Question
Computer Science Assignment 1 Implement the Cache class such that it passes all of the tests the CacheGrader class implements. Code: Skeleton (Where any code
Computer Science
Assignment 1
Implement the Cache class such that it passes all of the tests the CacheGrader class implements.
Code:
Skeleton (Where any code that is written goes)
/** * Class implementing a basic cache with a configurable size and associativity. * * The cache is backed-up by a "Memory" object which actually stores stores the values -- on a cache miss, the Memory should be accessed. * */ public class Cache implements ReadOnlyCache { private Memory m_memory;
/** * Constructor * @param memory - An object implementing the Memory functionality. This should be accessed on a cache miss * @param blockCount - The number of blocks in the cache. * @param bytesPerBlock - The number of bytes per block in the cache. * @param associativity - The associativity of the cache. 1 means direct mapped, and a value of "blockCount" means fully-associative. */ public Cache(Memory memory, int blockCount, int bytesPerBlock, int associativity) { m_memory = memory; }
/** * Method to retrieve the value of the specified memory location. * * @param address - The address of the byte to be retrieved. * @return The value at the specified address. */ public byte load(int address) { // TODO: implement the logic to perform the specified // fetch using caching logic. This implementation // does not do any caching, it just immediately // accesses memory, and is not correct. return m_memory.read(address, 1)[0]; } }
DO NOT WRITE CHANGE THESE FOLLOWING CLASSES, they are to be implemented in cache.
/** * Class to represent the Random Access Memory (RAM) of a * computer. Data can be read and written at any valid address. * * Multi-byte data are organized in big-endian order (i.e., most * significant byte is at the lowest address). */ public class Memory { protected final byte[] m_data; public Memory(int nBytes) { m_data = new byte [ nBytes ]; }
public byte read1(int address) { return m_data[address]; }
public byte[] read(int address, int nBytes) { byte[] out = new byte [ nBytes ]; for(int i=0; i public byte[] read2(int address) { return read(address, 2); } public byte[] read4(int address) { return read(address, 4); } public byte[] read8(int address) { return read(address, 8); } public void write(int address, byte[] data) { for(int i=0; i public void write1(int address, byte data) { m_data[address] = data; } } public interface ReadOnlyCache { public byte load(int address); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
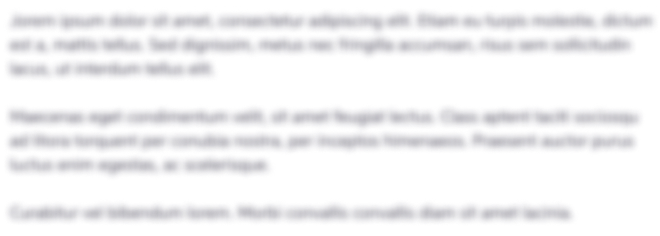
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started