Question
Create a C# class Polynomial with two attributes, an array of real coefficients and an integer deg representing the degree of this polynomial (for simplicity,
Create a C# class Polynomial with two attributes, an array of real coefficients and an integer deg representing the degree of this polynomial (for simplicity, we assume we do not have polynomials of complex coefficients)
(5) Create a method Product that calculates the products of two polynomials.
Product c(x) of two polynomials a(x) and b(x) means that for every coefficient c k of the k-th power x k, c k is the sum of a i and b k-i with i ranging from 0 to k. This one seems very obvious, however there can be some trick spot that causes you out of index exception.
For example, if a(x) = 1+x+x2 and b(x) = 1+x, then with k = 2, we are summing c[2] = a[2]b[0]+a[1]b[1] + a[0]b[2], and b[2] does not exist since b as an array has only two entries.
The way to get around is: define bnew as 1+x+0x2 ({1, 1, 0}), or 1+x+0x2+0x3 {1, 1, 0, 0} etc., which is a copy of b(x) but with a valid index at i = 2, or i = 3, etc., and define similar things for anew, and calculate c[2] = anew[2]bnew[0]+anew[1]bnew[1] + anew[0]bnew[2].
Can use whatever C# code you like. This is what was provided to me though. The only problem i've encountered with the code is that the sum of two polynomials only adds up to degree 2.
polynomial code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace _3_polynomial { class polynomial { private double[] poly = new double[1];
public polynomial(params double[] coeff) //use params when having an array as an input { this.poly = new double[coeff.Length]; //set new length of polynomial to amount of numbers you inputted
for (int i = 0; i < coeff.Length; i++) //assign values you inputted into the array { this.poly[i] = coeff[i]; } }
public void print() { int length = this.poly.Length - 1; //since 0-indexed, subtract by 1
Console.Write("{0} = ", this.poly[0]); //print out what polynomial is equal to first
for (int j = 1; j < length; j++) { Console.Write("{0}x^{1} + ", this.poly[j], j - 1); //print out array with '+' for all contents except the last } Console.WriteLine("{0}x^{1}", this.poly[length], length - 1); //print out last entry seperately as we don't want '+' attached to the end }
public int degree() { int length = this.poly.Length - 1; int degree = 0;
for (int k = length; k > 0; k--) //use for loop to check if coeff is 0 { if (this.poly[k] != 0) //if coeff is not 0, then we are currently sitting on the highest order { degree = k + 1; //add 1 b/c 0-index } }
Console.WriteLine("Degree: {0}", degree);
return degree; }
public void add(polynomial p2) { //LINQ shenannigans learned from Deitel's book chapter something double[] sum = this.poly.Select( (x, index) => x + p2.poly[index] ).ToArray();
polynomial ans = new polynomial(sum); Console.Write("Sum: "); ans.print();
}
public double horner(double a) { int length = this.poly.Length - 1; double ans = 0;
for (int l = 1; l <= length; l++) { ans += this.poly[l] * Math.Pow(a, l - 1); //basically do coeff * x^degree for each coefficient. ex: A then Bx then Cx^2 then Dx^3 and so on }
ans -= this.poly[0]; //bring what polynomial was equal to other side to solve for x Console.WriteLine("Evaluating @ x = {0}: {1}", a, ans); return ans; }
public void quadroots() { double a = this.poly[3]; double b = this.poly[2]; double c = this.poly[1] - this.poly[0]; //gather the constants together double rootp; double rootn;
rootp = (-b + Math.Sqrt(b * b - 4 * a * c)) / (2 * a); rootn = (-b - Math.Sqrt(b * b - 4 * a * c)) / (2 * a);
Console.WriteLine("Root 1: {0} Root 2: {1}", rootp, rootn); } } }
polynomialTest code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace _3_polynomial { class polynomialTest { static void Main(string[] args) { //have polynomials go from decreasing order to increasing to make arrays line up and thus easier addition
polynomial test = new polynomial(1, 2, 3, 0); //1 = 2 + 3x + 0x^2 test.print(); test.degree(); test.horner(3);
Console.WriteLine();
polynomial test2 = new polynomial(0, -2, -3, 1); //0 = -2 -3x +1x^2 test2.print(); test2.degree(); test2.horner(1);
Console.WriteLine();
test.add(test2); test2.quadroots(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
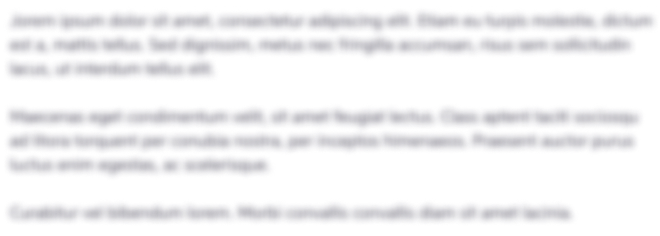
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started