Question
// compile and run this infix class using the Stack class, Tokenizer Class, and Queue Class. Debugged the three issues that I found when I
// compile and run this infix class using the Stack class, Tokenizer Class, and Queue Class. Debugged the three issues that I found when I run the infix class and fixed please import java.io.*; import MyStackQueue.*; class infix { static Queue infixToPostfix(String s) { Tokenizer token = new Tokenizer(s);//step3 Queue queue = new Queue(); Token tkn = token.nextToken();//step5 Stack theStack = new Stack();//step 1 theStack.push(new Operator('#'));//step2 while(tkn != null) {//step4 System.out.println(tkn); if(tkn instanceof Operand) {//step8 queue.enqueue(tkn); } else { Operator Opr = (Operator)tkn; if(Opr.operator == '(') {//step9 theStack.push(Opr); } else if(Opr.operator == ')') { while (((Operator)theStack.top()).operator != '(') { queue.enqueue(theStack.pop());//step6 } theStack.pop(); } else { while (((Operator)theStack.top()).precedence() >= (Opr.precedence())) { queue.enqueue(theStack.pop()); } theStack.push(Opr); } } tkn = token.nextToken(); } while(((Operator)theStack.top()).operator != '#') {//step11 queue.enqueue(theStack.pop()); } theStack.pop(); return queue; } static int evaluePostfix(Queue Post) { Stack theStack = new Stack(); int result= 0; while(!Post.isEmpty()) { Token tkn = (Token)Post.dequeue(); if(tkn instanceof Operand) { theStack.push(tkn); } else if(tkn instanceof Operator) { Operator Opr = (Operator)tkn; int opnd2= (int)((Operand)theStack.pop()).operand; int opnd1= (int)((Operand)theStack.pop()).operand; switch(Opr.operator) {//step 10 to perform an operation case('+'): result = opnd1 + opnd2; break; case('-'): result = opnd1 - opnd2; break; case('*'): result = opnd1*opnd2; break; case('/'): result = opnd1/opnd2; break; } theStack.push(new Operand(result)); } } return result; } public static void main(String[] args) throws IOException { Queue Post; while(true) { System.out.print("Enter infix: "); System.out.flush(); InputStreamReader isr = new InputStreamReader(System.in); BufferedReader br = new BufferedReader(isr); String s = br.readLine(); if ( s.equals("") ) break; Post = infixToPostfix(s); System.out.println("Postfix is " + Post.toString() + ' '); int result = evaluePostfix(Post); System.out.println("Result is " + result + ' '); } } } ------------------------------------------------------------------------------------------------------------------------------------------
class Tokenizer { private char [] Buf; private int cur; Tokenizer(String infixExpression) { Buf = infixExpression.toCharArray(); cur = 0; } boolean moreTokens() { while (cur< Buf.length && Buf[cur]== 32){//. cur++; if (Buf[cur]!=32) return true; //Skip blanks. } return cur
package MyStackQueue; public class Stack { private int topIndx = -1; int capacity = 100; private Object S[]; public Stack() { S = new Object[capacity]; } public boolean isEmpty() { return topIndx<0; } public boolean isFull() { return topIndx==capacity-1; } public void push(Object Element) { S[++topIndx] = Element; } public Object pop() { Object Element; Element = S[topIndx]; S[topIndx--] = null; return Element; } public Object top() { return S[topIndx]; } } -------------------------------------------------------------------------------------------------------
package MyStackQueue; public class Queue { private Node Rear = null, Front = null; private int size = 0; public boolean isEmpty() { return Front==null; } public void enqueue(Object Element) { Node Tmp = new Node(); Tmp.Data=Element; if (Rear==null) Rear = Front = Tmp; else { Rear.Next = Tmp; Rear = Tmp;} size++; } public Object dequeue() { Node Tmp = Front; Front = Front.Next; size--; if (Front==null) Rear = null; return Tmp.Data; } public String toString() { Node cur = Front; String Str = " "; while (cur!= null) { Str += cur.Data. toString() + " "; cur = cur.Next; } return Str; } class Node { Object Data; Node Next; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
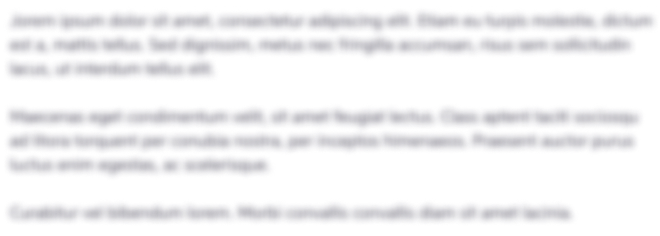
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started