Answered step by step
Verified Expert Solution
Question
1 Approved Answer
country.java /** * A country in the world * @author Cara Tang */ public class Country { private int id; private String name; private long
country.java
/** * A country in the world * @author Cara Tang */ public class Country { private int id; private String name; private long population; private double medianAge; private long coastlineKm; /** * Create a Country object with the given properties */ public Country(int id, String name, long population, double medianAge, long coastlineKm) { this.id = id; this.name = name; this.population = population; this.medianAge = medianAge; this.coastlineKm = coastlineKm; } public int getId() { return id; } public String getName() { return name; } public long getPopulation() { return population; } public double getMedianAge() { return medianAge; } public long getCoastlineKm() { return coastlineKm; } }
countryDB.java
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.ArrayList; import java.util.List; /** * Read data from the Countries database * @author Cara Tang */ public class CountryDB { private static final String DB_NAME = "Countries"; private static final String DB_URL = "jdbc:jtds:sqlserver://cisdbss.pcc.edu/" + DB_NAME; private static final String USERNAME = "233jstudent"; private static final String PASSWORD = "tnedutsj332"; private static final String GET_COUNTRIES_SQL = "SELECT * FROM COUNTRY"; private Listcountries; /** * Create a CountryDB object * Read from the Countries database and populate the countries list */ public CountryDB() { countries = readCountries(); } /** * @return list of countries read from the country database */ public List getCountries() { return countries; } /** * Create and return a connection to the database * @return connection to the countries database */ private Connection getConnection() throws SQLException { Connection connection = DriverManager.getConnection(DB_URL, USERNAME, PASSWORD); return connection; } /** * Read country info from the Country table. * If an error occurs, a stack trace is printed to standard error and an empty list is returned. * @return the list of countries read */ private List readCountries() { List countries = new ArrayList(); try ( Connection connection = getConnection(); PreparedStatement stmt = connection.prepareStatement(GET_COUNTRIES_SQL); ResultSet rs = stmt.executeQuery() ) { while (rs.next()) { countries.add(new Country(rs.getInt("Id"), rs.getString("Name"), rs.getLong("Population"), rs.getDouble("MedianAge"), rs.getLong("CoastlineKm"))); } } catch (SQLException e) { System.err.println("ERROR: " + e.getMessage()); e.printStackTrace(); } return countries; } }
main.java
import java.util.List; /** * Read from the Country database and print data on the countries * @author Cara Tang */ public class Main { public static void main(String[] args) { CountryDB cdb = new CountryDB(); ListImplement Project 1. Look at the starting source code. 2. Run the program and see what it does. Currently it reads the basic country information from the COUNTRY table of the database and prints information on the first country only. 3. Start by updating the Main class so that it prints information on all the countries, not just the first one. 4. Your primary task is to update the code so that the languages of each country are also read from the database and included in the output. Languages are stored in a second table, COUNTRY_LANGUAGE, which has two columns: Countryld and Language. The tables COUNTRY and COUNTRY_LANGUAGE have a 1:M (one-to-many) relationship. One country can have many languages. For example, the country Canada has languages English and French. The Countryld column in COUNTRY_LANGUAGE is a foreign key to the COUNTRY table. Country Database ERD COUNTRY COUNTRY_LANGUAGE int Name nvarchar Population bigint MedianAge decimal CoastlineKm bigint Countryld (FK) int Language nvarchar 5. Update the project to read the languages. You can follow these steps: o Update the Country class so that it can store a list of languages. You need to use a collection because a country can have more than 1 language. You'll need to add a field for the list, a getter, and a method that allows you to add a language to the collection (e.g., addLanguage(String language)) o Update CountryDB to read the languages. Update Main to print the languages for each country. 6. Hint: This project parallels the project used in the JDBC Tutorial. The last part of the tutorial shows how to implement code to read email addresses for each customer. You can do something similar to read languages for each country. 7. Use good coding style & design and follow standard Java conventions. 8. Test your code as you go. 9. Document your changes. o For any changed classes, be sure your name is in the @author list and update the version. o As you add and update methods make sure each method has a comment header that correctly describes its behavior. Implement Project 1. Look at the starting source code. 2. Run the program and see what it does. Currently it reads the basic country information from the COUNTRY table of the database and prints information on the first country only. 3. Start by updating the Main class so that it prints information on all the countries, not just the first one. 4. Your primary task is to update the code so that the languages of each country are also read from the database and included in the output. Languages are stored in a second table, COUNTRY_LANGUAGE, which has two columns: Countryld and Language. The tables COUNTRY and COUNTRY_LANGUAGE have a 1:M (one-to-many) relationship. One country can have many languages. For example, the country Canada has languages English and French. The Countryld column in COUNTRY_LANGUAGE is a foreign key to the COUNTRY table. Country Database ERD COUNTRY COUNTRY_LANGUAGE int Name nvarchar Population bigint MedianAge decimal CoastlineKm bigint Countryld (FK) int Language nvarchar 5. Update the project to read the languages. You can follow these steps: o Update the Country class so that it can store a list of languages. You need to use a collection because a country can have more than 1 language. You'll need to add a field for the list, a getter, and a method that allows you to add a language to the collection (e.g., addLanguage(String language)) o Update CountryDB to read the languages. Update Main to print the languages for each country. 6. Hint: This project parallels the project used in the JDBC Tutorial. The last part of the tutorial shows how to implement code to read email addresses for each customer. You can do something similar to read languages for each country. 7. Use good coding style & design and follow standard Java conventions. 8. Test your code as you go. 9. Document your changes. o For any changed classes, be sure your name is in the @author list and update the version. o As you add and update methods make sure each method has a comment header that correctly describes its behaviorcountries = cdb.getCountries(); Country firstCountry = countries.get(0); System.out.println("First country:"); System.out.println("Name: " + firstCountry.getName() + " Population: " + firstCountry.getPopulation() + " Median Age: " + firstCountry.getMedianAge() + " Coastline: " + firstCountry.getCoastlineKm() + "km"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
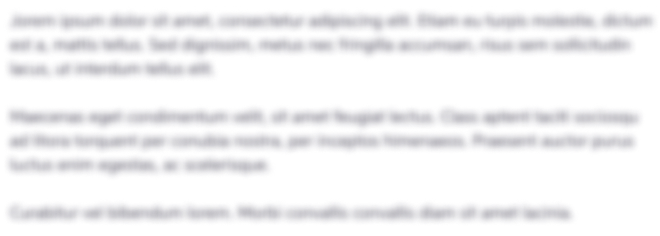
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started