Question
CODE YOUR ANSWER IN C#. I already know what a genetic algorithm looks like, I know how it works, I am asking for someone to
CODE YOUR ANSWER IN C#. I already know what a genetic algorithm looks like, I know how it works, I am asking for someone to do the code
to make a genetic algorithm work for N-Queens. My task is to make a visual N-queens board, in C# and Unity, that is solved by coding a genetic
algorithm (Generate, Evaluate, Sort, Cross-over, and Mutate) and a fitness function. The initial population size should be n = 8. The fitness function will
check the total number of attacks - sum up, for each piece, the number of pieces being attacked (some of these may be counted more than once and
that is fine). We want to find / favor solutions that minimize the number of attacks. Track the average fitness for each generation and graph those result
for 1000 generations. Track the best candidate seen thus far and graph that across the generations as well. Please code in C# and, Do NOT use AI for
this, it is easy to track and tell. Thank you so much for the assistance! ^^
Base Genetic Algorithm in C++ (Want the solution in C# please and thank you):
#include
#include
#include
#include
#include
#include
using namespace std;
int candidates = 6;
int candidateSize = 5;
float keep = 0.5;
float mutateRate = 0.2;
int limit = 10;
struct sample {
string code;
int value;
int eval;
};
int toDec(string decIn);
int f(int valueIn);
void evaluate(vector
void sort(vector
void crossover(vector
void mutate(vector
void generate(vector
for (int num = 0; num < candidates; num++) {
sample sample1;
//Generate a code based on candidateSize
//sample1.code = "010101";
string temp = "";
for (int i = 0; i < candidateSize; i++) {
temp += '0' + (rand() % 2);
}
sample1.code = temp;
//Determine value of string code
//sample1.value = 21;
sample1.value = toDec(sample1.code);
//Pass the value to the fitness function
sample1.eval = -1;
//sample1.eval = f(sample1.value);
samplesIn.push_back(sample1);
}
}
void showCandidates(vector
for (int i = 0; i < samplesIn.size(); i++) {
cout << samplesIn[i].code << "\t" << samplesIn[i].value << "\t" << samplesIn[i].eval << endl;
}
cout << "=======================================================" << endl;
}
int main(int argc, char** argv) {
cout << "Genetic Algorithm" << endl;
//Initializaion
srand(time(NULL));
vector
//Generate
generate(candidates);
showCandidates(candidates);
for (int loop = 0; loop < limit; loop++) {
cout << "Generation " << loop << endl;
//Evaluate
evaluate(candidates);
showCandidates(candidates);
//Sort
sort(candidates);
showCandidates(candidates);
//Cross-over
crossover(candidates);
showCandidates(candidates);
//Mutate
mutate(candidates);
showCandidates(candidates);
}
}
int toDec(string decIn) {
int value = 0;
int power = 0;
for (int i = decIn.size() - 1; i >= 0; i--) {
value += (pow(2, power++) * (decIn[i] - '0'));
}
return value;
}
int f(int valueIn) {
return pow(valueIn,2);
}
void evaluate(vector
for (int i = 0; i < sampleIn.size(); i++) {
sampleIn[i].eval = f(sampleIn[i].value);
}
}
void sort(vector
for (int i = 0; i < sampleIn.size() - 1; i++) {
for (int j = i; j < sampleIn.size(); j++) {
if (sampleIn[i].eval < sampleIn[j].eval) {
//Switch
sample temp = sampleIn[i];
sampleIn[i] = sampleIn[j];
sampleIn[j] = temp;
}
}
}
}
void crossover(vector
//Make temp vector for new samples
vector
//Loop on keep percentage of population size
int keepCandidates = (candidates * keep);
//cout << "Keep " << keepCandidates << " candidates. " << endl;
sample sample1;
sample sample2;
for (int i = 0; i < candidates / 2; i++) {
//Choose two candidates from keep population
sample1 = sampleIn[(rand() % keepCandidates)];
//do {
sample2 = sampleIn[(rand() % keepCandidates)];
//} while (sample1.code == sample2.code);
sample newSample1;
sample newSample2;
//Choose cut location
int cut = rand() % (candidateSize - 2) + 1;
//Make two new candidates
newSample1.code = "";
newSample2.code = "";
for (int j = 0; j < candidateSize; j++) {
if (j < cut) {
newSample1.code += sample1.code[j];
newSample2.code += sample2.code[j];
}
else {
newSample1.code += sample2.code[j];
newSample2.code += sample1.code[j];
}
}
//Save them
newSample1.value = toDec(newSample1.code);
newSample2.value = toDec(newSample2.code);
newSample1.eval = -1;
newSample2.eval = -1;
newCandidates.push_back(newSample1);
newCandidates.push_back(newSample2);
}
//Replace the old candidate list with the new one
for (int i = 0; i < candidates; i++) {
sampleIn[i] = newCandidates[i];
}
}
void mutate(vector
//My mutate function
for (int i = 0; i < samplesIn.size(); i++) {
if (((float)(rand() % 100) / (float)100) < mutateRate) {
int location = rand() % samplesIn[i].code.length();
if (samplesIn[i].code[location] == '0') {
samplesIn[i].code[location] = '1';
}
else if (samplesIn[i].code[location] == '1') {
samplesIn[i].code[location] = '0';
}
samplesIn[i].value = toDec(samplesIn[i].code);
}
}
//reset their strings
for (int i = 0; i < samplesIn.size(); i++) {
samplesIn[i].value = toDec(samplesIn[i].code);
}
}
void generationStatus(vector
float totalFitness = 0;
int largestNum = 0;
for (int i = 0; i < samplesIn.size(); i++) {
//noting the total fitness and then will be average by dividing by the size at the end of the loop
totalFitness += samplesIn[i].eval;
//finding the largest value of the generation
if (i == 0) {
largestNum = samplesIn[i].value;
}
else if (largestNum < samplesIn[i].value) {
largestNum = samplesIn[i].value;
}
}
totalFitness /= samplesIn.size();
cout << "The largest value of this generation is " << largestNum << "\nThe average fitness of this generation is " << totalFitness << endl << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
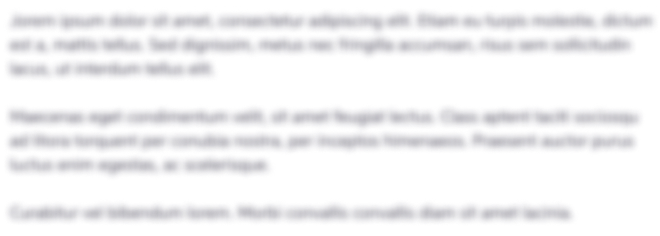
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started