Question
Could you please help me this assighment. This is Object oriented programming based on the concept of Multithreading with synchronization. Thank you so much!. ?
Could you please help me this assighment. This is Object oriented programming based on the concept of Multithreading with synchronization. Thank you so much!. ?
Question 1 - BANK:
For this problem we will simulate a bank. Our bank will track the balances in twenty different accounts. When the program begins, each of the accounts contains $1000. The program will process a list of transactions which transfer money between the accounts. Once all transactions have been processed the program will go through and for each account it will print both the accounts final balance and the number of transactions (deposits and withdrawals) which occurred on that account.
Our bank program will use the main thread to read the list of banking transactions from a file. A separate set of worker threads will process the transactions and update the accounts. Javas BlockingQueue will handle most of the communication between the main thread and the worker threads.
Instead of using a GUI your program will run from the command line. When executing the program users will provide two pieces of information as parametersthe name of an external file listing all the transactions and the number of threads which will be used to process transactions. Here is an example showing a request to process the transactions in the file small.txt using four worker threads.
> java homework6/Bank small.txt 4
acct:0 bal:999 trans:1 acct:1 bal:1001 trans:1 acct:2 bal:999 trans:1 acct:3 bal:1001 trans:1 acct:4 bal:999 trans:1 acct:5 bal:1001 trans:1 acct:6 bal:999 trans:1 acct:7 bal:1001 trans:1 acct:8 bal:999 trans:1 acct:9 bal:1001 trans:1 acct:10 bal:999 trans:1 acct:11 bal:1001 trans:1
acct:12 bal:999 trans:1 acct:13 bal:1001 trans:1
acct:14 bal:999 trans:1 acct:15 bal:1001 trans:1 acct:16 bal:999 trans:1 acct:17 bal:1001 trans:1 acct:18 bal:999 trans:1 acct:19 bal:1001 trans:1
File Format: Each line in the external file represents a single transaction, and contains three numbers: the id of the account from which the money is being transferred, the id of the account to which the money is going, and the amount of money. For example the line:
17 6 104
indicates that $104 is being transferred from Account #17 to Account #6.
The test data provided includes transfers with the same from and to account numbers, so make sure your program will work correctly for these transfers. For example:
5 5 40
Count these as two transactions for the account (one transaction taking money from the account and one putting money into the account).
Termination: We need a way of communicating to the Worker threads when the main thread has finished loading all the transactions into the queue. Since the Worker threads are already getting information from the queue, one easy way to do this is to put a special value into the queue, indicating the main thread is done loading transactions. We cant use null because the BlockingQueue already uses null as a special value for its own communication purposes. Instead you can create a special transactionsomething like this:
private final Transaction nullTrans = new Transaction(-1,0,0); When your main thread is done reading in all the transactions, it places a series of nullTrans references into the queue. When the worker thread pulls a nullTrans out of the queue it knows that all the real transactions are done and it can terminate. Remember that the worker threads are actually removing these references from the queue, so youll need to add one per worker thread. Youll also need a way for the worker threads to let the main thread know when they are done. The main thread cant print out all the account balances until its sure that all transactions have not only been entered in the queue but actually been processed.
Basic Testing: Ive provided you with three files for testing purposessmall.txt, 5k.txt, and 100k.txt. You can find these files under Files -> Homework -> HW6 on Canvas. The correct output for small.txt is shown above. For both 5k.txt and 100k.txt after all the transactions have been processed all accounts should be back to their original $1000 balance level.
Details
I recommend a design with four classesBank, Account, Transaction, and Worker. Both the Account and Transactions classes are quite simple.
Account needs to store an id number, the current balance for the account, and the number of transactions that have occurred on the account. Remember that multiple worker threads may be accessing an account simultaneously and you must ensure that they cannot corrupt its data. You may also want to override the toString method to handle printing of account information.
Transaction is a simple class that stores information on each transaction (see below for more information about each transaction). If youre careful you can treat the Transaction as immutable. This means that you do not have to worry about multiple threads accessing it. Remember an immutable objects values never change, therefore its values are not subject to corruption in a concurrent environment.
The Bank class maintains a list of accounts and the BlockingQueue used to communicate between the main thread and the worker threads. The Bank is also responsible for starting up the worker threads, reading transactions from the file, and printing out all the account values when everything is done. Note: make sure you start up all the worker threads before reading the transactions from the file.
I recommend making the Worker class is an inner class of the Bank class. This way it gets easy access to the list of accounts and the queue used for communication. Workers should check the queue for transactions. If they find a transaction they should process it. If the queue is empty, they will wait for the Bank class to read in another transaction (youll get this behavior for free by using a BlockingQueue). Workers terminate when all the transactions have been processed.
I have put a Bank.java on Canvas. Please feel free to use it as a starting point.
Question 2 - WebLoader
The WebLoader program loads a list url strings from a file. It presents the urls in the left column of a table. When one of the Fetch buttons is clicked, it forks off one or more threads to download the HTML for each url. A Stop button can kill off the downloading threads if desired. A progress bar and some other status fields show the progress of the downloads -- the number of worker threads that have completed their run, the number of threads currently running, and (when done running) the elapsed time.
There are two main classes that make up the WebLoader program
WebFrame
The WebFrame should contain the GUI, keep pointers to the main elements, and manage the overall program flow.
WebWorker
WebWorker is a subclass of Thread that downloads the content for one url. The "Fetch" buttons ultimately fork off a few WebWorkers.
Fetching URLs
When one of the Fetch buttons is clicked, the GUI should change to a "running" state -- fetch buttons disabled, stop button enabled, status strings reset, maximum on the progress bar set to the number of urls.
The swing thread cannot wait around to launch all the workers, so create a special "launcher" thread to create and start all the workers. We will include the launcher thread in the count of "running" threads. Having a separate launcher thread helps keep the GUI snappy we leave the GUI thread to service the GUI. Notice that GUI reacts quickly to mouse button clicks, etc. even as the launcher and all the worker threads are working and blocking.
The launcher should do the following
Run a loop to create and start WebWorker objects, one for each url.
Rather than starting all the workers at once, we will use a limit on the number of workers running at one time. This is a common technique -- starting a thousand threads at once can bog the system down. It's better to limit the number of concurrent workers to some reasonable number. When the Single Thread Fetch button is clicked, limit at one worker. If the Concurrent Fetch button is clicked, limit at the int value in the text field at the time of the click.
Use a semaphore to enforce the limit -- the launcher should not create/start more workers than the limit, and each worker at the very end of its run can signal the launcher that it can go ahead and create/start another worker.
At the very start of its run(), each worker should increment the running- threads count. At the very end of its run, each worker should decrement the running-threads count. The launcher thread should also do this to account for itself, so the running-threads count includes the launcher plus all the currently running workers.
You can detect that the whole run is done when the running thread count gets back down to zero. When that finally happens, compute the elapsed time and switch the GUI back to the "ready" state -- Fetch buttons enabled, Stop button disabled, and progress bar value set to 0.
When the launcher/limit system is working, the running-threads status string should hover right at or below the limit value plus one (for the launcher) at first, and gradually go down to zero. Make a new semaphore for each Fetch run -- that way you do not depend on the semaphore state left behind by the previous run. Interruption may mess up the internal state of the semaphore.
WebWorker Strategy
The WebWorker constructor should take a String url, the int row number in the table that it should update, and a pointer back to the WebFrame to send it messages.
The WebWorker should have a download() method, called from its run(), that tries to download the content of the url. The download() may or may not succeed for any number of reasons, it will probably take between a fraction of a second and a couple seconds to run
Given a url-string, Java has classes that make parsing the URL and retrieving its content pretty easy. The standard code to get an input stream from a URL and download its content is shown below (see the URLConnection API docs, and this code is in the starter file). The process may fail in many different ways at runtime, in which case the flow of control jumps down to the catch clauses and continues from there. If the download succeeds, control gets to the "Success" line.
I have put the sample java code for WebFrame (swing) and WebWorker in Canvas. Feel free to use these as starters.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
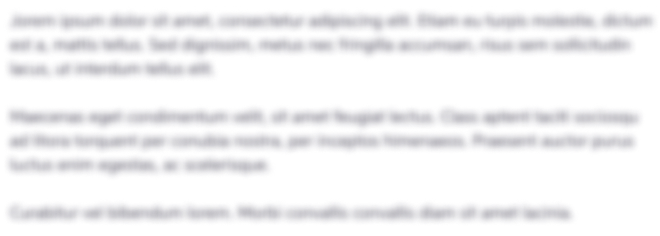
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started