Question
CONCERN : Save Button The user will input a song in the textfield and search for it by using the search button. If the user
CONCERN: Save Button
The user will input a song in the textfield and search for it by using the search button. If the user saw the song and it is existing in the database, the user have to modify the song by using the textfields on the side. And if the user clicked the save button, the database should update also.
For example you search for the song below:
song title artist aired
pink skies LANY 2017
And you want to modify it and make it Drivers License. After you click the save button the database will be like this:
song title artist aired
drivers license Olivia Rodrigo 2021
The code is below and I need to update it.
Kindly show also the output. Thank you.
import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JLabel; import javax.swing.JTextField; import javax.swing.JButton;
import javax.swing.SwingUtilities;
import java.awt.GridLayout; import java.awt.ComponentOrientation;
import java.awt.event.ActionListener; import java.awt.event.ActionEvent;
import java.sql.Connection; import java.sql.DriverManager; import java.sql.Statement; import java.sql.ResultSet;
public class DbUpdate {
static Connection objConn; static ResultSet objResultSet; static Statement objSQLQuery;
static boolean boolConn2Db;
static JLabel lblSearch; static JLabel lblTitle; static JLabel lblAired; static JLabel lblBand; static JLabel lblSong; static JLabel lblYear; static JLabel lblSinger; static JTextField txtSearch; static JTextField txtSong; static JTextField txtYear; static JTextField txtSinger; static JButton btnSearch; static JButton btnSave; static JButton btnExit; static JFrame frmDbUpdate; static JPanel pnlDbUpdate;
DbUpdate() {
String strDriver = "com.mysql.jdbc.Driver"; String strConn = "jdbc:mysql://localhost:3306/dbProto"; String strUser = "linus"; String strPass = "password123";
boolConn2Db = false;
try { Class.forName(strDriver); objConn = DriverManager.getConnection(strConn, strUser, strPass); objSQLQuery = objConn.createStatement(); boolConn2Db = true; } catch (Exception objEx) { System.out.println("Problem retrieving information.."); System.out.println(objEx); } // try
if (boolConn2Db) { setupGUI(); setupListener(); } // if (boolConn2Db) } // DbUpdate()
static void setupGUI() { lblSearch = new JLabel("Song"); lblTitle = new JLabel("Title"); lblAired = new JLabel("Aired"); lblBand = new JLabel("Band"); lblSong = new JLabel(""); lblYear = new JLabel(""); lblSinger = new JLabel(""); txtSearch = new JTextField(30); txtSong = new JTextField(30); txtYear = new JTextField(10); txtSinger = new JTextField(30); btnSearch = new JButton("Search"); btnSave = new JButton("Save"); btnExit = new JButton("Exit");
frmDbUpdate = new JFrame("Table update"); frmDbUpdate.setSize(400, 300); frmDbUpdate.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pnlDbUpdate = new JPanel(); pnlDbUpdate.setLayout(new GridLayout(5, 3)); pnlDbUpdate.setComponentOrientation(ComponentOrientation.LEFT_TO_RIGHT); pnlDbUpdate.add(lblSearch); pnlDbUpdate.add(txtSearch); pnlDbUpdate.add(btnSearch); pnlDbUpdate.add(lblTitle); pnlDbUpdate.add(txtSong); pnlDbUpdate.add(lblSong); pnlDbUpdate.add(lblAired); pnlDbUpdate.add(txtYear); pnlDbUpdate.add(lblYear); pnlDbUpdate.add(lblBand); pnlDbUpdate.add(txtSinger); pnlDbUpdate.add(lblSinger); pnlDbUpdate.add(btnSave); pnlDbUpdate.add(btnExit);
frmDbUpdate.add(pnlDbUpdate); frmDbUpdate.setVisible(true); } // static void setupGUI()
static void setupListener() { btnSearch.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent objAE) { try { boolean boolFound = false; String strSQLQuery = "SELECT strSong, intYear, strSinger " + "FROM tblSong " + "ORDER BY intYear"; String strComp, strData; objResultSet = objSQLQuery.executeQuery(strSQLQuery);
strComp = txtSearch.getText().trim(); while (objResultSet.next()) { strData = objResultSet.getString("strSong").trim(); if (strComp.equals(strData)) { lblSong.setText(objResultSet.getString("strSong")); lblYear.setText(Integer.toString(objResultSet.getInt("intYear"))); lblSinger.setText(objResultSet.getString("strSinger"));
boolFound = true; break; } // if (strComp.equals(strData)) } // while (objResultSet.next())
if (!boolFound) { lblSong.setText("Song"); lblYear.setText("not found"); lblSinger.setText("in the database"); } // if (!boolFound) } catch (Exception objEx) { System.out.println("Problem retrieving information.."); System.out.println(objEx); } // try } // public void actionPerformed(ActionEvent objAE) });
btnExit.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent objAE) { try { if (objResultSet != null) { objResultSet.close(); } // if (objResultSet != null) if (objConn != null) { objConn.close(); } // if (objConn != null) } catch (Exception objEx) { System.out.println("Problem closing the database!"); System.out.println(objEx.toString()); } // try
frmDbUpdate.dispose();
} // public void actionPerformed(ActionEvent objAE) });
} // static void setupListener()
public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable() { public void run() { new DbUpdate(); } // public void run() }); } // public static void main(String[] args)
} // public class DbUpdate
Step by Step Solution
There are 3 Steps involved in it
Step: 1
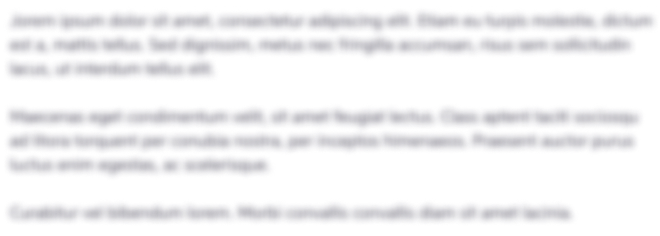
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started