Question
Complex class(attached) enables operations on so called complex numbers. These are numbers of the form realPart + imaginaryPart * i, where i has the value
Complex class(attached) enables operations on so called complex numbers. These are numbers of the form realPart + imaginaryPart * i, where i has the value of square root -1
The following are requirement for this assignment.
a). Modify the class to enable input and output of complex numbers through the overloaded >> and << operators, respectively. The << operator provides print ability. So the print function in the original Complex class can be removed. The >> operator provides the ability to change the private data members of the Complex class object.
b). Overload the multiplication operator to enable multiplication of two complex numbers as in algebra.
c). Overload the division operator to enable division of two complex numbers as in algebra. In here we need handle divide by zero situation.
d). Overload the == and != operators to allow comparisons of complex numbers.
e).Overload the = operator to allow assign a Complex object to the other Complex object.
f) Create CISP400V10A5.cpp to test all the functions designed in the assignment.
The following is an explanation on the complex number calculation.
Adding two complex numbers. The real parts are added together and the imaginary parts are added together. So, if we have (a + bi) + (c + di)), the result should be (a + c) + (b + d) i.
Subtracting two complex numbers. The real part of the right operand is subtracted from the real part of the left operand, and the imaginary part of the right operand is subtracted from the imaginary part of the left operand. So, if we have (a + bi) - (c + di)), the result should be (a - c) + (b - d) i.
Multiplying two complex numbers. The real part of the result is the real part of the right operand multiplies the real part of the left operand minus the imaginary part of the right operand multiply the imaginary part of the left operand. The imaginary part of the result is the real part of the left operand multiply the imaginary part of the left operand plus the imaginary part of the left operand multiply the real part of the right operand. So, if we have (a + bi) * (c + di)), the result should be (ac - bd) + (ad + bc) i.
Dividing two complex numbers. We set the value of square real part of the denominator plus square imaginary part of the denominator is A. The real part of the result is the real part of the numerator multiplies the real part of the denominator plus the imaginary part of the numerator multiply the imaginary part of the denominator and divided by A. The imaginary part of the result is the real part of the left operand multiply the imaginary part of the left operand plus the imaginary part of the left operand multiply the real part of the right operand. So, if we have (a + bi) / (c + di)), the result should be (ac+bd)+i(bc-ad))/(c2+d2).
If you have question regarding the calculation of complex numbers you can refer to Complex numbers.doc file which is attached with this assignment. Or you can search the internet for information.
CISP400V10A5.cpp
In CISP400V10A5.cpp we need to do the following
Instantiate 6 Complex objects ( x, y( 4.3, 8.2 ), z( 3.3, 1.1 ), k, l, and m(0, 0.1)).
Use cin >> k: statement to provide k object data.
Display all the object data by using cout << " x: " << x << " y: " << y << " z: " << z << " k: " << k << " l: " << l << " m: " << m << ' ';
Use x = y + z; statement to demonstrate overloading + and = operators. And use cout << " x = y + z: " << x << " = " << y << " + " << z << ' '; statement to display the result.
Use x = y - z; statement to demonstrate overloading - and = operators. And use cout << " x = y - z: " << x << " = " << y << " - " << z << ' '; statement to display the result.
Use x = y * z; statement to demonstrate overloading * and = operators. And use cout << " x = y * z: " << x << " = " << y << " * " << z << ' '; statement to display the result.
Use x = y / z; statement to demonstrate overloading / and = operators. And use cout << " x = y / z: " << x << " = " << y << " / " << z << ' '; statement to display the result.
Use x = y / l; statement to demonstrate overloading / and = operators. And use cout << " x = y / l: " << x << " = " << y << " / " << l << ' '; statement to display the result. This is a divide by zero situation.
< style="list-style-type:decimal;" >
Use x = y / m; statement to demonstrate overloading / and = operators. And use cout << " x = y / m: " << x << " = " << y << " / " << m << ' '; statement to display the result. This is a divide by a close to zero situation.
Use if ( x != k ) cout << x << " != " << k << ' '; statement to demonstrate overloading ! = operator and to display the result.
Use x = k; statement to demonstrate overloading = operator.
Use if (x == k ) cout << x << " == " << k << ' '; statement to demonstrate overloading == operator and to display the result.
The following are the files that must be modified to achieve the desired result
V10A5.cpp
// V10A5.cpp // Complex class test program. #include
int main() { Complex x; Complex y( 4.3, 8.2 ); Complex z( 3.3, 1.1 );
cout << "x: "; x.print(); cout << " y: "; y.print(); cout << " z: "; z.print();
x = y + z; cout << " x = y + z:" << endl; x.print(); cout << " = "; y.print(); cout << " + "; z.print();
x = y - z; cout << " x = y - z:" << endl; x.print(); cout << " = "; y.print(); cout << " - "; z.print(); cout << endl; } // end main
Complex.cpp
// Complex.cpp // Complex class member-function definitions. #include
// Constructor Complex::Complex( double realPart, double imaginaryPart ) : real( realPart ), imaginary( imaginaryPart ) { // empty body } // end Complex constructor
// addition operator Complex Complex::operator+( const Complex &operand2 ) const { return Complex( real + operand2.real, imaginary + operand2.imaginary ); } // end function operator+
// subtraction operator Complex Complex::operator-( const Complex &operand2 ) const { return Complex( real - operand2.real, imaginary - operand2.imaginary ); } // end function operator-
// display a Complex object in the form: (a, b) void Complex::print() const { cout << '(' << real << ", " << imaginary << ')'; } // end function print
Complex.h
// Complex.h // Complex class definition. #ifndef COMPLEX_H #define COMPLEX_H
class Complex { public: explicit Complex( double = 0.0, double = 0.0 ); // constructor Complex operator+( const Complex & ) const; // addition Complex operator-( const Complex & ) const; // subtraction void print() const; // output private: double real; // real part double imaginary; // imaginary part }; // end class Complex
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
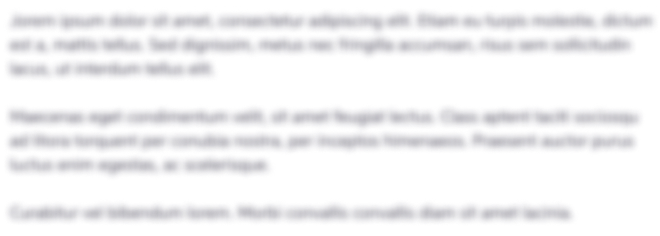
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started