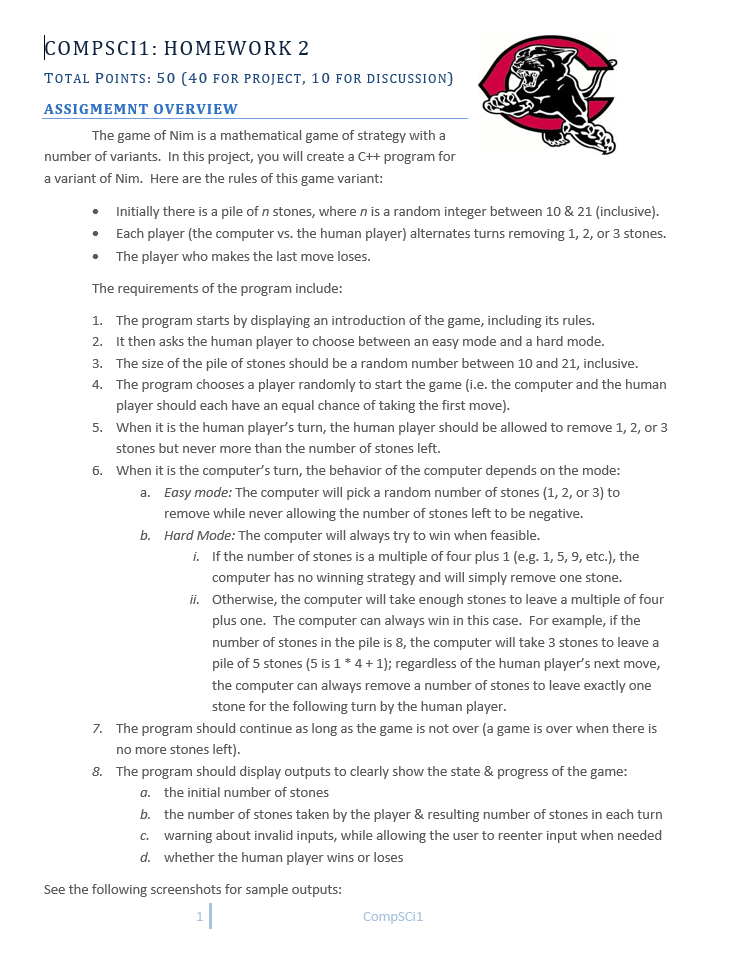
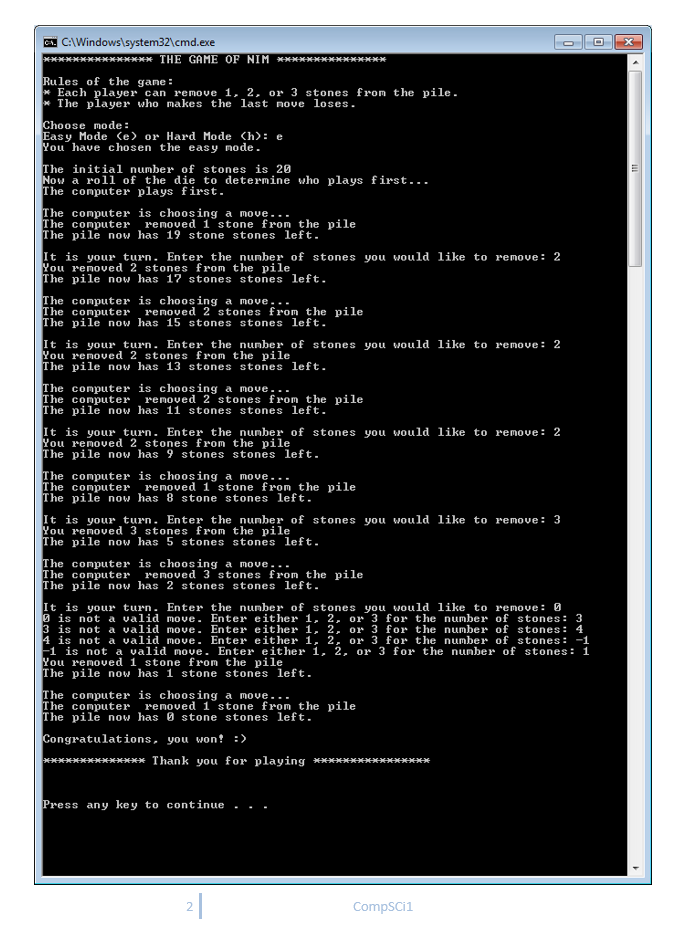
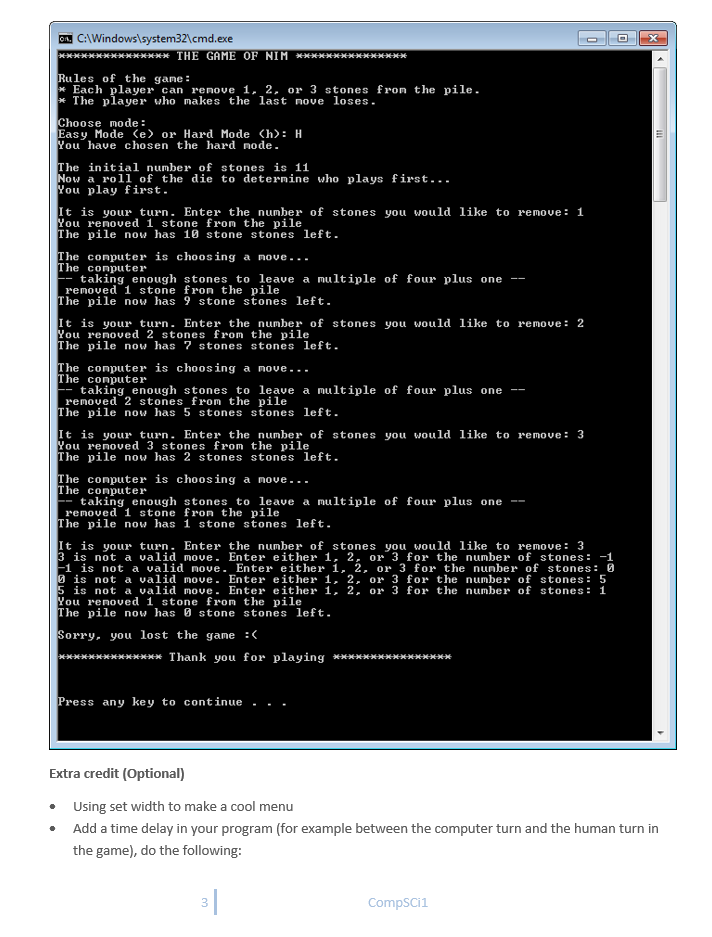
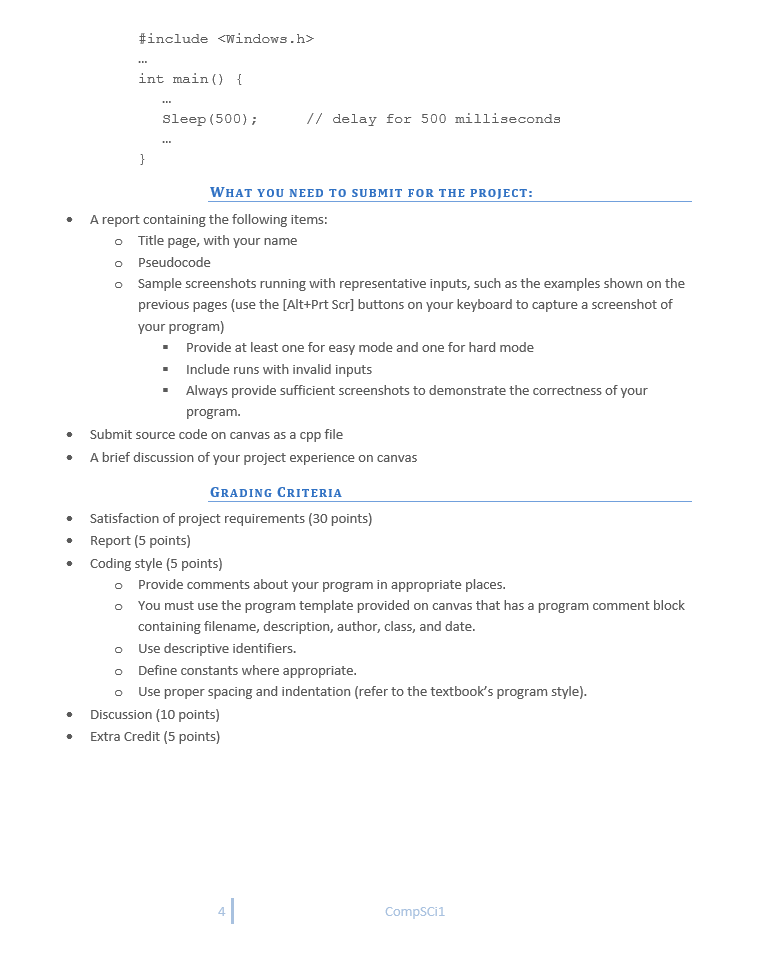
|COMPSCI1: HOMEWORK 2 TOTAL POINTS: 50 (40 FOR PROJECT, 10 FOR DISCUSSION) ASSIGMEMNT OVERVIEW The game of Nim is a mathematical game of strategy with a number of variants. In this project, you will create a C++ program for a variant of Nim. Here are the rules of this game variant: Initially there is a pile of n stones, where n is a random integer between 10 & 21 (inclusive). Each player (the computer vs. the human player) alternates turns removing 1, 2, or 3 stones. The player who makes the last move loses. The requirements of the program include: 1. The program starts by displaying an introduction of the game, including its rules. 2. It then asks the human player to choose between an easy mode and a hard mode. 3. The size of the pile of stones should be a random number between 10 and 21, inclusive. 4. The program chooses a player randomly to start the game (i.e. the computer and the human player should each have an equal chance of taking the first move). 5. When it is the human player's turn, the human player should be allowed to remove 1, 2, or 3 stones but never more than the number of stones left. 6. When it is the computer's turn, the behavior of the computer depends on the mode: a. Easy mode: The computer will pick a random number of stones (1, 2, or 3) to remove while never allowing the number of stones left to be negative. b. Hard Mode: The computer will always try to win when feasible. i. If the number of stones is a multiple of four plus 1 (e.g. 1,5,9, etc.), the computer has no winning strategy and will simply remove one stone. ii. Otherwise, the computer will take enough stones to leave a multiple of four plus one. The computer can always win in this case. For example, if the number of stones in the pile is 8, the computer will take 3 stones to leave a pile of 5 stones (5 is 1* 4+1); regardless of the human player's next move, the computer can always remove a number of stones to leave exactly one stone for the following turn by the human player. 7. The program should continue as long as the game is not over (a game is over when there is no more stones left). 8. The program should display outputs to clearly show the state & progress of the game: a. the initial number of stones b. the number of stones taken by the player & resulting number of stones in each turn c. warning about invalid inputs, while allowing the user to reenter input when needed d. whether the human player wins or loses See the following screenshots for sample outputs: 11 CompScii C. C:\Windows\system32\cmd.exe *************** THE GAME OF NIM *************** Rules of the gane : Each player can remove 1, 2, or 3 stones from the pile. * The player who makes the last nove loses. Choose mode: Easy Mode (e) or Hard Mode (h): e You have chosen the easy node. The initial number of stones is 20 Now a roll of the die to determine who plays first... The computer plays first. The computer is choosing a move... The computer removed 1 stone from the pile The pile now has 19 stone stones left. It is your turn. Enter the number of stones you would like to remove : 2 You removed 2 stones from the pile The pile now has 17 stones stones left. The computer is choosing a move... The computer renoved 2 stones from the pile The pile now has 15 stones stones left. It is your turn. Enter the number of stones you would like to remove : 2 You removed 2 stones from the pile The pile now has 13 stones stones left. The computer is choosing a move... The computer removed 2 stones from the pile The pile now has 11 stones stones left. It is your turn. Enter the number of stones you would like to renove: 2 You removed 2 stones from the pile The pile now has 9 stones stones left. The computer is choosing a move... The computer renoved 1 stone from the pile The pile now has 8 stone stones left. It is your turn. Enter the number of stones you would like to remove: 3 You removed 3 stones from the pile The pile now has 5 stones stones left. The computer is choosing a move... The computer removed 3 stones from the pile The pile now has 2 stones stones left. It is your turn. Enter the number of stones you would like to remove: 0 0 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 3 3 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 4 4 is not a valid move. Enter either 1, 2, or 3 for the number of stones: -1 1 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 1 You removed 1 stone from the pile The pile now has 1 stone stones left. The computer is choosing a move... The computer removed 1 stone from the pile The pile now has a stone stones left. Congratulations, you won! :) ************** Thank you for playing **************** Press any key to continue 21 Compscii Ox. C:\Windows\system32\cmd.exe *************** THE GAME OF NIM *************** Rules of the game: * Each player can renove 1, 2, or 3 stones from the pile. * The player who makes the last nove loses. Choose mode: Easy Mode (e) or Hard Mode (h): H You have chosen the hard node. The initial number of stones is 11 Now a roll of the die to deternine who plays first... You play first. It is your turn. Enter the number of stones you would like to remove: 1 You renoved 1 stone from the pile The pile now has 10 stone stones left. The computer is choosing a move... The computer taking enough stones to leave a multiple of four plus one renoved 1 stone fron the pile The pile now has 9 stone stones left. It is your turn. Enter the number of stones you would like to remove: 2 You renoved 2 stones from the pile The pile now has 7 stones stones left. The computer is choosing a move... The computer taking enough stones to leave a multiple of four plus one removed 2 stones from the pile The pile now has 5 stones stones left. It is your turn. Enter the number of stones you would like to remove: 3 You renoved 3 stones from the pile The pile now has 2 stones stones left. The computer is choosing a nove... The taking enough stones to leave a multiple of four plus one removed 1 stone from the pile The pile now has 1 stone stones left. It is your turn. Enter the number of stones you would like to remove: 3 3 is not a valid move. Enter either 1, 2, or 3 for the number of stones: -1 -1 is not a valid move. Enter either i, 2, or 3 for the nunber of stones: 0 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 5 5 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 1 You renoved 1 stone from the pile The pile now has stone stones left. Sorry, you lost the game :( ************** Thank you for playing **************** Press any key to continue Extra credit (Optional) Using set width to make a cool menu Add a time delay in your program (for example between the computer turn and the human turn in the game), do the following: 31 Compscii #include
int main() { Sleep (500); // delay for 500 milliseconds } WHAT YOU NEED TO SUBMIT FOR THE PROJECT: A report containing the following items: o Title page, with your name o Pseudocode o Sample screenshots running with representative inputs, such as the examples shown on the previous pages (use the [Alt+PrtScr] buttons on your keyboard to capture a screenshot of your program) Provide at least one for easy mode and one for hard mode Include runs with invalid inputs Always provide sufficient screenshots to demonstrate the correctness of your program. Submit source code on canvas as a cpp file A brief discussion of your project experience on canvas GRADING CRITERIA Satisfaction of project requirements (30 points) Report (5 points) Coding style (5 points) Provide comments about your program in appropriate places. o You must use the program template provided on canvas that has a program comment block containing filename, description, author, class, and date. o Use descriptive identifiers. o Define constants where appropriate. o Use proper spacing and indentation (refer to the textbook's program style). Discussion (10 points) Extra Credit (5 points) 41 CompScii |COMPSCI1: HOMEWORK 2 TOTAL POINTS: 50 (40 FOR PROJECT, 10 FOR DISCUSSION) ASSIGMEMNT OVERVIEW The game of Nim is a mathematical game of strategy with a number of variants. In this project, you will create a C++ program for a variant of Nim. Here are the rules of this game variant: Initially there is a pile of n stones, where n is a random integer between 10 & 21 (inclusive). Each player (the computer vs. the human player) alternates turns removing 1, 2, or 3 stones. The player who makes the last move loses. The requirements of the program include: 1. The program starts by displaying an introduction of the game, including its rules. 2. It then asks the human player to choose between an easy mode and a hard mode. 3. The size of the pile of stones should be a random number between 10 and 21, inclusive. 4. The program chooses a player randomly to start the game (i.e. the computer and the human player should each have an equal chance of taking the first move). 5. When it is the human player's turn, the human player should be allowed to remove 1, 2, or 3 stones but never more than the number of stones left. 6. When it is the computer's turn, the behavior of the computer depends on the mode: a. Easy mode: The computer will pick a random number of stones (1, 2, or 3) to remove while never allowing the number of stones left to be negative. b. Hard Mode: The computer will always try to win when feasible. i. If the number of stones is a multiple of four plus 1 (e.g. 1,5,9, etc.), the computer has no winning strategy and will simply remove one stone. ii. Otherwise, the computer will take enough stones to leave a multiple of four plus one. The computer can always win in this case. For example, if the number of stones in the pile is 8, the computer will take 3 stones to leave a pile of 5 stones (5 is 1* 4+1); regardless of the human player's next move, the computer can always remove a number of stones to leave exactly one stone for the following turn by the human player. 7. The program should continue as long as the game is not over (a game is over when there is no more stones left). 8. The program should display outputs to clearly show the state & progress of the game: a. the initial number of stones b. the number of stones taken by the player & resulting number of stones in each turn c. warning about invalid inputs, while allowing the user to reenter input when needed d. whether the human player wins or loses See the following screenshots for sample outputs: 11 CompScii C. C:\Windows\system32\cmd.exe *************** THE GAME OF NIM *************** Rules of the gane : Each player can remove 1, 2, or 3 stones from the pile. * The player who makes the last nove loses. Choose mode: Easy Mode (e) or Hard Mode (h): e You have chosen the easy node. The initial number of stones is 20 Now a roll of the die to determine who plays first... The computer plays first. The computer is choosing a move... The computer removed 1 stone from the pile The pile now has 19 stone stones left. It is your turn. Enter the number of stones you would like to remove : 2 You removed 2 stones from the pile The pile now has 17 stones stones left. The computer is choosing a move... The computer renoved 2 stones from the pile The pile now has 15 stones stones left. It is your turn. Enter the number of stones you would like to remove : 2 You removed 2 stones from the pile The pile now has 13 stones stones left. The computer is choosing a move... The computer removed 2 stones from the pile The pile now has 11 stones stones left. It is your turn. Enter the number of stones you would like to renove: 2 You removed 2 stones from the pile The pile now has 9 stones stones left. The computer is choosing a move... The computer renoved 1 stone from the pile The pile now has 8 stone stones left. It is your turn. Enter the number of stones you would like to remove: 3 You removed 3 stones from the pile The pile now has 5 stones stones left. The computer is choosing a move... The computer removed 3 stones from the pile The pile now has 2 stones stones left. It is your turn. Enter the number of stones you would like to remove: 0 0 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 3 3 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 4 4 is not a valid move. Enter either 1, 2, or 3 for the number of stones: -1 1 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 1 You removed 1 stone from the pile The pile now has 1 stone stones left. The computer is choosing a move... The computer removed 1 stone from the pile The pile now has a stone stones left. Congratulations, you won! :) ************** Thank you for playing **************** Press any key to continue 21 Compscii Ox. C:\Windows\system32\cmd.exe *************** THE GAME OF NIM *************** Rules of the game: * Each player can renove 1, 2, or 3 stones from the pile. * The player who makes the last nove loses. Choose mode: Easy Mode (e) or Hard Mode (h): H You have chosen the hard node. The initial number of stones is 11 Now a roll of the die to deternine who plays first... You play first. It is your turn. Enter the number of stones you would like to remove: 1 You renoved 1 stone from the pile The pile now has 10 stone stones left. The computer is choosing a move... The computer taking enough stones to leave a multiple of four plus one renoved 1 stone fron the pile The pile now has 9 stone stones left. It is your turn. Enter the number of stones you would like to remove: 2 You renoved 2 stones from the pile The pile now has 7 stones stones left. The computer is choosing a move... The computer taking enough stones to leave a multiple of four plus one removed 2 stones from the pile The pile now has 5 stones stones left. It is your turn. Enter the number of stones you would like to remove: 3 You renoved 3 stones from the pile The pile now has 2 stones stones left. The computer is choosing a nove... The taking enough stones to leave a multiple of four plus one removed 1 stone from the pile The pile now has 1 stone stones left. It is your turn. Enter the number of stones you would like to remove: 3 3 is not a valid move. Enter either 1, 2, or 3 for the number of stones: -1 -1 is not a valid move. Enter either i, 2, or 3 for the nunber of stones: 0 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 5 5 is not a valid move. Enter either 1, 2, or 3 for the number of stones: 1 You renoved 1 stone from the pile The pile now has stone stones left. Sorry, you lost the game :( ************** Thank you for playing **************** Press any key to continue Extra credit (Optional) Using set width to make a cool menu Add a time delay in your program (for example between the computer turn and the human turn in the game), do the following: 31 Compscii #include int main() { Sleep (500); // delay for 500 milliseconds } WHAT YOU NEED TO SUBMIT FOR THE PROJECT: A report containing the following items: o Title page, with your name o Pseudocode o Sample screenshots running with representative inputs, such as the examples shown on the previous pages (use the [Alt+PrtScr] buttons on your keyboard to capture a screenshot of your program) Provide at least one for easy mode and one for hard mode Include runs with invalid inputs Always provide sufficient screenshots to demonstrate the correctness of your program. Submit source code on canvas as a cpp file A brief discussion of your project experience on canvas GRADING CRITERIA Satisfaction of project requirements (30 points) Report (5 points) Coding style (5 points) Provide comments about your program in appropriate places. o You must use the program template provided on canvas that has a program comment block containing filename, description, author, class, and date. o Use descriptive identifiers. o Define constants where appropriate. o Use proper spacing and indentation (refer to the textbook's program style). Discussion (10 points) Extra Credit (5 points) 41 CompScii