Question
Create a class called AddressBook that uses either in the data section of the class. Since this is a template / generic type you need
Create a class called AddressBook that uses either
in the data section of the class. Since this is a template / generic type you need to make sure that you specify that ArrayList or vector will hold a Person. You should use the Person class you created in Assignment 1. Essentially, we are creating a collection class. In other words, the AddressBook class will be a collection of Person classes.
Constructors
AddressBook(); - default constructor. Should have no functionality. AddressBook(string first, string last); - Adds a Person class to the collection and sets address to an empty string AddressBook(string first, string last, string address); - Adds a Person class to the collection.
Mutators
setPerson(string first, string last, string address); - Adds a Person to the collection
Accessors
C++ All accessors will return a pointer to a Person
- Person *getPerson(); - Returns the next Person in the collection. Each time this function is called it will return the next Person in the list or NULL if the AddressBook is empty. Once you get to the end of the list you should start from element 0 and return the first person.
- Person *findPerson(string last); - returns the found Person or NULL if Person is not in the AddressBook
- Person *findPerson(string first, string last); - returns the found Person or NULL of Person is not in the AddressBook
- void print();- Prints out the entire AddressBook
Note:
You need to use one code path whenever possible. This means you must have delegating constructors. The working constructor can call a function if you wish. In other words, if you have a function that performs a task, you should call it instead of writing duplicate code.
Main
Create functions in main.cpp that will test each of the functions you created. I will leave it up to you as to how to test them but please make sure that I cannot easily force your functions to crash.
There are two tasks to this assignment.
Task 1
Currently you are using composition by creating an instance of a vector / ArrayList inside your AddressBook. This works fine but if we really think about it, an AddressBook is a collection like a stack or a queue or a vector for that matter. Your task is to:
C++ derive AddressBook from vector. In other words, use the vector as a base class and derive AddressBook from it. This means that you will need to remove the Vector from the private section of your class.
You are going to have to make some fundamental changes to your AddressBook so that you are using the vector / ArrayList functionality to store and retrieve people. In other words, you should be accessing the ArrayList / vector using this. C++ people, dont forget you are returning pointers in the accessors of the AddressBook class
Test your AddressBook the same way you did for assignment 2. If you have coded everything properly it should work the same.
Task 2
Create class called Player that is derived from Person. The Player class should hold the following information
- Average
- Slugging Percentage
- On Base Percentage
All should be double data types
Constructors
- Default
- Player(string first, string last, string address, double average, double slugging, double onBase)
You should delegate the default constructor to eliminate multiple code paths.
Mutators
- void setAverage(double avg
- void setSlugging(double slug)
- void setOBP(double OnBase)
Accessors
- double getAverage()
- double getSlugging()
- double getOBP()
print function
In the Person class add a public function called print. This function will print out the persons first, last, address. This function should be a virtual function C++ or an abstract method Java.
In Player override the print function so that you print out the players name, address, average, slugging %, On base %.
To print the information from the Person class you should call the print function from the Player print function.
Test your new class by creating a couple of Players. Make sure you test all functionality and that your print function prints everything from both Person and Player.
NOTE: Tasks 1 and 2 are main priority. Above is for information on what needs to be done. C++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
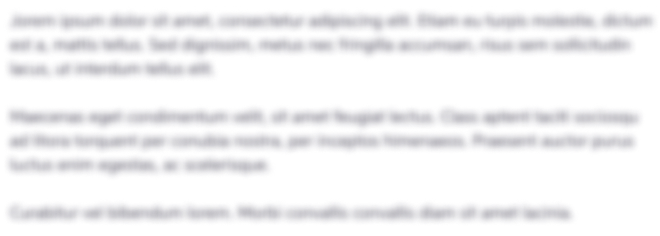
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started