Answered step by step
Verified Expert Solution
Question
1 Approved Answer
COMP 3 3 3 Assignment 2 / / / / WORKING WITH NODE / / You will need node.js ( https: / / nodejs .
COMP Assignment
WORKING WITH NODE
You will need node.js https:nodejsorgen installed and working
to run this. It's also possible, with some tweaking, to get it working
in a Web browser. In that case, you probably will want to replace
consolelog with some output routine.
To work with node from the command line, you can do the following:
Go to the directory containing the file using the cd command
Start node.js with the node command
Within the node.js prompt, type load list.js and hit enter.
This will read your program.
Run the tests by typing runTests and hitting enter.
This will execute the runTests function in this file.
ASSIGNMENT: IMMUTABLE SINGLYLINKED LIST IMPLEMENTATION
In this assignment, you'll be defining code for an immutable
singlylinked list. Lists are constructed with two kinds of objects:
A Cons object represents a nonempty list. It holds a single
element of the list, along with the rest of the list.
A Nil object represents an empty list, containing no elements,
and no other elements.
These objects are not wrapped around anything; if you take a list,
you take Cons or Nil objects.
This list is intended to be used in an immutable way. This means
For example, there is an append operation, but append does
not modify the list it was called on Instead, append will
return a new list, representing the result of the append. For
example, if we append onto append will
return a new list representing and the
original lists and will be unchanged.
Your goal with this assignment is to get all the tests to pass,
without modifying any of the testing code. There are enough
unit tests that they serve as a possibly incomplete specification
of what the code needs to do Some of the provided tests pass
out of the box without any changes you need to do on your end.
HINTS:
The behaviors for appendcontainslengthfilter
and map differ depending on whether or not they are called
on Cons or Nil Some tests force you to use virtual
dispatch to encode this difference.
Singlylinked lists are a recursive data structure, and
the methods can most naturally be implemented with recursion.
My reference solution contains less than lines of code.
If you start needing dramatically more than lines, talk
to me to make sure you're on the right track.
join
Parameters:
A List of elements
A delimeter to separate them by
Returns a single string, which results from calling
toString on each element, separated by the delimeter.
For example:
joinnew Nil
joinnew Cons new Nil
joinnew Cons new Cons new Nil
function joinlist delim
let retval ;
while list instanceof Cons &&
listtail instanceof Nil
retval list.head.toString delim;
list list.tail;
if list instanceof Cons
retval list.head.toString;
retval ;
return retval;
join
function List
List.prototype.join functiondelim
return jointhis delim;
;
List.prototype.toString function
return this.join;
;
function Conshead tail
this.head head;
this.tail tail;
Cons.prototype new List;
Cons.prototype.isEmpty function
return false;
function Nil
Nil.prototype new List;
Nil.prototype.isEmpty function
return true;
;
BEGIN CODE FOR TESTING
Do not modify! When I test your code myself,
I won't use this code below, so I won't be working
with any of your modifications!
function runTesttest
process.stdout.writetestname : ;
try
test;
process.stdout.writepass
;
catch error
process.stdout.writeFAIL
;
console.logerror;
runTest
function assertEqualsexpected received
if expected received
throw tExpected: expected.toString
tReceived: received.toString;
assertEquals
function testniljoin
let nil new Nil;
assertEquals
nil.join;
testniljoin
function testniltoString
let nil new Nil;
assertEquals
nil.toString;
testniltoString
function testnilinstanceoflist
let nil new Nil;
assertEqualstrue
nil instanceof List;
testnilinstanceoflist
function testnilh
Step by Step Solution
There are 3 Steps involved in it
Step: 1
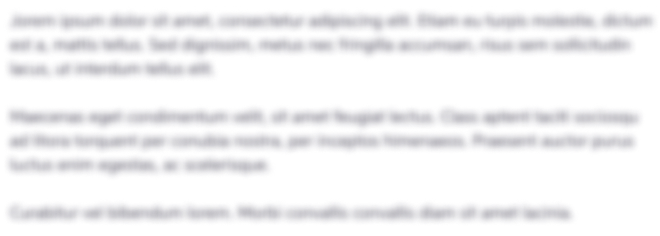
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started