Question
Create a C program: A caesar cipher is a simple cipher that shifts letters in a string. For example, shifting ab over by 1 would
Create a C program:
A caesar cipher is a simple cipher that shifts letters in a string. For example, shifting ab over by 1 would result in bc, and shifting xyz over by 2 would result in zab. The caesar program should take, in the command line an integer k, the amount to shift some text by, and a string f i l e, the name of a file containing text to encode using the cipher.
For example, suppose secret.txt contains defend the east wall of the castle, then running caesar with 3 should result in the following:
$ cat secret.txt
defend the east wall of the castle
$ ./caesar 3 secret.txt
ghihqg wkh hdvw zdoo ri wkh fdvwoh
HERES THE OUTLINE:
#include
/* This is a prototype for a function. * You should implement it below, not here. */ void caesar(long sz,char buf[sz],int shift);
/* Obtains the filesize by "seeking" at the end and restoring the seek pointer * to where it used to be before the call to filesize. */ long fileSize(FILE* f) {
fseek(f, 0, SEEK_END); long size = ftell(f); rewind(f);
return size; }
void caesar(long sz,char buf[sz],int shift);
/* Read the file into an array and use the caesar encoder with the specified shift * - f : the file to read from * - shift : the shift in the cipher [http://practicalcryptography.com/ciphers/caesar-cipher/] * Hint: use fileSize to figure out how much space you need. * Caesar never sent very long messages to his generals ;-) */ void readAndEncode(FILE* f,int shift) { /* Implement! */ }
/* The actual caesar encoder. Input is: * - sz : the buffer size * - buf : actual buffer * - shift : how much to shift by */ void caesar(long sz,char buf[sz],int shift) { /* Implement! */ }
/* Main program. Input on command line (2): * - first the shift * - second the filename of the secret to be encoded * Do error checking (we need 2 inputs) * The shift should be in (0..26) * We should have a filename * Print the encoded message on the standard output */ int main(int argc,char* argv[]) { if(argc != 3) { printf("usage: caesar
Step by Step Solution
There are 3 Steps involved in it
Step: 1
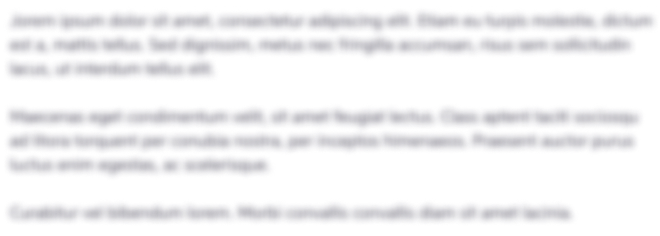
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started