Code using java streams and lambas
1. In the class OfficeHourSchedule, implement the following methods (documentation for each method is provided in the code). a) printIsInOn(String day) b) isAvailableFor(String instructor, int minutes)
2. Add a method (call it findInstructorsOnAfter) to OfficeHourSchedule that takes two parameters campus and hour and returns a String containing the names of all instructors who offer an office hour on given campus that starts not before hour. You should exclude duplicate names (if an instructor offers multiple office hours). The string should start with Instructors who are available on campus after hour:00 are:
Hint: Use filter and map to create a stream with all the (possibly duplicate) names. Add these names to an appropriate collection (you need to decide about the right one you want to eliminate the duplicates) and then use reduce on the corresponding stream to produce the output. Decide on the correct identity and formulate a two-parameter lambda that combines the running sum with the next element of the stream.
Here is the code for the classes
import java.util.ArrayList; import java.util.HashSet;
/** * Class OfficeHourSchedule. * It keeps information about available office hours at MUN. * * @author course instructor * @version 2021.02.11 */ public class OfficeHourSchedule { private ArrayList
/** * Constructor for objects of class OfficeHourSchedule */ public OfficeHourSchedule() { officeHours = new ArrayList<>(); addOfficeHoursFromFile("office.csv"); } /** * Prints names of instructors who have office hours on the given day * @param day The day of the week. */ public void printIsInOn(String day) { // put your code here } /** * Checks if instructor is available during the week for at least the given time * @param instructor The name of the instructor * @param minutes The time threashold * @return TRUE if instructor is available for the given threashold; otherwise FALSE */ public boolean isAvailableFor(String instructor, int minutes) { // put your code here return true; } /** * Add the office hours recorded in the given filename to the schedule. * @param filename A CSV file of OfficeHour records. */ private void addOfficeHoursFromFile(String filename) { OfficeHourReader reader = new OfficeHourReader(); officeHours.addAll(reader.getOfficeHours(filename)); }
/** * Prints for all office hours the corresponding info */ public void printAllOfficeHours() { for (OfficeHour officeh : officeHours) { officeh.printInfo(); System.out.println(); } } }
import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.ArrayList; import java.util.Optional; import java.util.function.Function; import java.util.stream.Collectors;
/** * A class to read CSV-style records of office hours . * */ public class OfficeHourReader { // How many fields are expected. private static final int NUMBER_OF_FIELDS = 8; // Index values for the fields in each record. private static final int INSTRUCTOR = 0, DAY = 1, HOUR = 2, MINUTE = 3, DURATION = 4, CAMPUS = 5, OFFICE = 6, COURSE = 7; public OfficeHourReader() { } /** * Read office hours in CSV format from the given file. * Return an ArrayList of OfficeHour objects created from * the information in the file. * * @param filename The file to be read - should be in CSV format. * @return A list of OfficeHour. */ public ArrayList
import java.util.ArrayList; public class OfficeHour { private String instructor; private String dayOfWeek; private int startTimeHours; private int startTimeMinutes; private int durationInMinutes; private String campus; private String office; private String course; // Ignore these two declarations private String minutesString; private String hoursString;
/** * Constructor for objects of class OfficeHour */ public OfficeHour(String instructor, String day, int startTimeH, int startTimeM, int duration, String campus, String office, String course) { this.instructor = instructor; dayOfWeek = day; startTimeHours = startTimeH; startTimeMinutes = startTimeM; durationInMinutes = duration; this.campus = campus; this.office = office; this.course = course; //ignore this part minutesString = startTimeM == 0 ? "00" : "" + startTimeM; hoursString = startTimeH < 10 ? "0" + startTimeH : ""+startTimeH; } public String getInstructor() { return instructor; } public int getStartHour() { return startTimeHours; } public String getCampus() { return campus; } public String getDay() { return dayOfWeek; } public int getDuration() { return durationInMinutes; } /** * Prints information about the office hour */ public void printInfo() { System.out.println(instructor + " | " + dayOfWeek + " | " + hoursString + ":" + minutesString + " | " + durationInMinutes + " minutes | " + campus + " | " + office + " | " + course); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
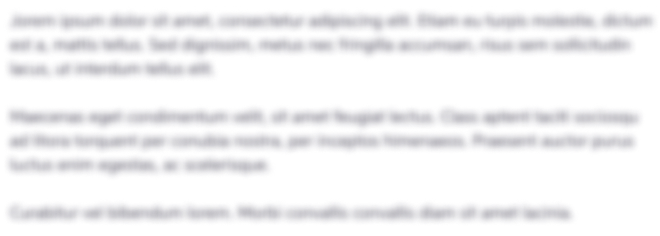
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started