Question
Compiler Design class: (CFG, Bison, Flex, C) Our professor aked us to just make a header file, that has C structures for all 14 rules
Compiler Design class: (CFG, Bison, Flex, C)
Our professor aked us to just make a header file, that has C structures for all 14 rules in the BNF grammar below. ( program, declaration, statementSequence, statement, etc...)
I'm not sure where to start and how to make C structures for those 14 terminals.
----------------------------------------------------------------------------------------------------------------
Numbers, Literals, and Identifiers:
num = [1-9][0-9]*|0
boollit = false|true
ident = [A-Z][A-Z0-9]*
Symbols and Operators:
LP = "("
RP = ")"
ASGN = ":="
SC = ";"
OP2 = "*" | "div" | "mod"
OP3 = "+" | "-"
OP4 = "=" | "!=" | "<" | ">" | "<=" | ">="
Keywords:
IF = "if"
THEN = "then"
ELSE = "else"
BEGIN = "begin"
END = "end"
WHILE = "while"
DO = "do"
PROGRAM = "program"
VAR = "var"
AS = "as"
INT = "int"
BOOL = "bool"
Built-in Procedures:
WRITEINT = "writeInt"
READINT = "readInt"
BNF Grammar
| ?
| ?
|
|
|
| ident ASGN READINT
| ?
|
|
|
| num
| boollit
| LP
Informal Type Rules
The operands of all OP2, OP3, and OP4 operators must be integers
The OP2 and OP3 operators create an integer result.
The OP4 operators create boolean results.
All variables must be declared with a particular type.
Each variables may only be declared once.
The left-hand of assignment must be a variable, and the right-hand side must be an expression of the variable's type.
When used as a value, a variable's type is its declared type.
Only integer variables may be assigned the result of readInt.
writeInt's expression must evaluate to an integer.
The expression guarding if-statements and while-loops must be boolean.
The literals "false" and "true" are boolean constants.
The literal numbers 0 through 2147483647 are integer constants. Numbers outside of that range should be flagged as illegal.
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Here is my flex file if you need it..
%{
#include
#include
#include
#include "proj2b.tab.h"
%}
%option noyywrap
%%
"if" {return IF; }
"then" {return THEN; }
"else" {return ELSE; }
"begin" {return Begin; }
"end" {return End; }
"while" {return WHILE; }
"do" {return DO; }
"program" {return PROGRAM; }
"var" {return VAR; }
"as" {return AS; }
"int" {return INT; }
"bool" {return BOOL;}
"writeInt" {return WRITEINT; }
"readInt" {return READINT; }
[1-9][0-9]*|0 { yylval.val = atoi(yytext); return num; }
"false"|"true" {return boollit; }
[A-Z][A-Z0-9]* { yylval.sval = yytext; return ident; }
"(" {return LP; }
")" {return RP; }
";" {return SC; }
":=" {return ASGN; }
"*"|"div"|"mod" {return OP2; }
"+"|"-" {return OP3; }
"="|"<"|">"|"!="|"<="|">=" {return OP4; }
[ \t ]+ { }
%%
-------------------------------------------------------------------------------------------------------
my bison file: ** this has a lot of mistakes btw.
%{
#include
#include
int yylex();
int yyerror(const char* p) { printf("yyerror: %s ", p); }
%}
%union {
int val;
char *sval;
char sym;
}
%token PROGRAM
%token Begin
%token End
%token VAR
%token AS
%token SC
%token INT
%token BOOL
%token ASGN
%token READINT
%token WRITEINT
%token IF
%token THEN
%token ELSE
%token WHILE
%token DO
%token OP2
%token OP3
%token OP4
%token LP
%token RP
%token num
%token boollit
%token ident
%start Program
%%
Program:
PROGRAM {printf("Program: ");}
Declarations Begin StatementSequence End
;
Declarations:
VAR ident AS Type SC {printf("Declarations: %s ", $2);}
Declarations
| /* nothing */
;
Type: INT {printf("Int Type ");}
| BOOL {printf("Bool Type ");}
;
StatementSequence:
Statement SC {printf("StatementSequence ");}
StatementSequence
| /* nothing */
;
Statement:
Assignment {printf("Assignment statement "); }
| IfStatement
| WhileStatement
| WriteInt
;
Assignment:
ident ASGN Expression {printf("ASGN Expression: %s ", $1);}
| ident ASGN READINT {printf("ASGN READINT %s ", $1); }
;
IfStatement:
IF {printf("IfStatement "); }
Expression THEN StatementSequence ElseClause End
;
ElseClause:
ELSE {printf("ElseClause "); }
StatementSequence
| /*nothing*/
;
WhileStatement:
WHILE {printf("WhileStatement ");}
Expression DO StatementSequence End
;
WriteInt:
WRITEINT {printf("WRITEINT Expression ");}
Expression
;
Expression:
SimpleExpression {printf("SimpleExpression ");}
| SimpleExpression OP4 SimpleExpression {printf("SimpleExpression OP4 SimpleExpression ");}
;
SimpleExpression:
Term OP3 Term {printf("TERM OP3 TERM ");}
| Term
;
Term:
Factor OP2 Factor {printf("Term "); }
| Factor
;
Factor:
ident {printf("ident factor: %s ", $1); }
| num {printf("num factor %d ", $1); }
| boollit {printf("boollit factor "); }
| LP Expression RP {printf("LP Expression RP");}
;
%%
int main(void) {
yyparse();
return 0;
}
---------------------------------------------------------------------------------------------------------------------------------------------------
I just need a C header file that has 14 structures for those described in the grammar.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
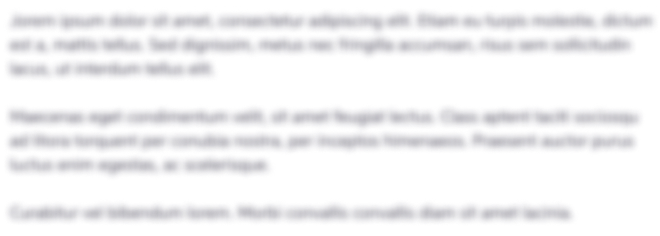
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started