Question
compi sci question 1. (4 pts) Assume the following declarations: char name[21]; char yourName[21]; char studentName[31]; Mark each of the following statement as valid or
compi sci question
1. (4 pts) Assume the following declarations: char name[21]; char yourName[21]; char studentName[31]; Mark each of the following statement as valid or invalid. If a statement is valid, explain what it does. For an invalid statement, explain whats wrong and correct it. a. yourName[0] = '\0'; b. yourName = studentName; c. if (yourName == name) // if contain same words std::cout << "Same word in " << yourName << " and " << name; d. for (int j=0; j < name.length; j++) std::cout << name[j]; PROJECT: Image Processing Write a program to simulate image processing (blur an image, see Unit 3_3_ch8_2dArray slides). An image file is simulated by a 2D array of ints. Shell code is provided in HW5_image_shell.cpp, which includes the following functions: Process an image file (blur the image). Print out blurred image on screen. The screenshot below shows a sample image: Remember, image blurring is done by calculating the weighted average of each pixel (each element of the 2D array). The weights are specified by a predefined 3 by 3 weight mask: 1 2 1 2 2 2 1 2 1 The center weight 2 (highlighted) is applied to a pixel itself and the other weights are applied to its 8 neighbors. New value of a pixel = [ sum (weight for itself/neighbor value of itself/neighbor) ] / sum of weights We will use approach 1 for pixels with less than 8 neightbors: Approach 1: pixels with fewer than 8 neighbors are not processed Blurred result: For example, new value for pixel[1][1] (in yellow rectangle) would be calculated based on itself and 8 neighbors. new value for pixel[1][1] = (1 * 10 + 2 * 100 + 1 * 10 + 2 * 10 + 2 * 300 + 2 * 10 + 1 * 100 + 2 * 10 + 1 * 100) / 14 // 14 is the sum of the weights: 1+2+1 + 2+2+2 + 1+2+1 = 1080 / 14 = 77.14 77 is kept as the result You need to add code to ADD #1-2: ADD #1: declaration of function blur. Be sure to add IN/OUT/INOUT comment to each parameter. ADD #2: implementation of function blur Youre not supposed to modify the given code otherwise (at least 10% penalty).
HW5_Image_shell_cpp
#include #include // std::setw()
//---------------------------------------------- // global declarations //----------------------------------------------
// max dimension of 2D array const int MAX_ROW = 100; const int MAX_COL = 100;
//---------------------------------------------- // function declarations //----------------------------------------------
// blur an image // Pre: pic filled with height x width numbers // Post: pic is blurred using a 3 x 3 predefined weight mask
// ADD CODE #1: function declaration
// END ADD CODE #1
// print an image on screen void printImage(const int pic[][MAX_COL]/*IN*/, int height/*IN*/, int width/*IN*/); // Pre: pic filled with height x width numbers // Post: image printed to screen. The height and width are printed first and then the image file data is printed
//----------------------------------------------
int main() { // one image int image[MAX_ROW][MAX_COL] = { { 10, 100, 10, 100, 10, 100 }, { 10, 300, 10, 300, 10, 300 }, { 100, 10, 100, 10, 100, 10 }, { 300, 10, 300, 10, 300, 10 } };
int imgHeight = 4; // height of image int imgWidth = 6; // width of image
// process the image blur(image, imgHeight, imgWidth); printImage(image, imgHeight, imgWidth);
return 0; } // end main
//---------------------------------------------- // Function Implementation //----------------------------------------------
// blur an image // Pre: pic filled with height x width numbers // Post: pic is blurred using a 3 x 3 predefined weight mask
// ADD CODE #2: implementation of function blur
// END ADD CODE #2
// print an image to output stream obj out void printImage(const int pic[][MAX_COL]/*IN*/, int height/*IN*/, int width/*IN*/) { std::cout << height << ' ' << width << ' '; for (int row = 0; row < height; row++) { for (int col = 0; col < width; col++) { std::cout << std::setw(4) << pic[row][col]; } std::cout << ' '; }
} // end printImage
Step by Step Solution
There are 3 Steps involved in it
Step: 1
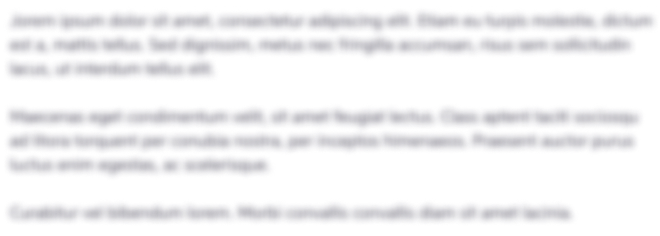
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started